Overcoming Mockito: Instantiating Abstract Class for @InjectMocks
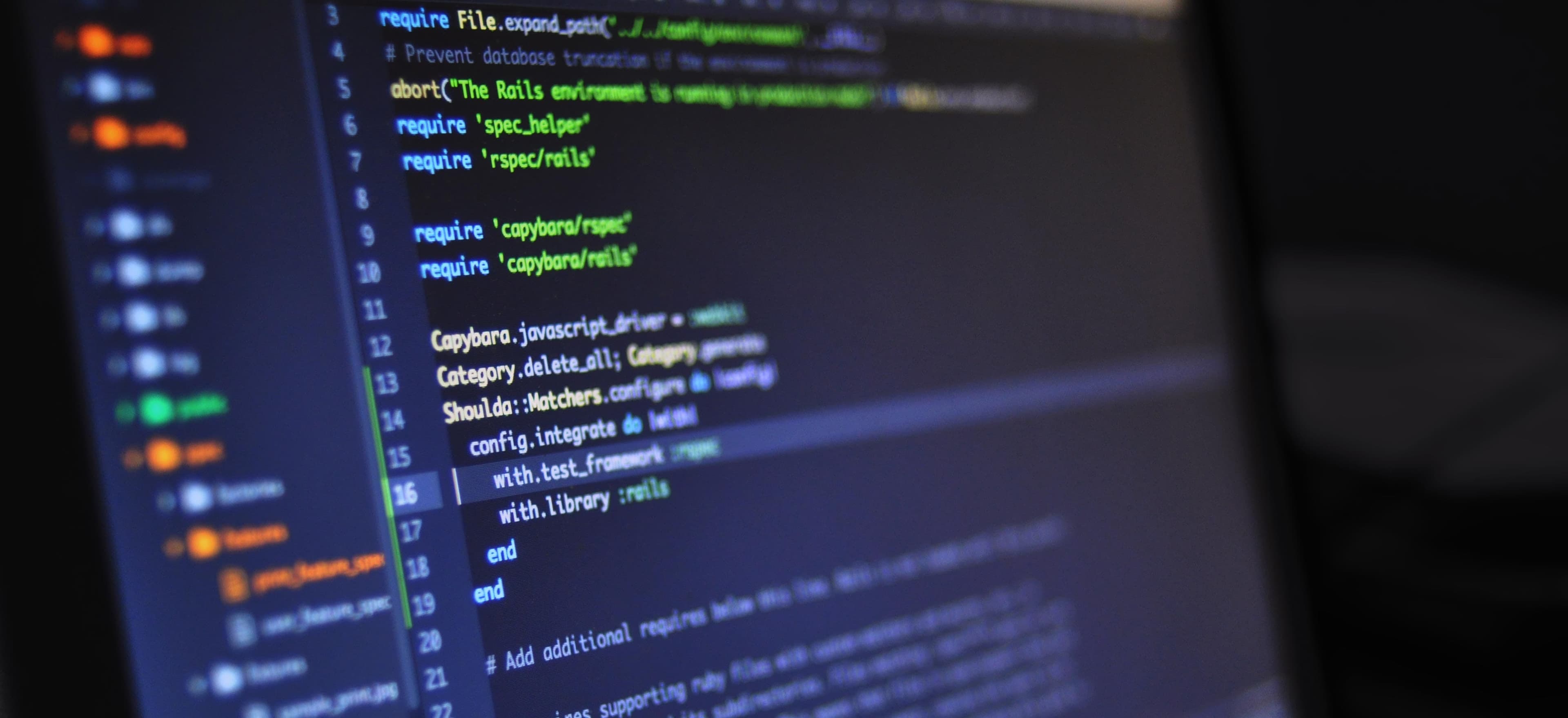
- Published on
Overcoming Mockito: Instantiating Abstract Class for @InjectMocks
When working with Mockito for unit testing in Java, you might encounter a situation where you need to instantiate an abstract class for the @InjectMocks annotation. Mockito is a powerful framework for creating mock objects, but it has its limitations when it comes to working with abstract classes. In this article, we will explore how to overcome this limitation and instantiate an abstract class for @InjectMocks in your unit tests.
Understanding @InjectMocks and Abstract Classes
Before we dive into solving the problem, let's have a brief understanding of @InjectMocks and abstract classes in Java.
@InjectMocks
@InjectMocks is a Mockito annotation used to inject mock or spy fields into the tested object automatically. It is helpful in creating unit tests where you want to inject mock dependencies into the class under test.
Abstract Classes
Abstract classes in Java cannot be instantiated directly as they may contain abstract methods that need to be implemented by a subclass. They are designed to be extended by concrete subclasses that provide implementations for the abstract methods.
The Challenge
When you have a class that has a dependency on an abstract class, using @InjectMocks becomes challenging since Mockito does not directly support mocking abstract classes. If the class under test directly instantiates the abstract class, it becomes hard to mock it using Mockito.
Let's consider the following scenario:
public abstract class AbstractService {
public abstract String process(String input);
}
public class MyBusinessService {
private AbstractService abstractService;
public MyBusinessService(AbstractService abstractService) {
this.abstractService = abstractService;
}
public String execute(String input) {
return abstractService.process(input);
}
}
Here, MyBusinessService
has a dependency on AbstractService
, an abstract class. Our goal is to unit test MyBusinessService
by injecting a mocked instance of AbstractService
using @InjectMocks.
Overcoming the Limitation
To overcome the limitation of instantiating an abstract class for @InjectMocks, we can use the MockitoAnnotations class provided by Mockito. The MockitoAnnotations.initMocks() method can be used to initialize objects annotated with Mockito annotations such as @InjectMocks.
Let's see how we can achieve this by creating a unit test for MyBusinessService
where we inject a mocked instance of AbstractService
.
import org.junit.Before;
import org.junit.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.when;
public class MyBusinessServiceTest {
@InjectMocks
private MyBusinessService myBusinessService;
@Mock
private AbstractService abstractService;
@Before
public void init() {
MockitoAnnotations.initMocks(this);
}
@Test
public void testExecute() {
// Arrange
String input = "testInput";
when(abstractService.process(input)).thenReturn("mockedOutput");
// Act
String result = myBusinessService.execute(input);
// Assert
assertEquals("mockedOutput", result);
}
}
In this example, we have used the MockitoAnnotations.initMocks() method in the @Before setup method to initialize the mocks and inject them into the test class.
Why MockitoAnnotations.initMocks()?
-
Initialization of @InjectMocks: The initMocks() method is used to initialize objects annotated with Mockito annotations. It prepares the test class for Mockito injection and sets up the mock objects.
-
Simplifying Test Setup: Using initMocks() simplifies the test setup process by handling the injection of mocked dependencies, including the ones declared with @InjectMocks.
-
Maintainability: By using initMocks(), the test setup remains maintainable even when there are changes to the dependencies or the class under test.
Final Thoughts
In this article, we have explored how to overcome the limitation of instantiating an abstract class for @InjectMocks in Mockito. By using MockitoAnnotations.initMocks(), we were able to inject a mocked instance of the abstract class into the class under test for unit testing.
Understanding Mockito annotations and their usage is essential for writing effective unit tests. MockitoAnnotations.initMocks() is a valuable tool in your unit testing arsenal, especially when dealing with complex dependencies and abstract classes.
By employing these techniques, you can enhance the quality and effectiveness of your unit tests, leading to robust and maintainable code.
Now that you've learned how to overcome this limitation in Mockito, you're one step closer to becoming a proficient unit tester in Java!