Effective Dozer Mapping for Converting JAXB to Business Domain Objects
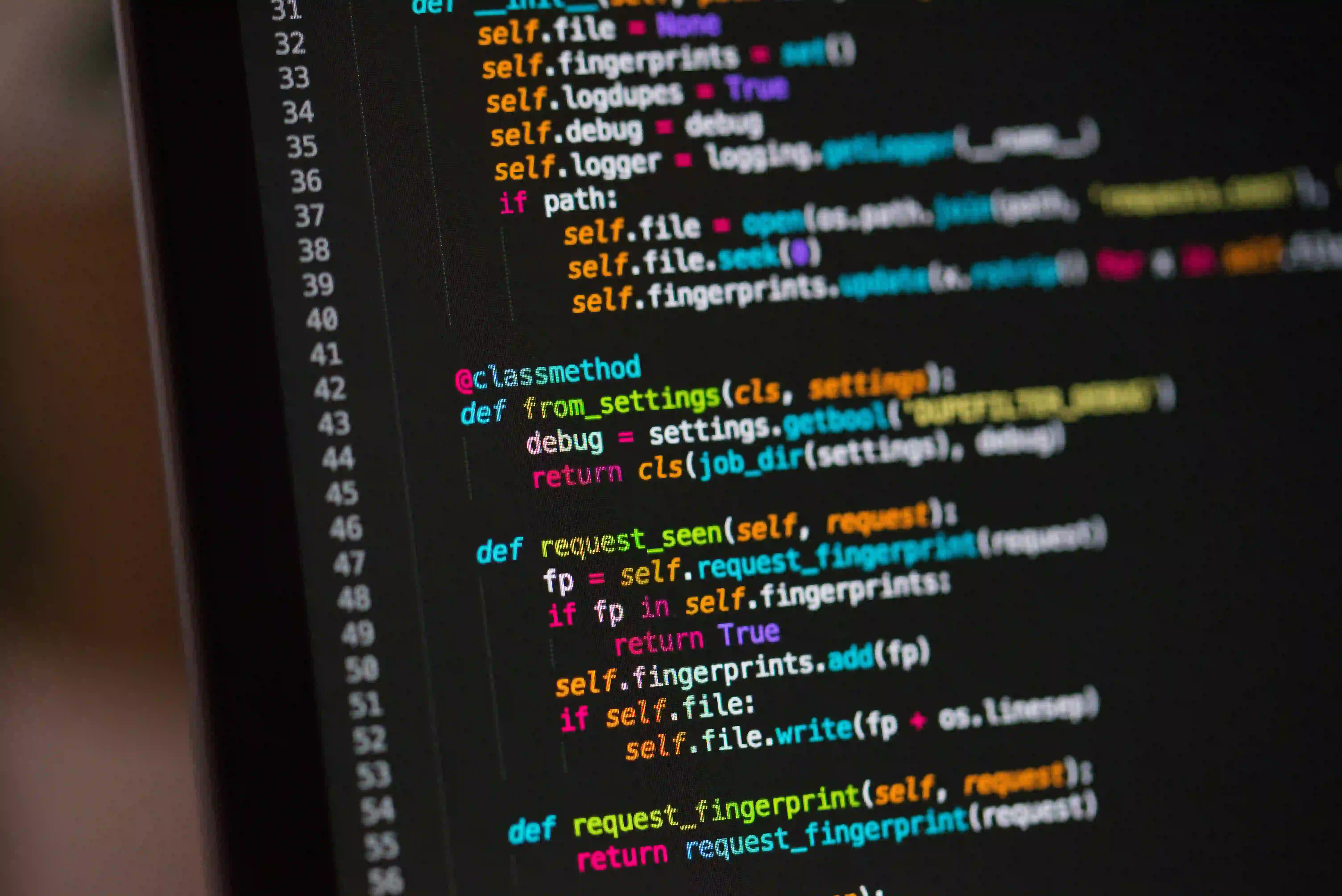
Effective Dozer Mapping for Converting JAXB to Business Domain Objects
When working with Java applications, it's common to encounter the need to convert data from one format to another. In many cases, this involves mapping data from JAXB objects to business domain objects. This process can be streamlined and made more efficient using tools such as Dozer, a powerful Java Bean mapping framework. In this post, we'll explore how to effectively use Dozer to map JAXB objects to business domain objects, and we'll provide some best practices for achieving this in a clean and maintainable way.
Understanding JAXB and Business Domain Objects
Before delving into the Dozer mapping process, it's important to understand the nature of JAXB objects and business domain objects. JAXB (Java Architecture for XML Binding) is a technology that allows Java developers to map Java classes to XML representations. These JAXB objects are often used to unmarshal XML data into Java objects or marshal Java objects into XML data.
On the other hand, business domain objects represent the core entities and value objects within a domain-specific business model. These objects typically encapsulate business logic and are used to model the real-world concepts and relationships within a given application domain.
