Optimizing Java Applications for Docker Deployment
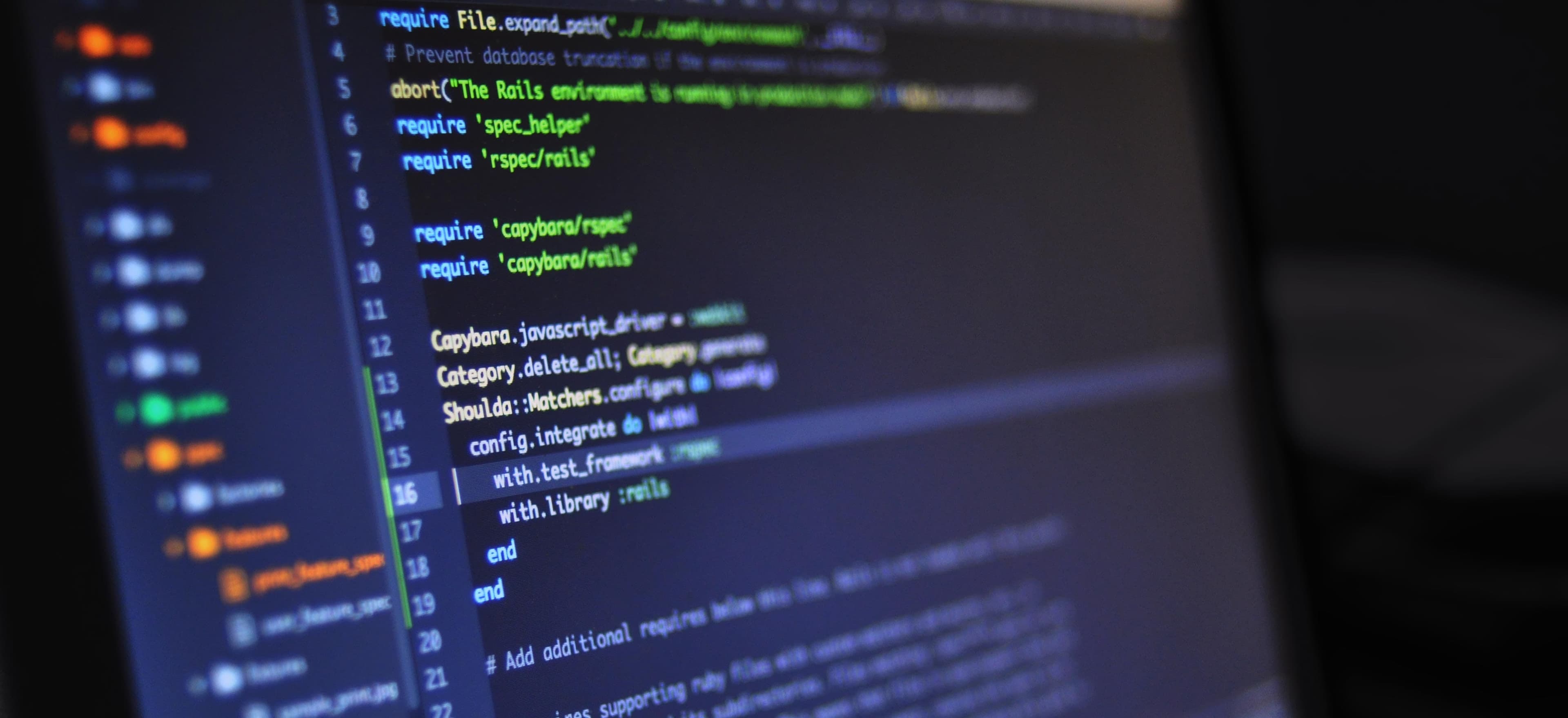
- Published on
Optimizing Java Applications for Docker Deployment
In recent years, Docker has emerged as a popular tool for packaging, shipping, and running applications within containers. Containers offer a consistent environment across development, testing, and production, making them an ideal choice for deploying Java applications. However, optimizing Java applications for Docker deployment involves addressing various aspects, including image size, startup time, and resource utilization. In this article, we'll explore best practices for optimizing Java applications to run efficiently in Docker containers.
