Maximizing Test Coverage in Software Development
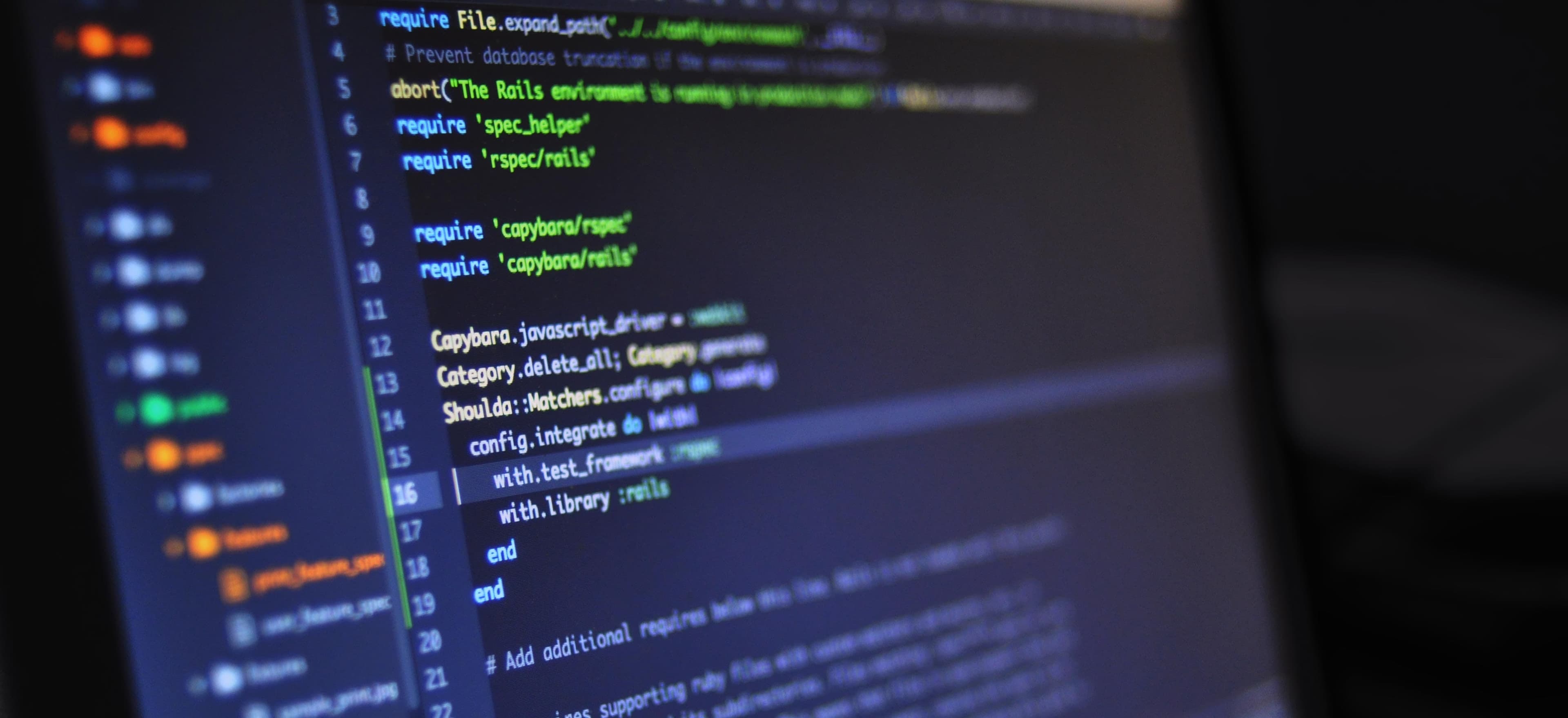
- Published on
Maximizing Test Coverage in Software Development
In the world of software development, testing is a crucial aspect of ensuring the quality and reliability of the final product. One of the key metrics used to measure the effectiveness of testing is test coverage. Test coverage indicates the percentage of your codebase that is exercised by your test suite. In this blog post, we will delve into the concept of test coverage, its importance, and strategies for maximizing test coverage in Java software development.
Understanding Test Coverage
Test coverage is a metric that measures the extent to which your code is tested by your test suite. It is typically expressed as a percentage, representing the portion of your codebase that is executed during the running of your tests. A high test coverage percentage indicates that a large portion of your codebase is being exercised by your tests, while a low test coverage percentage implies that there are untested or under-tested portions of your code.
