Efficient Alternatives for GWT HTTP Requests
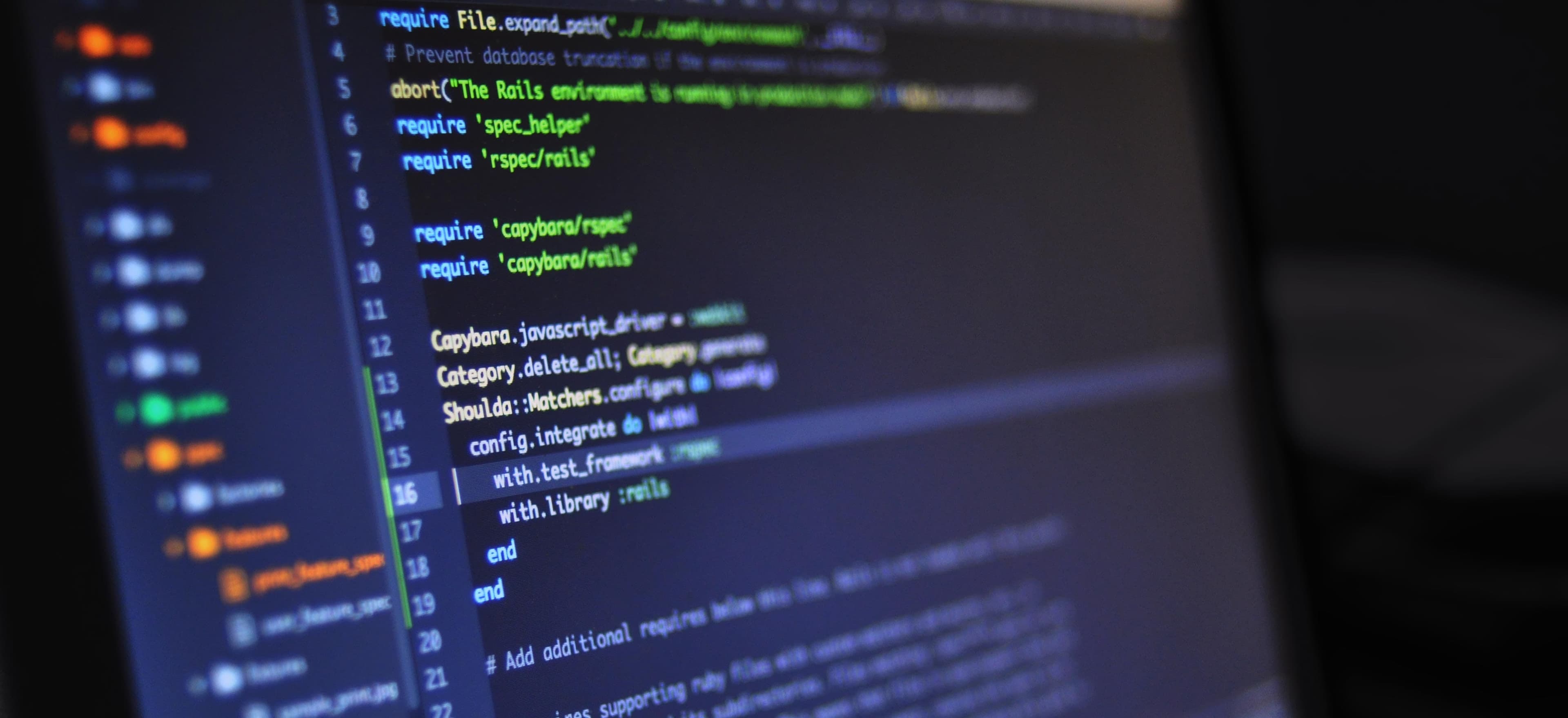
- Published on
Efficient Alternatives for GWT HTTP Requests
When working with GWT (Google Web Toolkit) applications, making efficient HTTP requests is crucial for ensuring a smooth user experience. While GWT provides its own built-in mechanism for making HTTP requests, there are alternative approaches that can offer better performance, flexibility, and ease of use. In this article, we'll explore some efficient alternatives for making HTTP requests in GWT applications.
The Problem with GWT's Default RequestBuilder
GWT's default way of making HTTP requests is through the RequestBuilder
class. While this approach is functional, it has some drawbacks in terms of verbosity and lack of modern features.
RequestBuilder builder = new RequestBuilder(RequestBuilder.GET, "https://api.example.com/data");
try {
builder.sendRequest(null, new RequestCallback() {
@Override
public void onResponseReceived(Request request, Response response) {
if (response.getStatusCode() == 200) {
// Process the response
} else {
// Handle the error
}
}
@Override
public void onError(Request request, Throwable exception) {
// Handle the error
}
});
} catch (RequestException e) {
// Handle the exception
}
The above code snippet demonstrates the verbosity of making a simple GET request using RequestBuilder
. The callback-based approach can lead to nested code, making it challenging to read and maintain.
Using JsInterop to Call Fetch API
One efficient alternative is to leverage the Fetch API, which is modern, concise, and provides powerful features for making HTTP requests. By using JsInterop, we can directly call the Fetch API from our GWT Java code.
@JsType(namespace = JsPackage.GLOBAL, name = "fetch", isNative = true)
public class Fetch {
public static native Promise<Response> fetch(String url);
}
With the above JsInterop declaration, we can now make HTTP requests using the Fetch API in a more concise and modern manner.
Fetch.fetch("https://api.example.com/data")
.then(response -> {
if (response.status == 200) {
return response.text();
} else {
throw new RuntimeException("HTTP error: " + response.status);
}
})
.then(data -> {
// Process the response data
return null;
})
.catch_(error -> {
// Handle the error
return null;
});
By using JsInterop to call the Fetch API, we eliminate the verbosity of RequestBuilder
and embrace a more modern approach to making HTTP requests in GWT applications.
Leveraging RestyGWT Library
Another efficient alternative for making HTTP requests in GWT is to use the RestyGWT library. RestyGWT provides a fluent interface for defining RESTful HTTP requests, making the code more readable and maintainable.
public interface DataService extends RestService {
@GET("/data")
void fetchData(MethodCallback<Data> callback);
}
With RestyGWT, we define an interface that represents our RESTful API, and the library handles the HTTP requests under the hood.
RestServiceProxy service = GWT.create(DataService.class);
service.fetchData(new MethodCallback<Data>() {
@Override
public void onSuccess(Method method, Data response) {
// Process the response
}
@Override
public void onFailure(Method method, Throwable exception) {
// Handle the error
}
});
RestyGWT simplifies the process of making RESTful HTTP requests in GWT applications and provides a more elegant and maintainable way of handling responses.
The Last Word
Efficiently making HTTP requests is essential for the performance and user experience of GWT applications. While the default RequestBuilder
provided by GWT is functional, leveraging modern alternatives such as the Fetch API with JsInterop or the RestyGWT library can greatly improve the readability, maintainability, and performance of HTTP requests in GWT applications. By embracing these efficient alternatives, developers can streamline their code and provide a better experience for their users.
In conclusion, when working with GWT applications, it's worth exploring these alternatives to make HTTP requests in a more efficient and modern way. By doing so, developers can simplify their code, improve performance, and enhance the overall user experience of their applications.