Maximizing Service Efficiency Through Cloud-Based Design
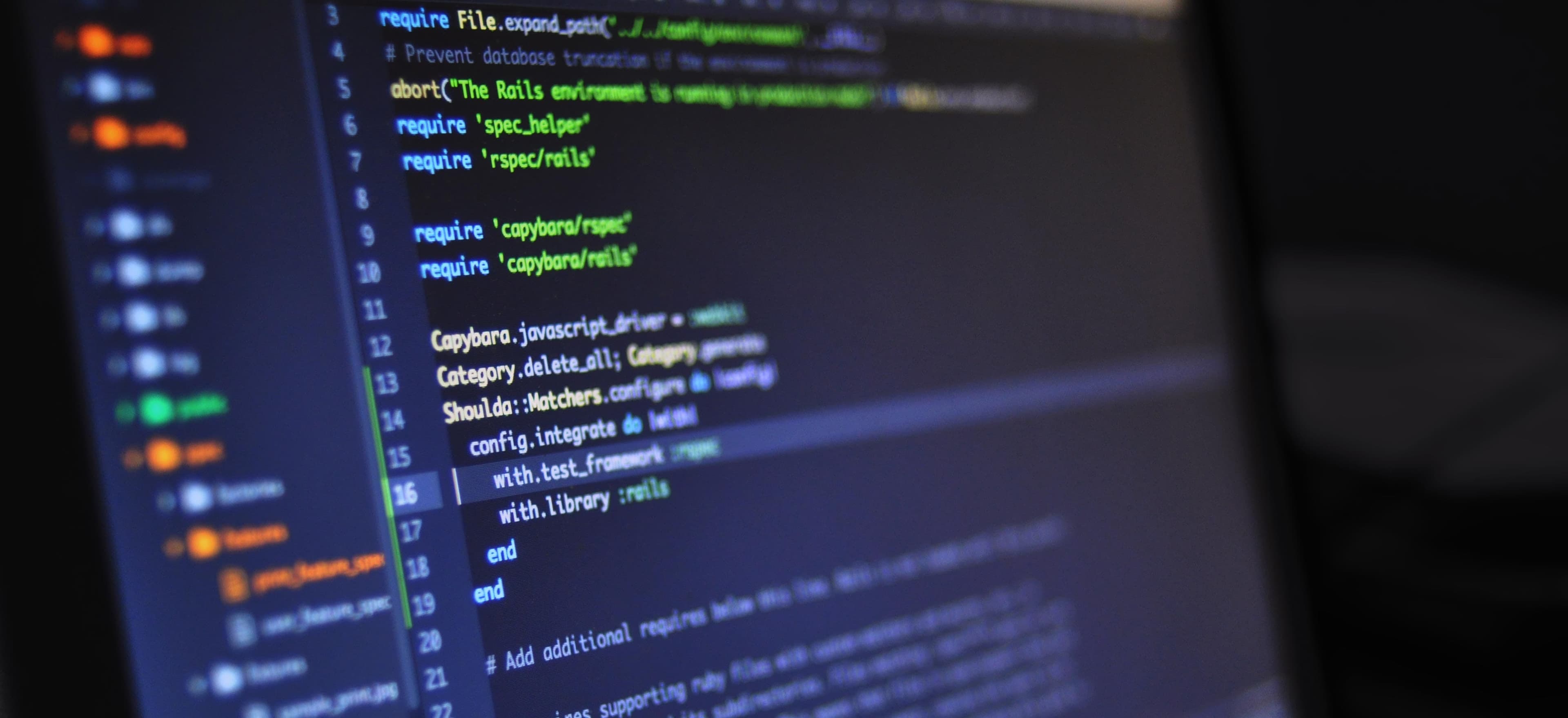
- Published on
Maximizing Service Efficiency Through Cloud-Based Design
In today's fast-paced digital world, businesses need to constantly find ways to improve service efficiency and scalability. One way to achieve this is by leveraging cloud-based design and architecture. By making use of cloud services, businesses can optimize their operations, reduce costs, and ensure high availability and flexibility. Java, as a versatile and popular programming language, plays a key role in developing cloud-based solutions. In this article, we will explore how Java can be utilized to maximize service efficiency through cloud-based design.
Why Cloud-Based Design?
Cloud computing offers a range of benefits including scalability, cost-efficiency, reliability, and security. By moving services and applications to the cloud, businesses can take advantage of on-demand resources, pay-as-you-go pricing models, and global accessibility. Cloud-based design enables businesses to scale their services rapidly based on demand, without the need to provision and manage physical infrastructure. This provides a competitive advantage by allowing businesses to focus on their core offerings while offloading non-core infrastructure management to cloud providers.
Java and Cloud-Native Development
Java has long been a popular choice for building enterprise applications due to its portability, scalability, and robustness. With the rise of cloud-native development, Java has seamlessly adapted to this new paradigm. The use of Java in cloud-native development is supported by a rich ecosystem of libraries, frameworks, and tools that enable developers to build resilient, scalable, and maintainable cloud-based applications.
Leveraging Java for Cloud-Based Design
1. Microservices Architecture
One of the key principles of cloud-native design is the use of microservices architecture, where large applications are broken down into smaller, interconnected services. Java, with its support for frameworks like Spring Boot and Micronaut, provides an ideal platform for building microservices. These frameworks offer features such as dependency injection, auto-configuration, and embedded servers, allowing developers to quickly create and deploy microservices.
// Example of a simple Spring Boot microservice
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
return userService.getUserById(id);
}
// Additional endpoints and logic
}
2. Containerization with Docker
Docker has become the de facto standard for containerization, allowing applications to be packaged with their dependencies and run consistently across different environments. Java applications can be containerized using Docker, providing a lightweight and portable way to deploy them in the cloud. The combination of Java and Docker simplifies the deployment process and ensures consistency from development to production environments.
3. Asynchronous Processing with Java
Cloud-based applications often require asynchronous processing to handle tasks such as message queuing, event-driven architectures, and background processing. Java provides robust support for asynchronous processing through libraries like CompletableFuture, RxJava, and reactive programming frameworks such as Spring WebFlux. By leveraging these capabilities, developers can design responsive and efficient cloud-based systems.
Managing Data in the Cloud with Java
Data management is a critical aspect of cloud-based design. Java offers a variety of tools and frameworks for working with cloud-based data services such as Amazon S3, Google Cloud Storage, and Azure Blob Storage. Libraries like Apache Hadoop, Apache Spark, and Elasticsearch provide the means to process and analyze large-scale data in the cloud. Java's strong ecosystem for data management makes it well-suited for building data-intensive cloud applications.
Ensuring Security and Reliability
Security and reliability are paramount in cloud-based design. Java's extensive set of security features, including standard cryptographic algorithms, secure communication protocols, and authentication mechanisms, make it a trustworthy choice for implementing secure cloud applications. Furthermore, Java's robust error handling, exception management, and monitoring capabilities contribute to building reliable and resilient cloud services.
Wrapping Up
In conclusion, Java provides a powerful and versatile foundation for maximizing service efficiency through cloud-based design. By embracing cloud-native development practices and leveraging Java's extensive ecosystem, businesses can build scalable, resilient, and secure cloud-based applications. With the rapid evolution of cloud technologies, Java continues to adapt and thrive as a leading language for cloud-based design and development.
If you are interested in diving deeper into cloud-based design with Java, check out the Java Cloud Development resources.
Remember, embracing cloud-based design with Java is not just about efficiency – it's about future-proofing your services and staying ahead in the digital age.
Checkout our other articles