Managing Concurrency in Spring State Machine
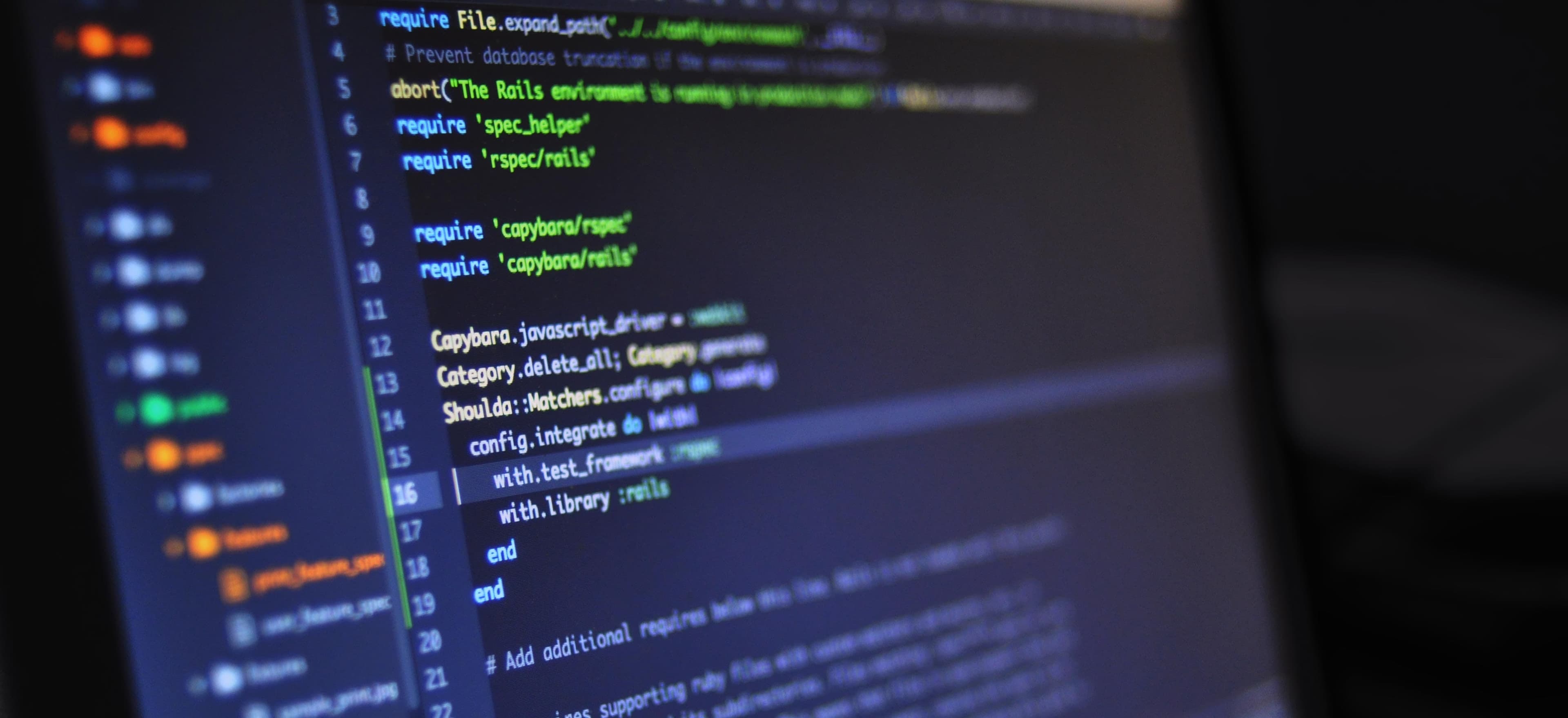
- Published on
Essentials at a Glance
Concurrency in software development is the ability of a system to handle multiple tasks at the same time. In the context of Java and Spring applications, managing concurrency is crucial for ensuring that the system can efficiently execute tasks in parallel without compromising data consistency or causing conflicts. In this blog post, we will explore how to manage concurrency in Spring State Machine, a powerful framework for implementing state machine-based behavior in your applications.
