Improving Test Reporting for Effective Continuous Testing
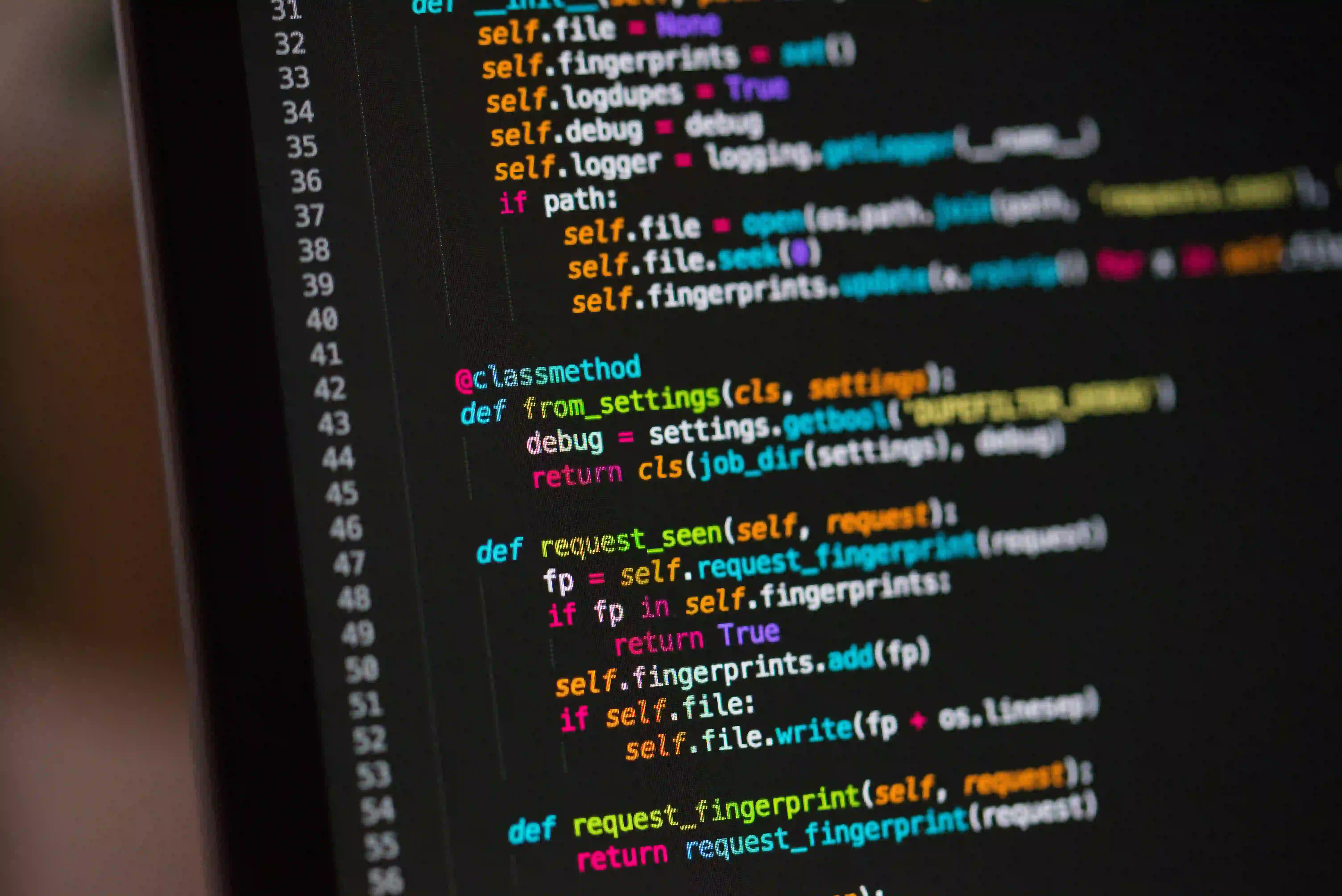
Improving Test Reporting for Effective Continuous Testing
Continuous testing has become an essential part of the software development lifecycle, ensuring that any changes made to the codebase do not negatively impact the existing functionality. A crucial aspect of continuous testing is the ability to generate detailed and actionable test reports. In this blog post, we will explore how to enhance test reporting in Java to achieve more effective continuous testing.
The Importance of Test Reporting
Test reporting plays a pivotal role in continuous testing by providing visibility into the test results, identifying any potential issues, and facilitating informed decision-making. It enables development teams to assess the quality of the codebase, track the progress of test suites, and pinpoint areas that require attention or improvement.
