Implementing Scalable Event-Driven Architecture
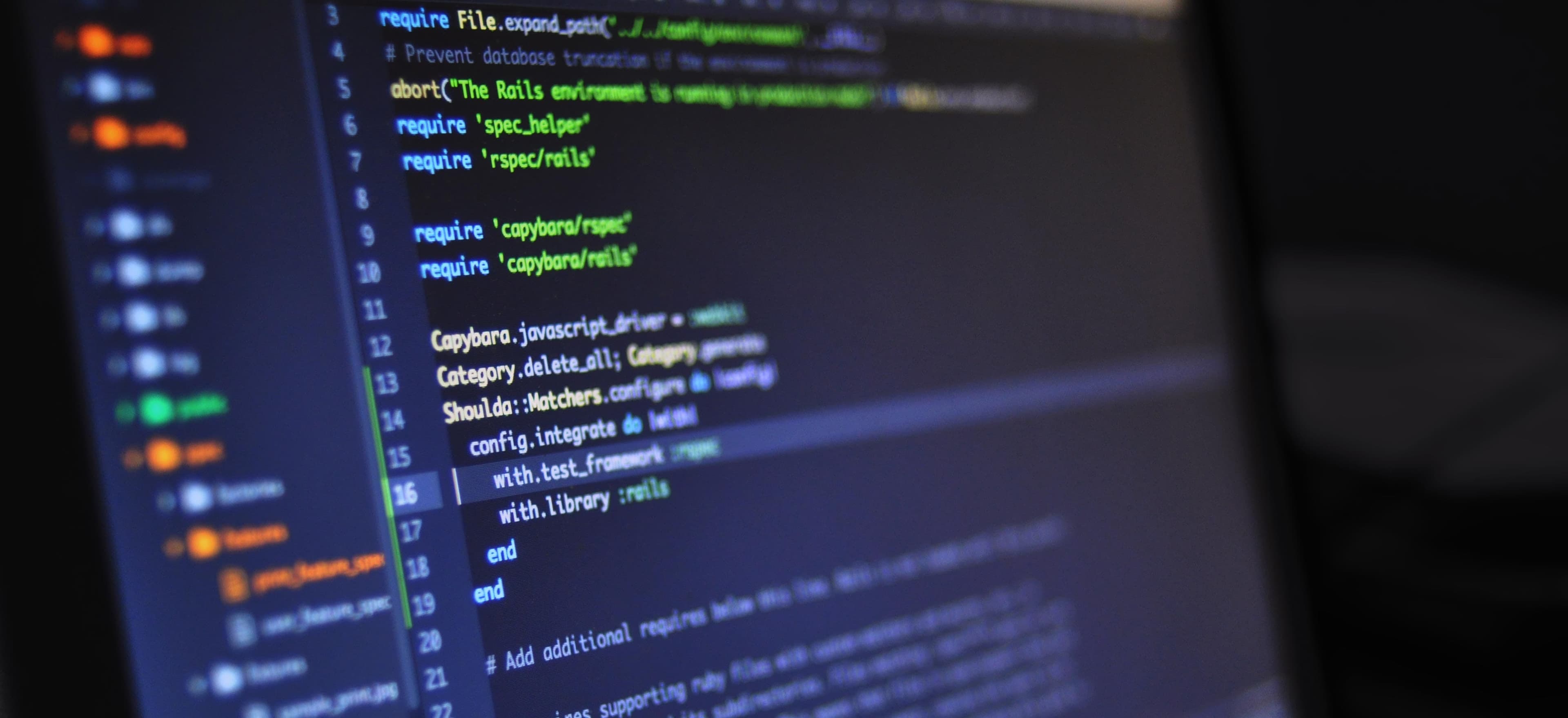
- Published on
Building Scalable Event-Driven Architecture in Java
In today's world of distributed systems, building scalable, resilient, and responsive applications is crucial. Event-driven architecture (EDA) has gained popularity due to its ability to handle asynchronous processing, decouple components, and scale effectively. Java, as a robust and versatile programming language, provides powerful tools and libraries to implement EDA. In this article, we will explore the key principles of building a scalable event-driven architecture in Java and demonstrate how to implement it effectively.
Understanding Event-Driven Architecture
Event-driven architecture is a design pattern that promotes the production, detection, consumption, and reaction to events. Events are immutable messages that represent a change in state or occurrence within a system. In an event-driven system, components communicate through events, enabling loose coupling and flexible integration.
Key Components of Event-Driven Architecture
- Event Producers: Entities that generate events based on certain triggers or changes in state.
- Event Channel: Mechanism for propagating events to interested consumers.
- Event Consumers: Components that subscribe to and process events of interest.
