Handling Asynchronous Responses in JMeter Stress Testing
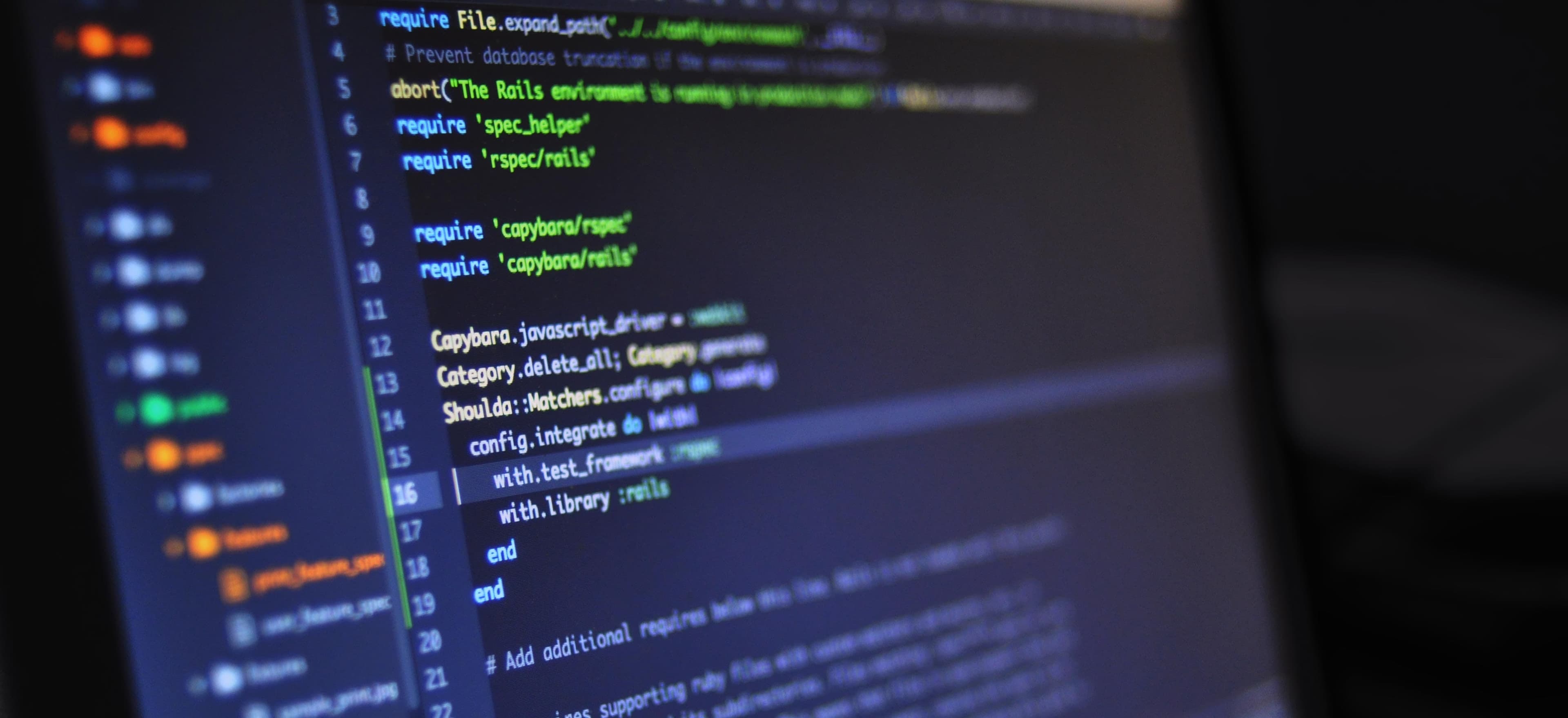
- Published on
How to Handle Asynchronous Responses in JMeter Stress Testing
When conducting stress testing in JMeter, it's crucial to consider how the application handles asynchronous responses. Asynchronous responses are a common occurrence in web applications, especially in scenarios involving AJAX calls, web sockets, or server push technologies.
In this blog post, we'll explore the challenges of dealing with asynchronous responses in JMeter stress testing and discuss strategies for effectively handling them.
Understanding Asynchronous Responses
Before delving into the specifics of handling asynchronous responses in JMeter, let's briefly understand what asynchronous responses are and why they are important in stress testing.
Asynchronous responses occur when the server responds to a client request at a later time, often independently of the original request. This can introduce complexities in stress testing scenarios, as the timing and sequencing of responses become unpredictable.
In stress testing, it's essential to accurately capture and validate asynchronous responses to ensure the stability and reliability of the tested application under heavy load.
Challenges of Dealing with Asynchronous Responses in JMeter
When using JMeter for stress testing, dealing with asynchronous responses presents several challenges:
- Timing Dependencies: Asynchronous responses may arrive at unpredictable intervals, leading to timing dependencies that are hard to simulate in a traditional JMeter test plan.
- Correlating Requests and Responses: Matching asynchronous responses to the corresponding requests can be complex, especially when multiple concurrent requests are involved.
- Response Validation: Validating asynchronous responses, which may not necessarily be directly linked to the original requests, requires careful handling to ensure accuracy.
Strategies for Handling Asynchronous Responses in JMeter
1. Using Timers
JMeter provides various timer elements that can be used to introduce delays between requests. While this may not directly address asynchronous responses, strategically placed timers can help simulate realistic timing scenarios and account for delayed responses.
// Example of a constant timer in JMeter
public class ConstantTimerExample {
public static void main(String[] args) {
ConstantTimer constantTimer = new ConstantTimer(2000); // 2000 milliseconds delay
constantTimer.run();
}
}
In this code snippet, the ConstantTimer
is used to introduce a 2000 milliseconds delay between consecutive requests in a JMeter test plan.
2. Post-Processor for Response Correlation
Utilizing JMeter's Post-Processor elements such as Regular Expression Extractor or JSON Extractor can help in correlating asynchronous responses with the corresponding requests. These post-processors extract data from the server responses, which can then be used in subsequent requests or for validation purposes.
// Example of using Regular Expression Extractor in JMeter
Regular_Expression_Extractor extractor = new Regular_Expression_Extractor();
String response = "Sample response body";
String regex = "pattern_to_extract_data";
String matchedValue = extractor.extract(response, regex);
In this example, the Regular Expression Extractor is used to extract a specific value from the response body using a regular expression pattern, which can be further utilized in subsequent requests or for validation.
3. Response Assertion
JMeter's Response Assertion can be employed to validate the content or the structure of asynchronous responses. By defining assertion rules based on the expected response content, status codes, or patterns, you can ensure that the asynchronous responses meet the required criteria.
// Example of using Response Assertion in JMeter
Response_Assertion assertion = new Response_Assertion();
String response = "Sample response body";
assertion.checkResponse(response); // Validate the response against defined assertion rules
Key Takeaways
In conclusion, effectively handling asynchronous responses is critical for conducting comprehensive stress testing in JMeter. By understanding the challenges and employing the strategies discussed in this post, testers can ensure that their stress tests accurately simulate real-world scenarios, ultimately leading to more reliable and insightful results.
Mastering the art of handling asynchronous responses in JMeter is not only a valuable skill but also a significant factor in achieving meaningful stress testing outcomes.
For further in-depth understanding, you can refer to the JMeter User Manual and JMeter Best Practices for stress testing.
Stay tuned for more insightful discussions on JMeter and stress testing best practices!