Understanding Spring State Machine
Spring State Machine is a framework that provides state machine based programming model for Spring framework. It allows you to define state machines and configure transitions between states. State machines are useful for modeling complex logic and defining workflows within an application.
Concurrency Challenges in State Machine
When dealing with state machines in a concurrent environment, several challenges may arise. These challenges include:
- Ensuring thread safety when transitioning between states
- Managing shared resources accessed by multiple state machine instances
- Coordinating state machine events and actions in a multi-threaded environment
Managing Concurrency with Spring State Machine
Let's explore some techniques for managing concurrency in Spring State Machine.
1. Synchronized Transitions
One way to manage concurrency in Spring State Machine is to use synchronization to ensure that only one thread can transition the state machine at a time. This can be achieved by adding synchronization to the transition methods of the state machine.
@Configuration
@EnableStateMachine
public class StateMachineConfig extends StateMachineConfigurerAdapter<String, String> {
@Override
public void configure(StateMachineConfigurationConfigurer<String, String> config) throws Exception {
config
.withConfiguration()
.machineConfiguration()
.listener(new StateMachineListenerAdapter<String, String>() {
@Override
public void transition(Transition<String, String> transition) {
synchronized (this) {
// Perform transition logic
}
}
});
}
}
In the above code, we use the synchronized
keyword to ensure that the transition
method is executed by only one thread at a time, thus preventing concurrent transitions that may lead to unintended behavior or data corruption.
2. Using Thread-safe Actions and Guards
Another approach to manage concurrency in Spring State Machine is to make sure that the actions and guards associated with state machine transitions are thread-safe. This means that the code within these actions and guards should be designed to work correctly when called from multiple threads concurrently.
@Configuration
@EnableStateMachine
public class StateMachineConfig extends StateMachineConfigurerAdapter<String, String> {
@Override
public void configure(StateMachineTransitionConfigurer<String, String> transitions) throws Exception {
transitions
.withExternal()
.source("STATE1")
.target("STATE2")
.event("EVENT1")
.action((stateContext) -> {
// Ensure thread-safe action logic
})
.guard((stateContext) -> {
// Ensure thread-safe guard logic
return true;
});
}
}
Here, the action
and guard
methods are designed to execute thread-safe logic, ensuring that the state machine transitions are handled correctly even in a concurrent environment.
3. Using Asynchronous Executors
Spring provides support for asynchronous task execution through the @Async
annotation and TaskExecutor
interface. By leveraging asynchronous execution, you can offload state machine tasks to separate threads, thus improving concurrency and responsiveness of the application.
@Component
public class StateMachineService {
@Async
public void processStateMachineEvent() {
// Handle state machine event asynchronously
}
}
In the above example, the processStateMachineEvent
method is annotated with @Async
to indicate that it should be executed asynchronously. Under the hood, Spring will manage the concurrency by using a separate thread pool to process these asynchronous state machine events.
4. Resource Isolation and Locking
In scenarios where multiple state machine instances need to access shared resources, it's essential to implement proper resource isolation and locking mechanisms. This can be achieved using tools such as Java's Lock
interface or higher-level constructs like ReentrantLock
or ReadWriteLock
to control access to shared resources.
@Component
public class SharedResourceService {
private ReentrantLock lock = new ReentrantLock();
public void accessSharedResource() {
lock.lock();
try {
// Access shared resource in a thread-safe manner
} finally {
lock.unlock();
}
}
}
In the code snippet above, the ReentrantLock
is used to ensure that only one state machine instance can access the shared resource at a time, thus preventing concurrent access issues.
Lessons Learned
In this blog post, we've explored techniques for managing concurrency in Spring State Machine. By ensuring thread safety, leveraging asynchronous execution, and implementing proper resource isolation, you can effectively handle concurrent state machine transitions and actions within your applications. Managing concurrency in Spring State Machine is crucial for building robust and scalable applications that can efficiently handle multiple tasks in parallel.