Choosing the Right Base Image
When optimizing Java applications for Docker, the choice of base image plays a crucial role. It's essential to select a base image that is specifically designed for running Java applications. For example, using an Alpine Linux-based image with OpenJDK can significantly reduce the overall image size compared to using a standard Linux distribution. This reduction in image size can lead to faster image pull times and reduced disk space usage.
Example:
FROM openjdk:11-jre-slim
In this example, we use the openjdk:11-jre-slim
base image, which provides a minimal runtime environment for Java 11 applications. The slim variant helps in reducing the size of the Docker image, making it more lightweight and efficient.
Minimizing Image Layers
When building Docker images for Java applications, minimizing the number of layers can contribute to improved build and deployment times. Each instruction in a Dockerfile results in the creation of a new layer. Therefore, it's beneficial to combine related commands to reduce the overall number of layers.
Example:
FROM openjdk:11-jre-slim
WORKDIR /app
COPY target/my-application.jar /app
CMD ["java", "-jar", "my-application.jar"]
In this example, the WORKDIR
, COPY
, and CMD
instructions are combined to create a single layer, thereby reducing the number of layers in the Docker image.
Memory Considerations
Java applications often have specific memory requirements, and it's essential to configure the JVM memory settings appropriately when running them in Docker containers. Failure to allocate an optimal amount of memory can lead to performance issues and potential out-of-memory errors.
Example:
FROM openjdk:11-jre-slim
WORKDIR /app
COPY target/my-application.jar /app
CMD ["java", "-Xmx512m", "-jar", "my-application.jar"]
In this example, the -Xmx512m
flag sets the maximum heap size for the JVM to 512 MB, ensuring that the Java application running in the Docker container has sufficient memory allocated.
Use of Build Caching
Utilizing build caching can significantly speed up the Docker build process for Java applications. By leveraging build caching, Docker can reuse previously built layers from the cache, thus reducing the need to rebuild unchanged dependencies and layers.
Example:
FROM openjdk:11-jre-slim
# Install maven dependencies
COPY pom.xml /tmp/
RUN mvn -f /tmp/pom.xml dependency:go-offline
# Build application
COPY . /tmp/
RUN mvn -f /tmp/pom.xml package
WORKDIR /app
RUN cp /tmp/target/my-application.jar /app
CMD ["java", "-jar", "my-application.jar"]
In this example, the COPY pom.xml /tmp/
and RUN mvn -f /tmp/pom.xml dependency:go-offline
instructions install Maven dependencies, and due to build caching, these layers will be reused if the pom.xml
file remains unchanged.
Monitoring and Performance Tuning
Monitoring the performance of Java applications running in Docker containers is essential for identifying potential bottlenecks and optimizing resource utilization. Tools like Prometheus and Grafana can be used to collect and visualize metrics, providing insights into CPU usage, memory allocation, and other performance indicators.
Example:
version: '3.8'
services:
my-application:
image: my-application:latest
ports:
- "8080:8080"
environment:
- JAVA_OPTS=-Xmx512m
deploy:
resources:
limits:
cpus: '0.5'
memory: 512M
reservations:
cpus: '0.25'
memory: 256M
In this example, a Docker Compose file is used to define resource limits and reservations for the Java application container, ensuring that it operates within specific constraints.
The Bottom Line
Optimizing Java applications for Docker deployment involves a combination of best practices, including choosing the right base image, minimizing image layers, configuring memory settings, leveraging build caching, and monitoring performance. By implementing these optimizations, Java applications can run efficiently and reliably within Docker containers, contributing to a streamlined development and deployment process.
In conclusion, optimizing Java applications for Docker deployment is essential for achieving efficient resource utilization, minimizing image size, and ensuring optimal performance within containerized environments.
By following these best practices, developers can create Dockerized Java applications that are well-suited for modern, cloud-native architectures, and seamlessly integrate into container orchestration platforms.
For further exploration, be sure to check out useful resources like Docker Hub for official Docker images and the Docker documentation for in-depth guidance on optimizing Java applications for Docker deployment. Happy coding!
Checkout our other articles