Maximizing Performance with Hibernate Query Caching
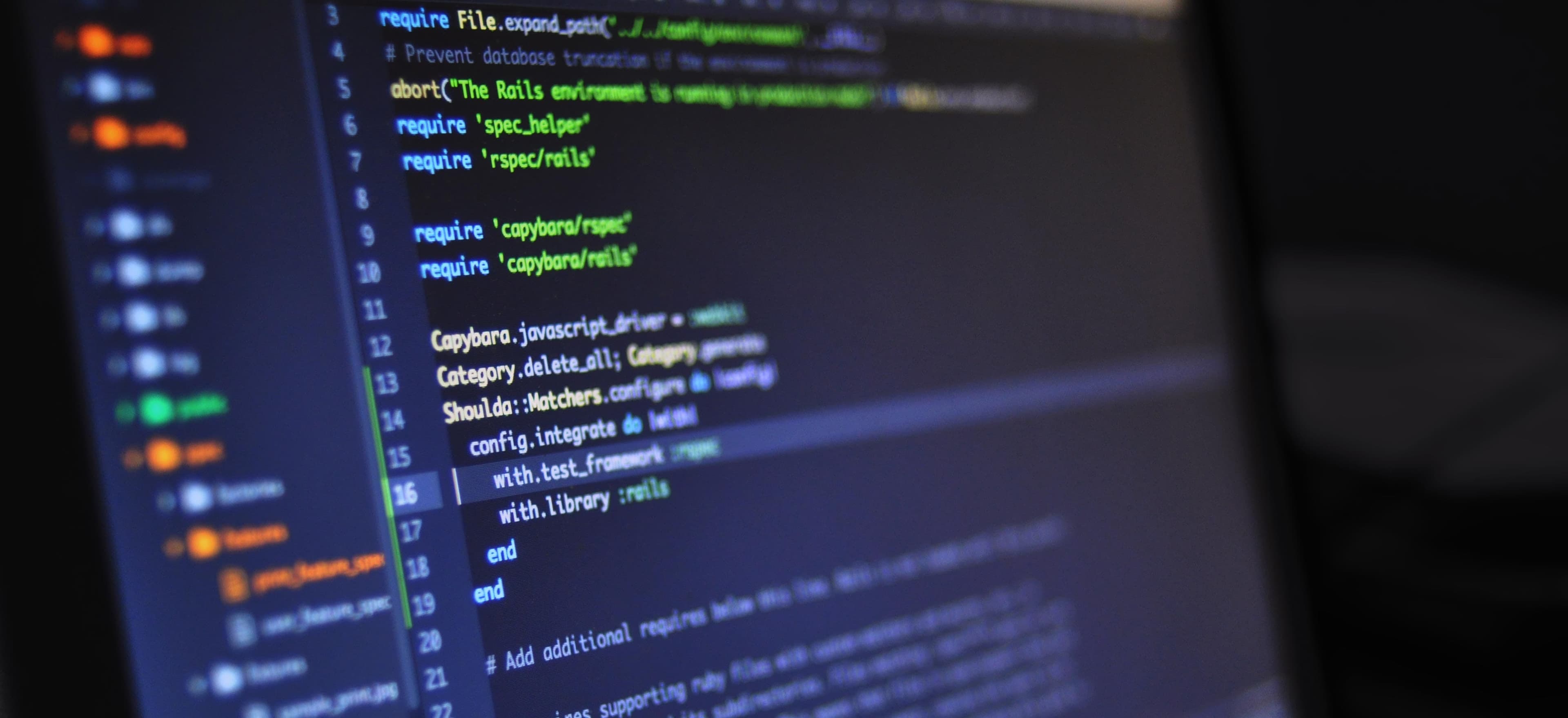
- Published on
Maximizing Performance with Hibernate Query Caching
When developing applications with Hibernate, it’s essential to maximize performance where possible. One effective way to achieve this is through query caching. Hibernate query caching can significantly improve application performance by caching the results of database queries, thereby reducing the number of round trips to the database.
In this article, we'll explore Hibernate query caching in depth, covering its benefits, implementation, best practices, and potential pitfalls. By the end, you’ll have a solid understanding of how to leverage Hibernate query caching to boost the performance of your Java applications.
Understanding Hibernate Query Caching
Hibernate, a popular object-relational mapping (ORM) framework for Java, provides robust support for caching to minimize database interactions. Hibernate query caching, in particular, focuses on caching the results of queries executed against the database.
