Deploying Spring Boot on Wildfly 8.2: Common Issues
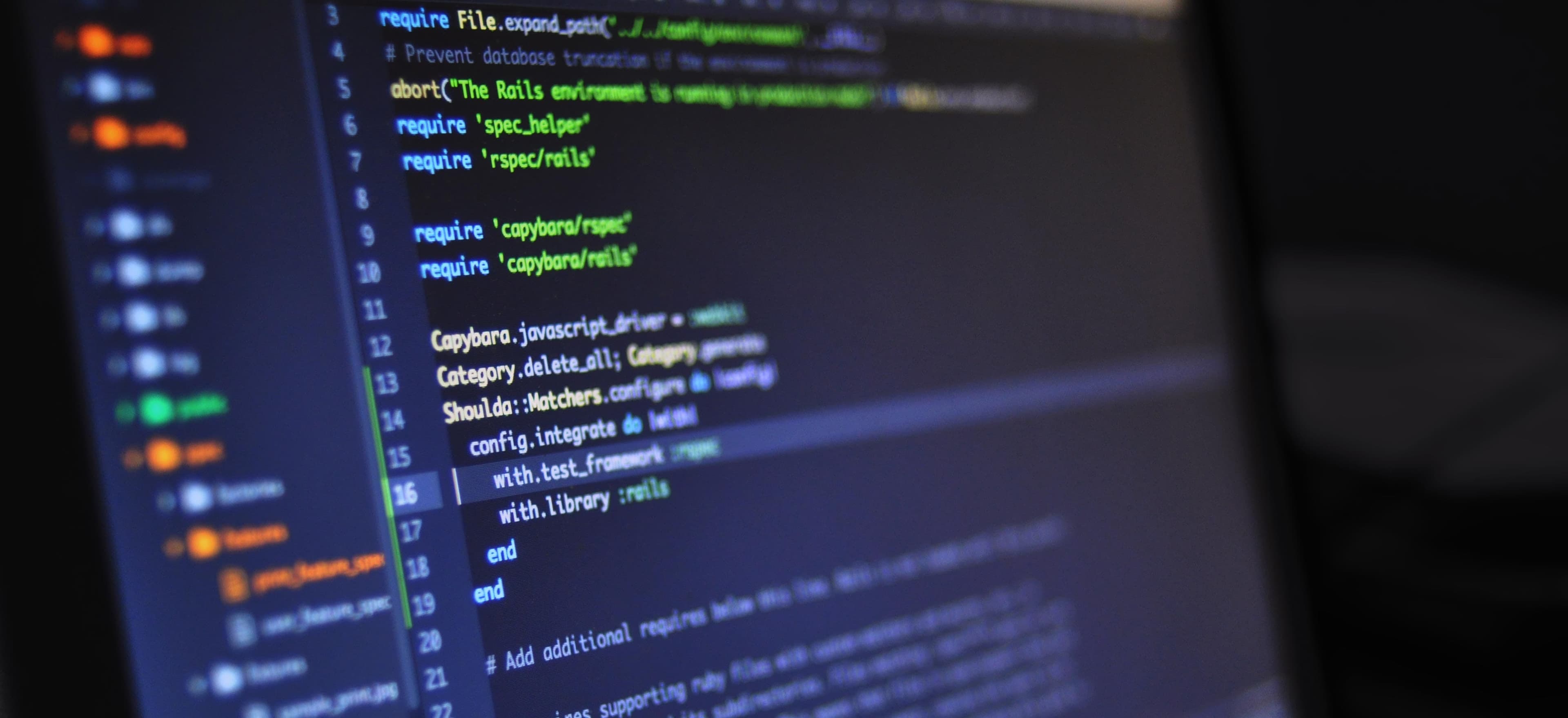
- Published on
Common Issues when Deploying Spring Boot on Wildfly 8.2
When deploying a Spring Boot application on Wildfly 8.2, several common issues may arise that can hinder successful deployment and operation. In this blog post, we will delve into some of these issues and provide solutions to help you overcome them.
Issue 1: ClassNotFound Exception for Spring Classes
One common issue encountered when deploying a Spring Boot application on Wildfly 8.2 is a java.lang.ClassNotFoundException
for Spring classes. This occurs because the necessary Spring libraries are not included in the deployment package.
To resolve this issue, you can manually include the required Spring libraries in your Spring Boot application's WAR file. The easiest way to achieve this is by using the Maven war plugin to build an executable WAR file with all dependencies included.
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
By using the spring-boot-maven-plugin
with the repackage
goal, all dependencies, including the Spring libraries, will be bundled into the WAR file, ensuring they are available during runtime on Wildfly.
Issue 2: Incompatible JAR Files
Another common issue that may arise when deploying a Spring Boot application on Wildfly 8.2 is the presence of incompatible JAR files. This can lead to classloading issues and unexpected runtime errors.
To address this issue, it is essential to ensure that the versions of JAR files included in your Spring Boot application are compatible with the version of Wildfly 8.2. You can use Maven dependency:tree to analyze the dependencies and their versions, and exclude any conflicting or redundant dependencies.
mvn dependency:tree
By identifying and excluding conflicting dependencies, you can prevent classloading conflicts and ensure that your Spring Boot application operates smoothly on Wildfly 8.2.
Issue 3: Incorrect Datasource Configuration
Datasource configuration is crucial for the proper functioning of a Spring Boot application, especially when deploying on a Java EE container like Wildfly 8.2. Incorrect datasource configuration can lead to connection errors and database access issues.
When deploying on Wildfly 8.2, it is recommended to define the datasource in the application server's configuration and access it via JNDI lookup within the Spring Boot application.
Here is an example of datasource configuration in Wildfly's standalone.xml:
<datasource jta="true" jndi-name="java:jboss/datasources/MyDS" pool-name="MyDS" enabled="true" use-ccm="true">
<connection-url>jdbc:mysql://localhost:3306/mydb</connection-url>
<driver-class>com.mysql.jdbc.Driver</driver-class>
<driver>mysql</driver>
<security>
<user-name>username</user-name>
<password>password</password>
</security>
</datasource>
Ensure that the JNDI name specified in the datasource configuration matches the JNDI name used for datasource lookup in your Spring Boot application's application.properties
or application.yml
.
spring:
datasource:
jndi-name: java:jboss/datasources/MyDS
By aligning the JNDI names and datasource configurations, you can establish a successful connection to the database when deploying your Spring Boot application on Wildfly 8.2.
Issue 4: Security Constraints and Configuration
When deploying a Spring Boot application on Wildfly 8.2, you may encounter issues related to security constraints and configuration. Wildfly 8.2 has its own security subsystem, and it is important to ensure that the security constraints defined in the application do not conflict with the security settings of the application server.
To avoid security conflicts, configure the security settings in Wildfly's web.xml
or jboss-web.xml
rather than in the Spring Boot application's configuration. This ensures that the security constraints are enforced by the application server and do not interfere with the application's functionality.
Bringing It All Together
Deploying a Spring Boot application on Wildfly 8.2 can be a challenging task, but by addressing the common issues discussed in this blog post, you can ensure a smooth and successful deployment. By including the necessary Spring libraries, managing dependencies, configuring the datasource correctly, and addressing security concerns, you can overcome the obstacles and leverage the capabilities of both Spring Boot and Wildfly 8.2.
If you encounter any additional issues or have further questions, feel free to explore the Wildfly official documentation and the Spring Boot reference guide. Happy coding!