When it comes to Java, the ecosystem offers a variety of frameworks and libraries that can be leveraged to enhance test reporting capabilities. By incorporating these tools into the testing process, teams can streamline the generation of comprehensive test reports and extract valuable insights from the test results.
Leveraging ExtentReports for Enhanced Test Reporting
One popular option for improving test reporting in Java is to integrate ExtentReports, a versatile reporting library that provides rich and interactive reports for test automation. ExtentReports offers a user-friendly interface, customizable report designs, and support for a wide range of frameworks including TestNG and JUnit.
To get started with ExtentReports, begin by adding the library as a dependency in your Maven project:
<dependency>
<groupId>com.aventstack</groupId>
<artifactId>extentreports</artifactId>
<version>5.0.7</version>
</dependency>
Next, you can create an instance of ExtentReports and configure the report settings according to your preferences. This includes defining report names, document types, and additional information such as build numbers or environment details.
Here's an example of initializing ExtentReports:
ExtentReports extent = new ExtentReports();
extent.setSystemInfo("Environment", "QA");
extent.setSystemInfo("Build Number", "1.0");
ExtentHtmlReporter htmlReporter = new ExtentHtmlReporter("extent-report.html");
extent.attachReporter(htmlReporter);
Now, you can use ExtentReports to create test logs, add test information, and capture screenshots or other media to enrich the report content. Upon executing your test suite, ExtentReports will generate a visually appealing and comprehensive report that encapsulates the test results and related metadata.
Generating Custom Metrics and Insights
In addition to standard test reporting features, it's often beneficial to incorporate custom metrics and insights into test reports to gain deeper visibility into the quality and performance of the application under test.
To achieve this in Java, consider leveraging libraries such as Gauge or Prometheus to capture and record application-specific metrics during test execution. These metrics can range from response times and throughput to error rates and resource utilization, providing a holistic view of the system's behavior under varying conditions.
By integrating custom metrics into test reporting, teams can identify performance bottlenecks, assess the impact of code changes on system resources, and make data-driven decisions to optimize application behavior.
Visualizing Test Reports with Allure Framework
Another compelling option for enhancing test reporting in Java is to adopt the Allure Framework, a powerful tool for creating detailed and visually appealing test reports. Allure seamlessly integrates with popular testing libraries such as TestNG, JUnit, and Cucumber, making it a versatile choice for diverse testing environments.
To integrate Allure into your Java project, add the Allure TestNG dependency to your Maven configuration:
<dependency>
<groupId>io.qameta.allure</groupId>
<artifactId>allure-testng</artifactId>
<version>2.14.0</version>
<scope>test</scope>
</dependency>
Once the dependency is in place, you can annotate your test methods with Allure-specific annotations to capture additional metadata and attach supplementary information to the test reports. Allure provides annotations for marking test status, adding descriptions, tagging tests, and attaching files or screenshots.
Here's an example of using Allure annotations in a TestNG test method:
@Test
@Description("Verify login functionality with valid credentials")
@Severity(SeverityLevel.CRITICAL)
public void testLoginWithValidCredentials() {
// Test code goes here
}
After running your tests, you can generate the Allure report by executing the following command:
mvn allure:report
The generated report will contain detailed information about test execution, including test steps, attached artifacts, and any additional context provided through annotations. Allure's visually appealing interface and intuitive design make it easier for teams to analyze test results and derive actionable insights.
The Bottom Line
Effective test reporting is essential for enabling continuous testing practices to thrive within software development teams. By leveraging tools such as ExtentReports, custom metrics, and the Allure Framework, Java developers can elevate their test reporting capabilities and gain deeper insights into the quality and performance of their applications.
Incorporating these reporting enhancements not only improves the visibility of test results but also empowers teams to make data-driven decisions, identify areas for improvement, and ensure the reliability and resilience of their software products.
Continuous testing, supported by robust and insightful test reporting, forms the bedrock of high-quality software delivery, and Java developers are well-equipped with the tools and libraries to establish and maintain effective continuous testing practices.
Enhancing test reporting is not just a matter of pretty visualizations; it's about gaining actionable insights from test results to drive continuous improvement and build robust, reliable software systems.
By embracing these reporting enhancements in Java, teams can reinforce their commitment to quality and accelerate the delivery of valuable software to their users.
For further insights on the importance of continuous testing, you may find this article on The Future of Continuous Testing insightful. Additionally, for an in-depth dive into ExtentReports, refer to the official ExtentReports documentation.