Maximizing Code Coverage for Continuous Delivery
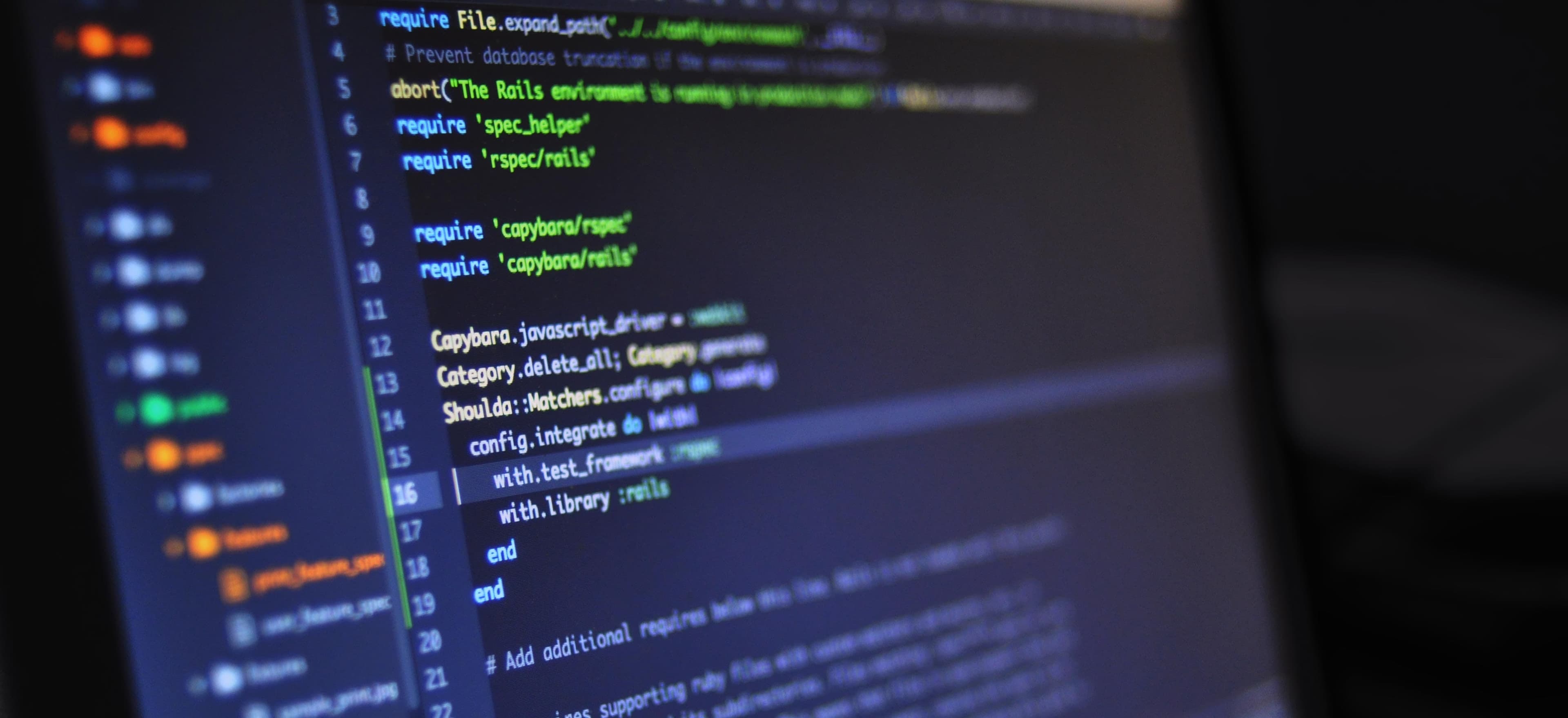
- Published on
Maximizing Code Coverage for Continuous Delivery
In today's fast-paced software development world, continuous delivery is crucial for staying competitive and meeting customer demands. However, to ensure the quality of our software, we need a robust set of automated tests. One essential metric for assessing test coverage is code coverage, which measures the percentage of our codebase exercised by our tests. In this blog post, we'll explore how to maximize code coverage in Java for continuous delivery.
What is Code Coverage?
Code coverage is a metric that determines the percentage of code that is executed by automated tests. It helps us identify areas of our codebase that lack proper testing and ensures that our tests are thorough. There are several types of code coverage—line coverage, branch coverage, and path coverage, each providing a different level of granularity when analyzing our code.