Choosing the Right Framework for Event-Driven Architecture
When building event-driven systems in Java, it's essential to select a well-suited framework. Spring Cloud Stream and Apache Kafka are popular choices for implementing event-driven architecture.
Spring Cloud Stream
Spring Cloud Stream provides a framework for building highly scalable event-driven microservices connected with shared messaging systems. It offers seamless integration with message brokers such as Apache Kafka, RabbitMQ, and others.
Apache Kafka
Apache Kafka, a distributed streaming platform, excels in handling high throughput and fault-tolerant event streaming. Its distributed nature and fault tolerance make it a go-to choice for building robust event-driven systems.
Implementing Event Producers in Java
Let's start by creating an event producer using Spring Boot and Spring Cloud Stream with Kafka binder. In the following example, we'll produce a 'UserCreatedEvent' whenever a new user is registered in the system.
@SpringBootApplication
@EnableBinding(UserCreatedSource.class)
public class UserCreatedEventProducer {
@Autowired
private UserCreatedSource userCreatedSource;
public void publishUserCreatedEvent(User user) {
UserCreatedEvent event = new UserCreatedEvent(user.getId(), user.getName());
userCreatedSource.output().send(MessageBuilder.withPayload(event).build());
}
}
interface UserCreatedSource {
@Output("userCreatedOut")
MessageChannel output();
}
public class UserCreatedEvent {
private String userId;
private String userName;
// Constructor and getters
}
In this example, UserCreatedEventProducer
is a Spring Boot application that publishes UserCreatedEvent
to the Kafka topic bound to the userCreatedOut
output channel.
Why Spring Cloud Stream with Kafka?
Spring Cloud Stream simplifies the integration with message brokers while providing a high-level abstraction for building event-driven systems. By leveraging Kafka binder, it ensures seamless connectivity and scalability, making it an ideal choice for event producers.
Subscribing to Events as Event Consumers
Now, let's implement an event consumer that listens to the 'UserCreatedEvent' and performs certain actions, such as sending a welcome email.
@Service
@EnableBinding(UserCreatedSink.class)
public class UserCreatedEventConsumer {
@StreamListener("userCreatedIn")
public void handleUserCreatedEvent(UserCreatedEvent event) {
// Send welcome email to the newly registered user
emailService.sendWelcomeEmail(event.getUserId(), event.getUserName());
}
}
interface UserCreatedSink {
@Input("userCreatedIn")
SubscribableChannel input();
}
public class EmailService {
public void sendWelcomeEmail(String userId, String userName) {
// Implementation of sending welcome email
}
}
In this consumer implementation, UserCreatedEventConsumer
listens to the 'userCreatedIn' channel and triggers the sendWelcomeEmail
method of EmailService
upon receiving a UserCreatedEvent
.
Why Use Spring Cloud Stream for Event Consumers?
Spring Cloud Stream simplifies the process of binding application code to message brokers, abstracting the complexity of dealing with low-level APIs. By using Spring Cloud Stream, the consumer can focus on handling the business logic associated with the received events, resulting in a clean and maintainable codebase.
Ensuring Scalability in Event-Driven Architectures
Scalability is a critical aspect of event-driven systems, especially when dealing with high event volumes. Let's discuss a few strategies to ensure scalability in Java-based event-driven architectures.
1. Partitioning and Parallelism
In Kafka, partitioning allows for distributing data across multiple nodes, enabling parallel processing and scalability. When designing event-driven systems with Kafka, careful consideration of partitioning strategies is crucial to achieve optimal scalability.
2. Load Balancing
Utilize load balancing techniques to evenly distribute event processing across consumer instances. This ensures efficient resource utilization and prevents overload on individual consumers.
3. Consumer Group Management
In Kafka, consumer groups enable parallel consumption of events, providing fault tolerance and scalability. Proper management and scaling of consumer groups are essential for handling varying workloads efficiently.
Monitoring and Observability
Monitoring event-driven architectures is essential for identifying bottlenecks, ensuring system health, and making informed scaling decisions. Tools like Prometheus combined with Grafana can provide comprehensive insights into the performance and behavior of event-driven systems, enabling proactive scaling and optimization.
Closing Remarks
Implementing scalable event-driven architecture in Java requires a well-thought-out design and careful consideration of the tools and frameworks. By leveraging the power of Spring Cloud Stream, Apache Kafka, and proven scalability strategies, developers can build resilient, responsive, and scalable event-driven systems. Embracing event-driven architecture empowers applications to handle modern challenges, including real-time data processing, decoupling of microservices, and seamless scalability.
In conclusion, Java, coupled with robust event-driven architecture principles, paves the way for building the next generation of scalable and resilient distributed systems. Embrace the power of events, decouple your components, and scale with confidence using Java's event-driven capabilities.
By adopting these approaches, Java developers can unlock the potential for scalable, resilient, and responsive applications through event-driven architecture.