Maximize Efficiency with Native CDI Qualifiers
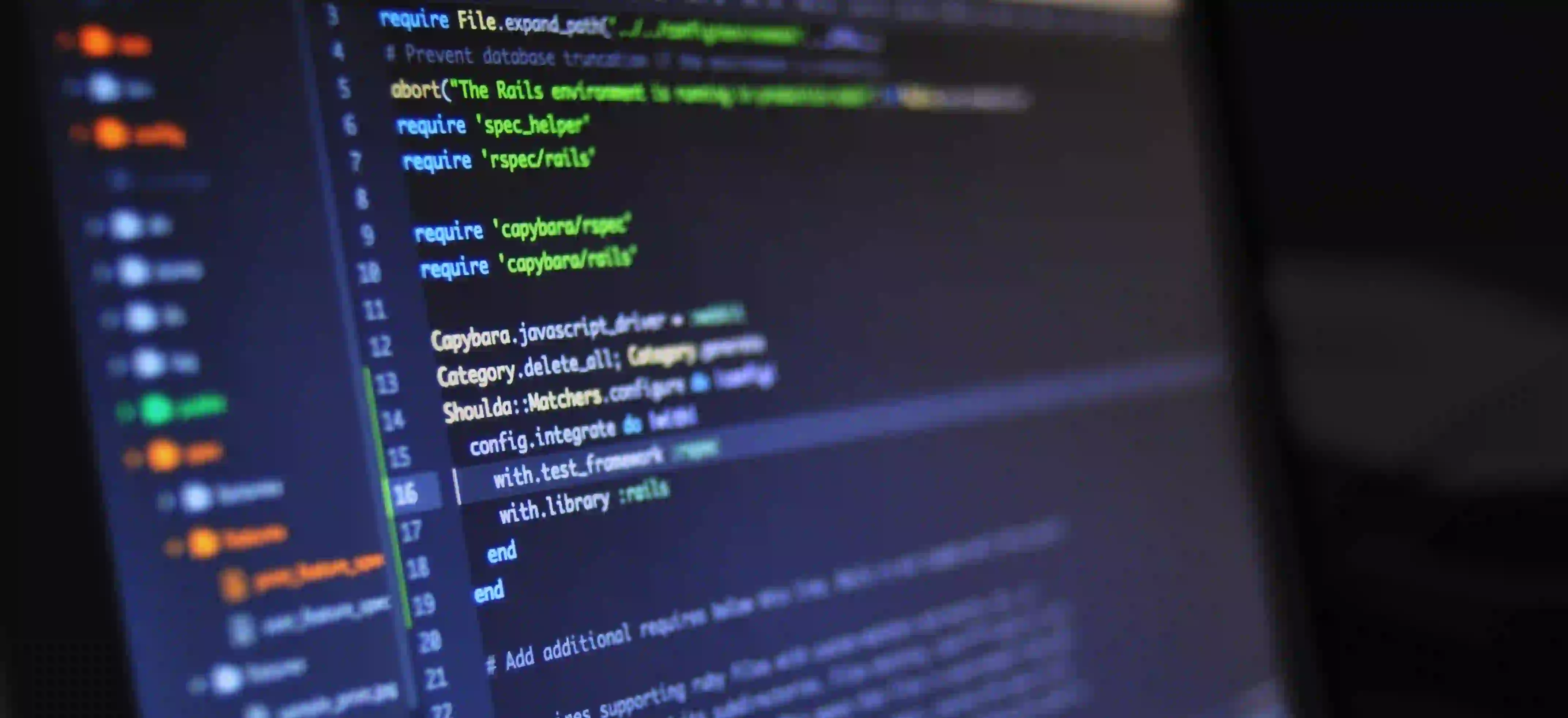
Maximize Efficiency with Native CDI Qualifiers
Java's Contexts and Dependency Injection (CDI) is a powerful framework that facilitates the development of loosely coupled systems with the help of Dependency Injection. One of the many powerful features of CDI is the use of qualifiers to effectively differentiate between beans of the same type. While CDI provides the @Qualifier
meta-annotation to create custom qualifiers, Java SE 8 introduced the concept of native qualifiers, allowing for a more streamlined and efficient approach.
In this article, we will explore the benefits of native CDI qualifiers and demonstrate how they can be used to maximize efficiency in your Java applications.
Understanding Native Qualifiers
Native CDI qualifiers are annotations that can be used as qualifiers without the need for creating a separate @Qualifier
annotation. This feature was introduced in CDI 2.0 as part of the Java EE 8 platform.
Using native qualifiers eliminates the need to create separate qualifier annotations for simple use cases, reducing boilerplate code and improving code readability. This results in a more streamlined and efficient development process.
Implementation of Native Qualifiers
Let's take a look at how native qualifiers can be implemented and their benefits. Consider the following example where we have a NotificationService
interface with two different implementations: EmailNotificationService
and SMSService
.
public interface NotificationService {
void sendNotification(String message);
}
@Email
public class EmailNotificationService implements NotificationService {
public void sendNotification(String message) {
// Implementation for sending email notification
}
}
@SMS
public class SMSService implements NotificationService {
public void sendNotification(String message) {
// Implementation for sending SMS notification
}
}
In the above code, @Email
and @SMS
are native qualifiers, and they can be used directly to specify the bean to be injected, without needing to create separate qualifier annotations.
Benefits of Native Qualifiers
Improved Readability and Maintainability
By using native qualifiers, the code becomes more concise and easier to read. It eliminates the need for creating and managing separate qualifier annotations, thereby reducing boilerplate code and improving code maintainability. Native qualifiers allow developers to focus on the actual business logic rather than getting bogged down by infrastructure code.
Reduced Development Time
Native qualifiers enable faster development by eliminating the need to write additional qualifier annotations. This leads to reduced development time and allows developers to focus on implementing core functionalities. The streamlined approach offered by native qualifiers can significantly increase overall development efficiency.
Seamless Integration with Existing Code
Native qualifiers seamlessly integrate with existing CDI features and can be used alongside custom qualifiers. This ensures compatibility and smooth migration for projects that already utilize custom qualifiers. The flexibility of native qualifiers allows developers to adopt them gradually, without disrupting the current codebase.
Closing Remarks
In conclusion, native CDI qualifiers offer a more efficient and streamlined approach to bean qualification in Java applications. By eliminating the need for separate qualifier annotations, native qualifiers improve code readability, reduce development time, and seamlessly integrate with existing code. This makes them a valuable addition to the CDI framework, allowing developers to maximize efficiency and productivity in their projects.
To delve deeper into CDI and its native qualifiers, you can refer to the official Java EE 8 documentation. Additionally, exploring real-world use cases and examples will provide further insights into leveraging native qualifiers within your applications.
Integrating native qualifiers into your CDI-based projects can lead to cleaner, more maintainable, and efficient code, ultimately enhancing the overall development experience.
Make the most of native CDI qualifiers and elevate the quality of your Java applications!