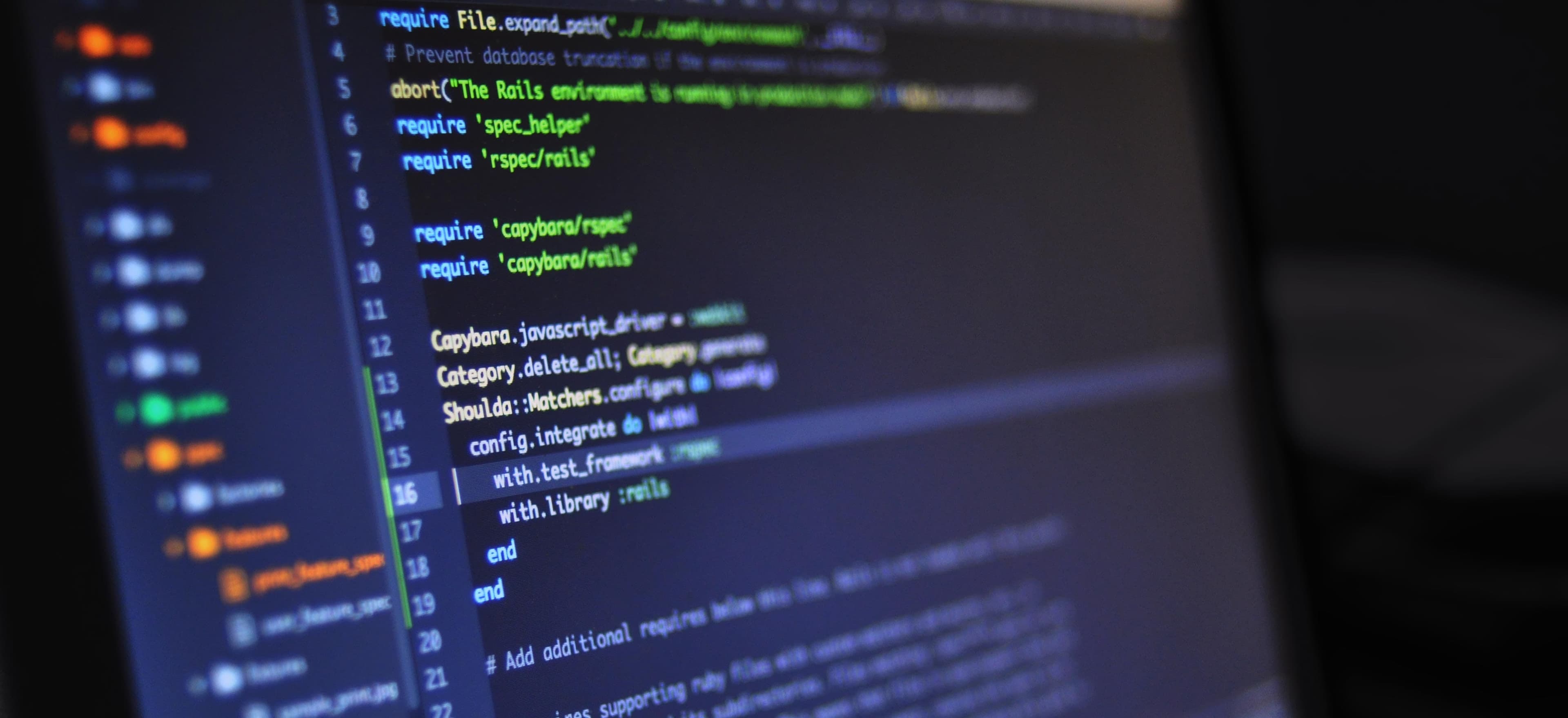
- Published on
Introduction
In the world of developing RESTful APIs with Spring, one of the common hurdles developers face is dealing with Cross-Origin Resource Sharing (CORS). CORS is a security feature implemented in web browsers that restricts web pages from making requests to a different domain than the one that served the original web page. This restriction is important for preventing certain types of attacks, but it often poses a challenge when developing and consuming RESTful APIs.
In this article, we will delve into the concept of CORS, discuss its implications, and then explore how to handle CORS in Spring when building RESTful APIs.
Understanding CORS
When a web page makes a request to a different domain than the one it came from, it is referred to as a cross-origin request. Browsers, by default, restrict such requests for security reasons. This restriction helps in preventing unauthorized access to sensitive data. However, with the rise of client-side technologies such as JavaScript frameworks and single-page applications, it has become increasingly common for web applications to require access to resources from different origins.
This is where CORS comes into play. CORS is a mechanism that allows servers to declare which origins are permitted to access its resources. It is implemented using HTTP headers that the server sends along with the response. When a browser receives a cross-origin request, it conducts a preflight request using the OPTIONS method to determine if the actual request is safe to send. The server then responds with the appropriate CORS headers to indicate whether the request is permitted.