When a query's results are cached, subsequent executions of the same query can retrieve the results from the cache rather than hitting the database again. This can lead to a significant performance boost, especially for frequently executed or resource-intensive queries.
Benefits of Hibernate Query Caching
1. Reduced Database Load
By caching query results, Hibernate minimizes the number of database requests, thereby reducing the load on the database server. This is especially beneficial in applications with complex query patterns and high user concurrency.
2. Improved Response Times
Caching query results can drastically improve response times for repetitive read-heavy operations. This is particularly advantageous in scenarios where the same read queries are executed frequently with relatively static data.
3. Enhanced Scalability
Query caching can contribute to better scalability by allowing more frequent reuse of cached results, enabling the application to handle a larger user base and higher throughput with existing resources.
Implementing Hibernate Query Caching
Implementing query caching in Hibernate involves the following key steps:
1. Enabling the Second-Level Cache
Before utilizing query caching, ensure that the second-level cache is enabled in Hibernate. This can be achieved through configuration settings in the persistence.xml
file or hibernate.cfg.xml
file, depending on whether you are using JPA or pure Hibernate.
2. Marking Entities and Collections for Caching
Entities and collections that are frequently queried should be marked for caching using Hibernate's @Cacheable
annotation or XML configuration. By doing so, results obtained from querying these entities can be cached for subsequent use.
3. Enabling Query Caching
To enable query caching for specific queries, utilize Hibernate's setCacheable(true)
method when creating query objects. This instructs Hibernate to cache the results of the query, provided that query caching is enabled and properly configured.
4. Configuring Cache Providers
Hibernate supports multiple cache providers such as Ehcache, Infinispan, and Hazelcast. Choose a suitable cache provider and configure it to work in tandem with Hibernate's query caching mechanism.
5. Monitoring Cache Utilization
It's crucial to monitor and analyze cache utilization to ensure that the cache is effectively improving performance. Tools like JMX, which integrates with various cache providers, can be invaluable for monitoring cache statistics and performance metrics.
Best Practices for Hibernate Query Caching
To maximize the effectiveness of Hibernate query caching, adhere to the following best practices:
1. Identify Query Hotspots
Identify the most frequently executed and resource-intensive queries in your application. These are the prime candidates for query caching and can yield the most significant performance gains.
2. Optimize Cache Expiry Policies
Tailor the expiry policies of cached query results based on data volatility and query patterns. Set an appropriate expiration time or implement eviction policies to ensure that the cache remains relevant and efficient.
3. Leverage Query Result Set Caching
Consider caching not only the query results but also the entire result set. This can be useful when the same query is used with different parameters, reducing redundant query processing.
4. Beware of Cache Invalidation
Be mindful of cache invalidation to prevent serving stale data. Properly manage cache eviction and invalidation to ensure data consistency and integrity.
Potential Pitfalls and Considerations
While Hibernate query caching offers notable benefits, there are potential pitfalls and considerations to keep in mind:
1. Overcaching
Overcaching can lead to increased memory usage and potential performance degradation. Carefully evaluate the necessity of caching each query result and strike a balance between caching benefits and overhead.
2. Cache Synchronization
When using a distributed cache, consider the implications of cache synchronization across multiple nodes to maintain consistency and coherence.
3. Query Parameterization
Be cautious when caching queries with parameters, as different parameter values may yield different results. Ensure that cached results are specific to the exact query parameters.
4. Testing and Benchmarking
Thoroughly test and benchmark the performance impact of query caching in various scenarios to validate its efficacy and identify any unforeseen drawbacks.
Example Usage of Hibernate Query Caching
Let's consider a simple example to demonstrate the usage of Hibernate query caching. Suppose we have an entity Product
that is frequently queried, and we want to enable caching for the retrieval of all products priced above a certain threshold:
Query query = session.createQuery("FROM Product WHERE price > :threshold");
query.setParameter("threshold", 100.0);
query.setCacheable(true);
List<Product> products = query.list();
In this example, by invoking query.setCacheable(true)
, we instruct Hibernate to cache the results of the query, provided that query caching is enabled and configured.
A Final Look
Hibernate query caching is a powerful mechanism for optimizing application performance by reducing database overhead and improving response times. By leveraging query caching effectively and adhering to best practices, developers can significantly enhance the scalability and responsiveness of their Hibernate-based applications.
Understanding the benefits, implementation steps, best practices, and potential pitfalls of Hibernate query caching is essential for making informed decisions on when and how to integrate query caching into your applications. By doing so, you can unlock substantial performance gains and deliver superior user experiences.
References:
Checkout our other articles