Importance of Code Coverage for Continuous Delivery
High code coverage is crucial for successful continuous delivery. It provides confidence that our code changes are unlikely to introduce new bugs, as the affected code is well-tested. Additionally, high code coverage simplifies debugging, as uncovered code paths are more likely to contain bugs. By aiming for high code coverage, we can ensure that our continuous delivery pipeline delivers high-quality software at a rapid pace.
Utilizing JUnit for Unit Testing
In Java, JUnit is a popular framework for writing and executing unit tests. Let's look at an example of a simple JUnit test case and analyze how it contributes to code coverage.
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 4);
assertEquals(7, result);
}
}
In this example, we have a test case for a Calculator
class that tests the add
method. When we run our tests, our code coverage tool will track the lines of code executed during the test. By aiming to test every code path, we can achieve higher code coverage.
Using Mockito for Mocking Dependencies
Often in Java development, our classes have dependencies on external services, databases, or other complex systems. Mocking these dependencies during testing is essential to ensure that our tests focus solely on the unit under test. Mockito is a popular framework for creating mock objects in Java. Let's see how it aids in achieving comprehensive code coverage.
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testUserCreation() {
UserRepository userRepository = mock(UserRepository.class);
UserService userService = new UserService(userRepository);
User user = new User("John Doe");
userService.createUser(user);
verify(userRepository, times(1)).saveUser(user);
}
}
In this example, we use Mockito to create a mock UserRepository
and verify that the saveUser
method is invoked when the createUser
method is called. By mocking the UserRepository
, we isolate the testing of the UserService
and ensure that our tests cover its behavior exclusively.
Leveraging Integration Testing with Spring Boot
While unit tests are essential, integration tests play a significant role in achieving high code coverage, especially in the context of continuous delivery. Spring Boot provides a powerful framework for writing integration tests that interact with the application in a more comprehensive manner.
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.context.SpringBootTest.WebEnvironment;
import org.springframework.boot.test.web.client.TestRestTemplate;
import org.springframework.boot.web.server.LocalServerPort;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.web.servlet.MockMvc;
@SpringBootTest(webEnvironment = WebEnvironment.RANDOM_PORT)
@AutoConfigureMockMvc
public class UserControllerIntegrationTest {
@LocalServerPort
private int port;
@Autowired
private TestRestTemplate restTemplate;
@Autowired
private MockMvc mockMvc;
@Test
public void testGetUserById() {
// Perform a REST API request and validate the response
}
}
In this example, we use a combination of Spring Boot's testing annotations, TestRestTemplate
, and MockMvc
to write an integration test for a web endpoint. Integration tests ensure that the various components of our application work together as expected, ultimately contributing to higher code coverage and confidence in our continuous delivery pipeline.
Achieving High Code Coverage with JaCoCo
To measure code coverage in our Java projects, we can use JaCoCo, a popular code coverage library. It provides detailed reports on code coverage, highlighting which areas of our codebase are lacking test coverage.
Integrating JaCoCo with Maven
To integrate JaCoCo with Maven, we need to include the jacoco-maven-plugin
in our project's pom.xml
file. This plugin will generate code coverage reports during the build process.
<project>
...
<build>
<plugins>
...
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.6</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<phase>test</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
...
</plugins>
</build>
...
</project>
In this configuration, the prepare-agent
goal instruments our code during the build, while the report
goal generates the code coverage report during the test phase.
Viewing Code Coverage Reports
After running our tests with JaCoCo enabled, we can view the code coverage reports in the target/site/jacoco/index.html
file. These reports provide a detailed overview of our codebase, highlighting which lines and branches are covered by our tests.
By integrating JaCoCo into our build process, we can continuously monitor and improve our code coverage, ensuring that our codebase is well-tested and ready for continuous delivery.
The Bottom Line
Maximizing code coverage in Java is essential for ensuring the quality and reliability of our software during continuous delivery. By utilizing tools and frameworks such as JUnit, Mockito, Spring Boot, and JaCoCo, we can create a comprehensive suite of tests that exercise our codebase thoroughly.
High code coverage not only improves the robustness of our code but also boosts our confidence in deploying changes rapidly. By embracing a test-driven mindset and leveraging the appropriate tools, we can build a continuous delivery pipeline that delivers high-quality software consistently.
In conclusion, achieving high code coverage in Java is not just a metric—it is a crucial step towards building reliable, maintainable, and successful software products.
Stay tuned for more insightful Java development content!