Importance of Test Coverage
High test coverage is desirable for several reasons. Firstly, it instills confidence in the stability and correctness of your codebase. When a significant portion of your code is covered by tests, you can be more certain that changes or refactoring will not introduce unexpected bugs. Additionally, high test coverage facilitates early bug detection, as changes that break existing functionality are more likely to be caught by the test suite. Moreover, a comprehensive test suite can serve as living documentation, showcasing the expected behavior of your code.
Strategies for Maximizing Test Coverage in Java
Now that we understand the significance of test coverage, let’s explore some strategies for maximizing it in Java software development.
1. Identify Critical Code Paths
Analyze your codebase to identify critical code paths. Focus on testing the code that is most essential to the functioning of your application. This may include complex algorithms, error-prone modules, or frequently used components.
2. Utilize Code Coverage Tools
Java provides several code coverage tools such as JaCoCo, Cobertura, and Emma. These tools can help you visualize which portions of your code are covered by tests and identify areas that require additional testing. Integrating a code coverage tool into your build process can provide insightful metrics to guide your testing efforts.
3. Write Unit Tests
Unit tests form the foundation of a robust test suite. Write focused unit tests for individual components, aiming to cover different code paths, edge cases, and error scenarios. By thoroughly testing the smallest units of code, you can achieve a high degree of test coverage at the granular level.
@Test
public void testCalculateTotalPrice() {
ShoppingCart cart = new ShoppingCart();
cart.addItem(new Item("ABC123", 2, 25.0));
cart.addItem(new Item("DEF456", 1, 10.0));
double totalPrice = cart.calculateTotalPrice();
assertEquals(60.0, totalPrice, 0.01);
}
In the code snippet above, we have a unit test for the calculateTotalPrice
method of a shopping cart. This test covers a specific functionality and ensures that the method behaves as expected.
4. Employ Integration Tests
In addition to unit tests, integration tests are valuable for testing interactions between different components of your application. Use integration tests to validate the behavior of interconnected modules and to verify that different parts of your application work together seamlessly.
@Test
public void testUserAuthentication() {
// Setup test user
User testUser = new User("testuser", "password");
// Simulate user authentication process
AuthenticationService authService = new AuthenticationService();
boolean isAuthenticated = authService.authenticate(testUser);
assertTrue(isAuthenticated);
}
The above integration test examines the user authentication process by verifying that a test user can successfully authenticate.
5. Leverage Mocking Frameworks
Mocking frameworks such as Mockito or PowerMock can be employed to simulate the behavior of dependencies and external systems, allowing you to isolate the code under test. This is particularly useful in scenarios where certain dependencies are difficult to replicate in a testing environment.
@Test
public void testOrderProcessing() {
// Setup mock OrderService
OrderService mockOrderService = mock(OrderService.class);
when(mockOrderService.processOrder(any(Order.class))).thenReturn(true);
// Exercise the code under test
ShoppingCart cart = new ShoppingCart();
boolean orderPlaced = cart.placeOrder(mockOrderService);
assertTrue(orderPlaced);
}
In the above test, Mockito is used to create a mock OrderService
to simulate the behavior of the actual service, enabling focused testing of the placeOrder
method in the ShoppingCart
class.
6. Continuous Integration and Code Reviews
Integrate automated test runs into your continuous integration (CI) pipeline to regularly monitor test coverage and to prevent regressions. Additionally, leverage code reviews as a means to ensure that new code contributions are accompanied by corresponding tests, thus promoting the continual improvement of test coverage.
A Final Look
In conclusion, test coverage is a vital aspect of software development that directly influences the reliability and maintainability of your codebase. By identifying critical code paths, writing comprehensive unit and integration tests, leveraging mocking frameworks, and integrating code coverage tools into your workflow, you can maximize test coverage in your Java projects. Adopting these strategies can bolster the quality of your software, mitigate the introduction of bugs, and build confidence in the stability of your application.
Maximizing test coverage requires an ongoing commitment to testing and a dedication to continuously improving the quality of your test suite. By incorporating these strategies into your development process, you can enhance the robustness of your Java applications and deliver higher-quality software to your users. Embrace the challenge of maximizing test coverage and reap the benefits of a more resilient and dependable codebase.
Remember, a well-tested codebase is the foundation of a successful software application.
For more in-depth knowledge on maximizing test coverage in Java, you can refer to resources like Oracle's Java Tutorials and JUnit documentation. Happy testing!