Troubleshooting Common Issues with Arquillian 1.0.0.Final
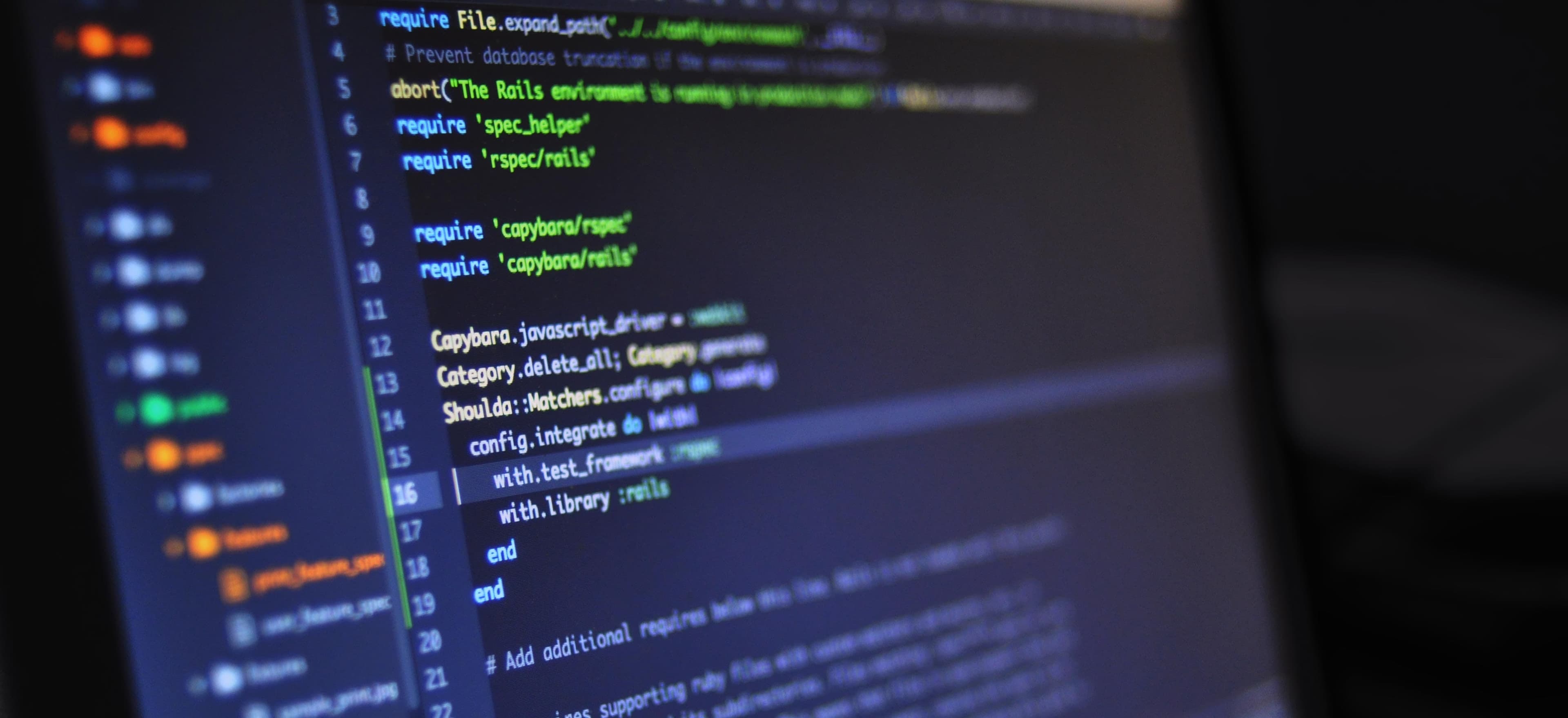
- Published on
Troubleshooting Common Issues with Arquillian 1.0.0.Final
Arquillian is a powerful and flexible testing platform for the Java platform that takes the pain out of testing Java components. However, like any tool, it has its quirks and challenges. This article will guide you through some common issues you might encounter when using Arquillian 1.0.0.Final and provide solutions to overcome them.
Problem 1: ClassNotFoundException when Running Tests
If you encounter a ClassNotFoundException
when running your Arquillian tests, it usually indicates that the necessary classes are not being included in the deployment package. To resolve this issue, ensure that the classes and dependencies required for your test are properly included in the deployment package.
Example:
@Deployment
public static Archive<?> createDeployment() {
return ShrinkWrap.create(WebArchive.class)
.addClasses(HelloWorld.class, GreetingService.class)
.addAsResource("test-persistence.xml", "META-INF/persistence.xml");
}
In this example, the addClasses
method is used to include the HelloWorld
and GreetingService
classes in the deployment package, ensuring that they are available during the test execution.
Problem 2: Container Startup Timeout
Sometimes, the container in which the tests are being executed takes longer than expected to start, leading to a timeout. This can happen due to various reasons such as heavy resource consumption or network issues.
To address this, you can adjust the container startup timeout configuration in the arquillian.xml
file.
Example:
<container qualifier="wildfly-managed" default="true">
<configuration>
<property name="startupTimeoutInSeconds">180</property>
</configuration>
</container>
Increasing the startupTimeoutInSeconds
from the default value of 60 to 180 allows the container more time to start up before Arquillian times out.
Problem 3: Resource Injection Failures
If your test involves injecting resources such as persistence units or EJBs and you encounter failures related to resource injection, it could be due to improper configuration or usage of the resources.
Check that you have declared the correct JNDI names and that the resources are properly deployed and accessible within the testing environment.
Example:
@PersistenceContext
private EntityManager entityManager;
In this example, @PersistenceContext
is used to inject an EntityManager. Ensure that the persistence unit referenced by this annotation is correctly configured and accessible in the test environment.
Problem 4: Maven Dependency Issues
Maven dependency conflicts can often lead to runtime issues when running Arquillian tests. These conflicts can arise from transitive dependencies or different versions of the same library being pulled into the project.
Use Maven dependency management tools to identify and resolve any conflicts or inconsistencies in the dependencies.
Example:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.10.Final</version>
</dependency>
</dependencies>
</dependencyManagement>
In this example, the dependencyManagement
section is utilized to enforce a specific version of the hibernate-core
library, ensuring that any transitive dependencies that may cause conflicts are resolved.
Problem 5: Customizing Test Execution Environment
If your tests require specific configuration or setup in the execution environment, failing to account for these requirements can lead to test failures or unexpected behavior.
Customize the test execution environment according to your test's needs, such as setting system properties or deploying additional resources.
Example:
@Deployment
public static WebArchive createDeployment() {
return ShrinkWrap.create(WebArchive.class)
.addAsResource("test-persistence.xml", "META-INF/persistence.xml")
.addAsManifestResource("test-ds.xml", "resources/test-ds.xml");
}
In this example, the addAsManifestResource
method is used to include a custom datasource configuration file in the deployment package, ensuring that the required datasource is available during test execution.
In Conclusion, Here is What Matters
By addressing the common issues discussed in this article, you can streamline your experience with Arquillian 1.0.0.Final and ensure that your tests run smoothly and effectively. Remember to always refer to the official Arquillian documentation and community resources for further insights and solutions to any issues you may encounter. Happy testing with Arquillian!
Checkout our other articles