Improving code readability with effective method parameter names
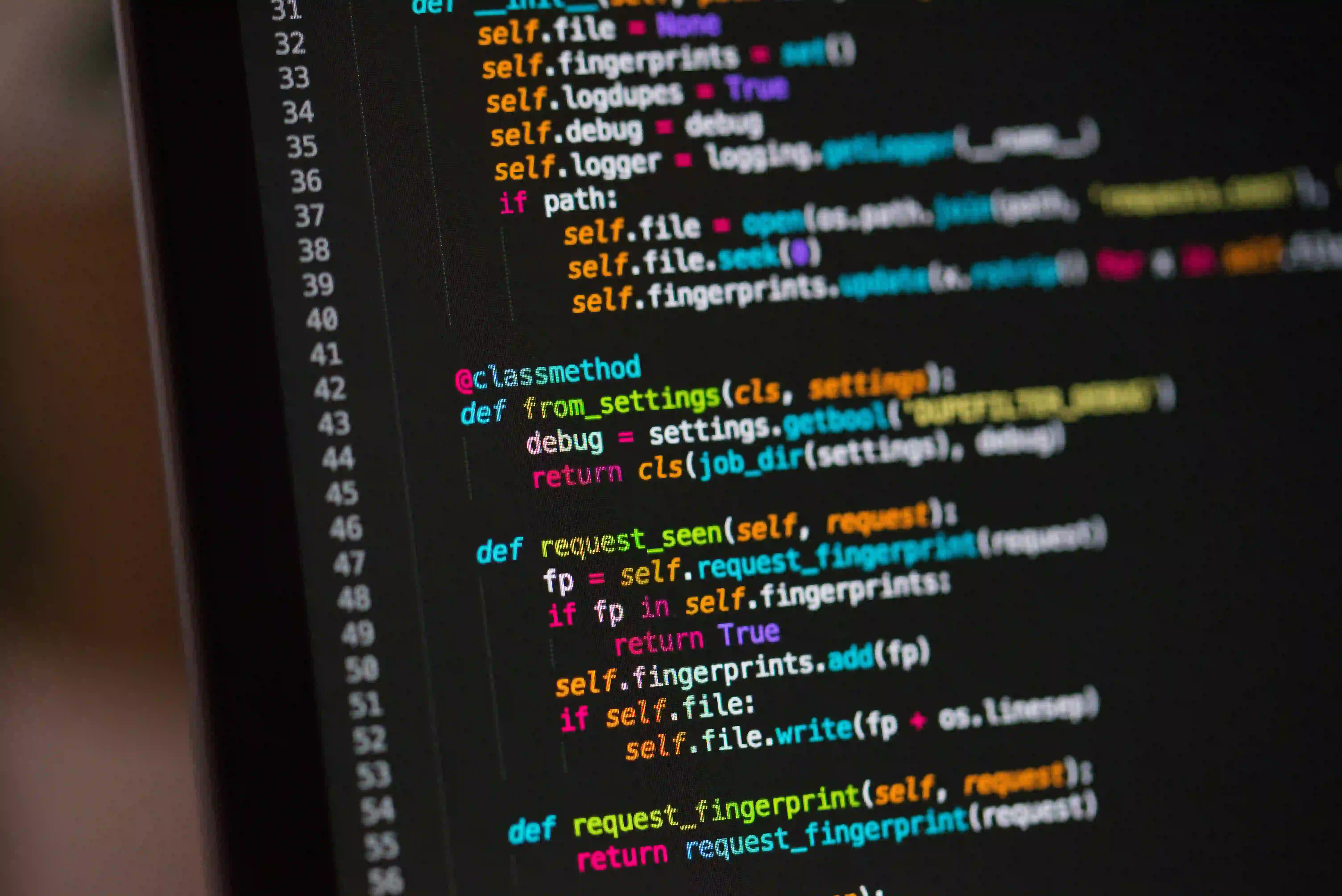
When writing Java code, it's crucial to prioritize readability to ensure that your code is easily understandable and maintainable. One important aspect of writing clean and readable code is using effective method parameter names. Well-named method parameters can significantly enhance the understanding of the code and make it easier for other developers (and your future self) to work with your codebase.
In this article, we'll explore the importance of method parameter names and discuss best practices for naming method parameters in Java. We'll also provide examples to illustrate how meaningful parameter names can improve the readability and maintainability of your Java code.
Importance of Method Parameter Names
Meaningful method parameter names play a vital role in conveying the purpose and usage of a method. When someone reads a method or a function signature, the parameter names should clearly indicate what kind of values the method expects and how those values will be used within the method.
Consider the following method signature:
public void calculateTotal(int a, int b) {
// Method implementation
}
In this example, the parameter names a
and b
are not descriptive. This might lead to confusion about the purpose of these parameters, especially if the method implementation is complex or if the method is called in various contexts throughout the codebase.
Now, let's see how meaningful parameter names can enhance the readability and understanding of the method:
public void calculateTotal(int quantity, int price) {
// Method implementation
}
By using descriptive names like quantity
and price
, the purpose of the parameters becomes immediately clear, even without looking at the method implementation. This simple change can make a significant difference in how easily developers can comprehend and use the method.
Best Practices for Naming Method Parameters
When naming method parameters in Java, it's essential to follow best practices to ensure consistency and clarity throughout your codebase. Here are some best practices for naming method parameters:
-
Use Descriptive Names: Always use descriptive names that accurately represent the purpose of the parameter. The name should reflect the kind of value the method expects and how that value will be used within the method.
-
Avoid Single-letter Names: Resist the temptation to use single-letter names like
a
,b
,x
, ory
for parameters. While these names may seem convenient, they are not descriptive and can lead to confusion, especially in larger codebases or when revisiting the code after a period of time. -
Be Consistent: Maintain consistency in parameter naming across methods in your codebase. If a similar concept is represented by a particular name in one method, try to use the same name for similar concepts in other methods.
-
Use Camel Case: Following the standard Java naming conventions, use camel case for parameter names (e.g.,
parameterName
). This aligns with the expected naming style in Java code and contributes to the overall readability.
Example: Improving Readability with Meaningful Parameter Names
Let's consider a practical example to demonstrate the impact of meaningful parameter names on code readability. Suppose we have a method that calculates the total price of items based on their quantity and unit price.
Here's an initial implementation with poorly named parameters:
public double calculateTotal(int x, int y) {
// Method implementation
}
The method signature and the parameter names (x
and y
) provide little information about the purpose of these parameters. Now, let's refactor the method to use meaningful parameter names:
public double calculateTotal(int quantity, int unitPrice) {
// Method implementation
}
With the improved parameter names quantity
and unitPrice
, it's immediately clear what values the method expects and how they will be used to calculate the total. This clarity significantly enhances the method's readability and makes it easier for developers to understand and use the method without delving into the implementation details.
Lessons Learned
In conclusion, using effective method parameter names is a simple yet powerful way to enhance the readability and maintainability of your Java code. By choosing descriptive names that accurately represent the purpose of the parameters, you can make your code more understandable to others and your future self. Consistency in naming and following best practices for naming method parameters will contribute to a more cohesive and readable codebase.
By prioritizing meaningful parameter names, you can contribute to the overall professionalism and quality of your Java code, and ultimately make the development and maintenance processes smoother for everyone involved.
Remember, code is read more often than it is written. Therefore, investing time in choosing appropriate method parameter names is a small effort that pays off in the long run.