The process of mapping data from JAXB objects to business domain objects is crucial for integrating external data sources (such as XML payloads) with the internal business logic of an application.
Before We Begin to Dozer
Dozer is a popular Java Bean mapping framework that provides a flexible and powerful approach to object mapping. With Dozer, developers can define mapping configurations using XML or annotations, and the framework takes care of the actual data conversion.
Dozer simplifies the mapping process by allowing developers to specify the mapping rules between different types of objects. It provides support for complex mappings, custom converters, and bidirectional mappings, making it a versatile choice for data transformation tasks.
Setting Up Dozer for Mapping
To start using Dozer for mapping JAXB objects to business domain objects, you'll need to include the Dozer dependency in your project. For Maven users, this involves adding the following dependency to your pom.xml
:
<dependency>
<groupId>net.sf.dozer</groupId>
<artifactId>dozer</artifactId>
<version>5.5.1</version>
</dependency>
Once the Dozer dependency is added, you can begin configuring the mapping rules for your JAXB and business domain objects.
Defining Mapping Rules
Dozer allows mapping configurations to be defined using XML or annotations. In this example, we'll use XML to specify the mapping rules. Here's a basic example of a Dozer mapping file (mapping.xml
) that maps JAXB objects to business domain objects:
<?xml version="1.0" encoding="UTF-8"?>
<mappings xmlns="http://dozer.sourceforge.net" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://dozer.sourceforge.net
https://dozermapper.github.io/schema/beanmapping.xsd">
<mapping>
<class-a>com.example.jaxb.JAXBUser</class-a>
<class-b>com.example.domain.BusinessUser</class-b>
<field>
<a>firstName</a>
<b>name</b>
</field>
<field>
<a>lastName</a>
<b>surname</b>
</field>
<!-- Additional field mappings -->
</mapping>
</mappings>
In this XML mapping file, we define a mapping between a JAXBUser
class and a BusinessUser
class. We specify the mapping rules for individual fields, mapping the firstName
field of the JAXB object to the name
field of the business domain object, and the lastName
field to the surname
field.
Using Dozer Mapper for Mapping
Once the mapping rules are defined, we can utilize the Dozer Mapper to perform the actual mapping process. Here's an example of how to use Dozer for mapping JAXB objects to business domain objects:
import org.dozer.DozerBeanMapper;
public class JAXBToBusinessMapper {
private final DozerBeanMapper dozerMapper;
public JAXBToBusinessMapper() {
dozerMapper = new DozerBeanMapper();
}
public BusinessUser mapJAXBToBusiness(JAXBUser jaxbUser) {
return dozerMapper.map(jaxbUser, BusinessUser.class);
}
}
In this example, we create a JAXBToBusinessMapper
class that contains a DozerBeanMapper
instance. We then define a method mapJAXBToBusiness
that takes a JAXBUser
object and uses the Dozer mapper to convert it to a BusinessUser
object based on the defined mapping rules.
Best Practices for Effective Dozer Mapping
When working with Dozer for mapping JAXB objects to business domain objects, it's important to follow some best practices to ensure clean and maintainable mapping configurations:
-
Use Descriptive Field Names: When defining mapping rules, use descriptive field names to clearly indicate the purpose of each mapping. This improves readability and understanding of the mapping configurations.
-
Separate Complex Mappings: For complex mappings involving nested objects or custom data transformations, consider separating the mapping configurations into distinct mapping files or sections. This helps in organizing and managing the mapping rules effectively.
-
Unit Test Mapping Configurations: Write unit tests to validate the correctness of the mapping configurations. Use sample input data to verify that the mapping behaves as expected, especially for edge cases and complex mappings.
-
Leverage Custom Converters: Dozer allows the creation of custom converters to handle specific data transformation requirements. Leveraging custom converters can streamline the mapping process and accommodate unique mapping scenarios.
-
Documentation and Comments: Document the mapping configurations and include comments to explain any non-trivial mappings or edge cases. This documentation serves as a valuable resource for maintaining and extending the mapping logic in the future.
By adhering to these best practices, developers can ensure that their Dozer mapping configurations are robust, maintainable, and well-documented.
Closing the Chapter
In this post, we've explored the effective use of Dozer for mapping JAXB objects to business domain objects. By leveraging Dozer's powerful mapping capabilities, developers can streamline the data conversion process and integrate external data sources with their application's internal business logic. With careful consideration of mapping rules and best practices, Dozer empowers Java developers to efficiently handle data transformations in a clean and maintainable manner.
With the understanding of Dozer mapping, developers can enhance their data integration workflows and optimize the mapping of complex object structures.
To delve deeper into Dozer and its capabilities, check out the official Dozer documentation for comprehensive insights and advanced features.
Start enhancing your data conversion workflows with Dozer today!