The Implications of CORS for RESTful APIs
For developers working with RESTful APIs, understanding and dealing with CORS is crucial. When developing the backend of an application with Spring, you may encounter situations in which the API server needs to respond to requests from origins other than its own. This is especially true when building a decoupled architecture with a separate client-side application consuming the API.
Failure to handle CORS properly can result in frustrating errors such as the famous "No 'Access-Control-Allow-Origin' header is present on the requested resource" message in the browser console. These errors can impede the integration of the API with client applications and lead to a poor user experience.
Handling CORS in Spring
Now that we comprehend the significance of CORS, let's explore how to handle it in a Spring-based RESTful API. Spring provides simple yet powerful mechanisms to address CORS-related issues.
Using @CrossOrigin Annotation
For simple CORS requirements, Spring offers the @CrossOrigin
annotation, which can be applied at the controller level or to specific handler methods.
@RestController
@CrossOrigin("http://localhost:3000")
@RequestMapping("/api")
public class ExampleController {
// Handler methods
}
In this example, the @CrossOrigin
annotation allows requests from http://localhost:3000
to access the resources served by the ExampleController
. While this approach is straightforward, it may not be suitable for complex CORS configurations that involve variations in HTTP methods and headers.
Global CORS Configuration
For more advanced CORS setups, Spring allows you to define global CORS configuration using a WebMvcConfigurer
. This approach provides greater control over CORS settings and is well-suited for applications with diverse CORS requirements.
@Configuration
public class CorsConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**")
.allowedOrigins("http://localhost:3000")
.allowedMethods("GET", "POST", "PUT", "DELETE")
.allowedHeaders("Authorization", "Content-Type")
.exposedHeaders("Header1", "Header2")
.allowCredentials(true);
}
}
With this configuration, all endpoints under /api
will allow requests from http://localhost:3000
with specific HTTP methods, headers, and exposed headers. It's important to note the use of allowCredentials(true)
, which specifies that the browser should include any credentials (e.g., cookies, HTTP authentication) in the CORS request.
Preflight Requests and Caching
When a client sends a cross-origin request with certain characteristics (e.g., custom headers or non-simple methods), the browser will first issue a preflight request to determine the server's CORS policy. This preflight request includes the HTTP method and headers to be used in the actual request. For performance reasons, browsers cache the results of preflight requests for a certain period of time.
In Spring, you can customize the CORS preflight caching behavior using the CorsConfiguration
class. This allows you to control how long the preflight results are cached in the client's browser.
@Configuration
public class CorsConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
CorsConfiguration config = new CorsConfiguration();
config.applyPermitDefaultValues();
config.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "DELETE"));
config.setMaxAge(3600L);
registry.addMapping("/api/**").allowedOrigins("http://localhost:3000").allowCredentials(true).exposedHeaders("Header1", "Header2").addMapping("/api/**").addMapping("/**").allowedOrigins("*").allowCredentials(false).configuration(config);
}
}
In this configuration, config.setMaxAge(3600L)
sets the maximum amount of time in seconds that the results of a preflight request can be cached. Adjusting this value can impact the number of preflight requests sent by the browser, ultimately affecting the performance of your API.
Handling Preflight Requests for Custom Endpoints
When implementing custom endpoints or non-standard HTTP methods, it's essential to ensure that the server responds correctly to preflight requests for these endpoints. Spring provides the @RequestMapping
and @CrossOrigin
annotations to specify the CORS configuration for custom endpoints.
@RestController
public class CustomController {
@CrossOrigin(
origins = "http://localhost:3000",
methods = {RequestMethod.GET, RequestMethod.POST, RequestMethod.OPTIONS},
allowedHeaders = {"Authorization", "Content-Type"},
exposedHeaders = {"Header1", "Header2"},
allowCredentials = "true",
maxAge = 3600
)
@RequestMapping(value = "/custom-endpoint", method = RequestMethod.CUSTOM_METHOD)
public ResponseEntity<String> handleCustomRequest() {
// Handler logic
}
}
In this example, the @CrossOrigin
annotation is used in conjunction with @RequestMapping
to configure the CORS settings for the CustomController
. It specifies the allowed origins, methods, headers, exposed headers, whether credentials are allowed, and the maximum age for caching preflight results.
The Closing Argument
In modern web development, dealing with CORS is an unavoidable aspect of building and consuming RESTful APIs. Spring equips us with flexible and effective tools to manage and mitigate CORS-related challenges. Whether it's through simple annotations, global configurations, caching optimizations, or custom endpoint settings, Spring offers a comprehensive suite of solutions to ensure seamless CORS handling in our RESTful APIs.
Incorporating these techniques not only enhances the interoperability of our APIs with client applications but also contributes to a smoother and more secure user experience. As always, understanding the nuances of CORS and staying abreast of best practices is essential for developers working in the realm of web technologies.