Improving Test Environment Stability with Docker
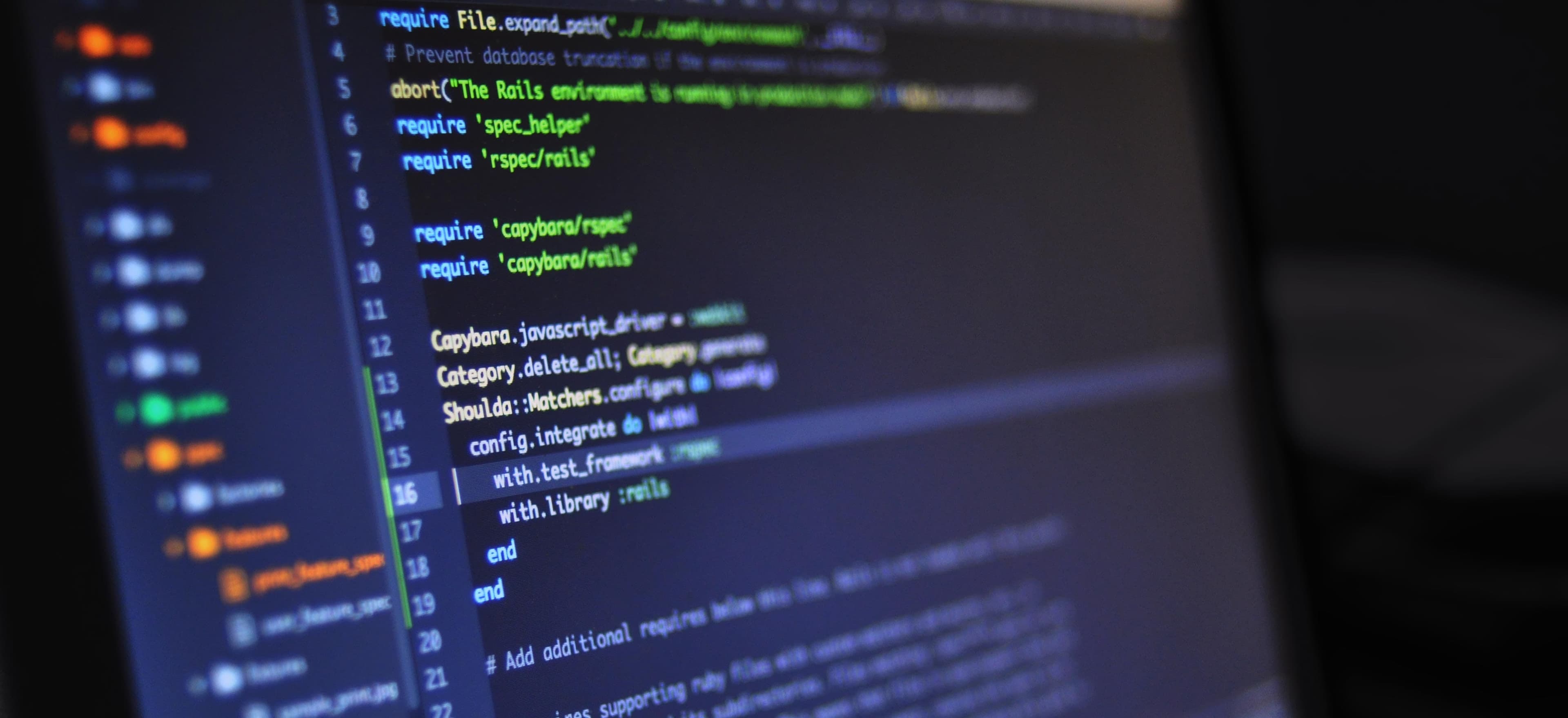
- Published on
Improving Test Environment Stability with Docker
In software development, creating a stable and reliable test environment is crucial for ensuring the quality of the code. One of the challenges developers face is the inconsistency between development, testing, and production environments. Docker is a powerful tool that can help address this challenge by providing a consistent environment across different stages of the development lifecycle. In this blog post, we will explore how Docker can improve test environment stability and discuss best practices for implementing Docker in the testing process.
Understanding the Problem
Traditional test environments often suffer from inconsistencies due to differences in operating systems, library versions, and configurations. Developers may encounter the infamous "it works on my machine" issue where code runs perfectly on a developer's local environment but fails in testing or production. These discrepancies can lead to bugs and errors that are hard to reproduce and fix.
Introducing Docker
Docker is a containerization platform that allows developers to package applications and their dependencies into isolated containers. These containers can be run on any machine that has Docker installed, providing a consistent environment regardless of the underlying infrastructure. By using Docker, developers can create a standardized testing environment that closely mirrors the production setup, reducing the chances of environment-related issues.
Implementing Docker in Testing
Dockerizing the Test Environment
The first step in leveraging Docker for test environment stability is to dockerize the test environment. This involves creating a Dockerfile that specifies the environment's configuration, including base images, dependencies, and any required setup steps. Let's take a look at an example Dockerfile for a Java application:
# Use a base image with Java
FROM openjdk:11
# Set the working directory in the container
WORKDIR /app
# Copy the application JAR file into the container
COPY target/my-application.jar /app
# Specify the command to run the application
CMD ["java", "-jar", "my-application.jar"]
In this Dockerfile, we start with a base image containing Java, set the working directory, copy the application's JAR file into the container, and define the command to run the application. This encapsulates the test environment and ensures consistent execution across different environments.
Docker Compose for Test Dependencies
In complex applications, dependencies such as databases, message brokers, or external services are often required for testing. Docker Compose is a tool for defining and running multi-container Docker applications. It allows developers to describe the services and their dependencies in a single file, making it easy to spin up and tear down test environments.
Let's consider an example Docker Compose file for setting up a test environment with a MySQL database alongside the Java application:
version: '3.8'
services:
app:
build: .
depends_on:
- db
environment:
DATABASE_URL: jdbc:mysql://db:3306/my_database
DATABASE_USERNAME: root
DATABASE_PASSWORD: secret
db:
image: mysql:5.7
environment:
MYSQL_ROOT_PASSWORD: secret
MYSQL_DATABASE: my_database
In this Docker Compose file, we define two services: the Java application (app
) and the MySQL database (db
). The depends_on
directive ensures that the database is started before the application. By using Docker Compose, the entire test environment, including dependencies, can be managed as code and easily reproduced across different development environments.
Benefits of Using Docker for Testing
Consistent Environments
By containerizing the test environment with Docker, developers can ensure that the environment is consistent across different stages of the development lifecycle. This consistency minimizes the "works on my machine" problem and reduces the likelihood of environment-related issues.
Isolation and Reproducibility
Docker containers provide isolation, allowing tests to run in a controlled environment without impacting the developer's machine or other services. Additionally, Docker's declarative approach to defining environments with Dockerfiles and Docker Compose files enables easy reproduction of test setups.
Scalability and Parallel Testing
Docker's lightweight containers make it practical to spin up multiple instances of the test environment in parallel, enabling faster test execution and scalability. This is particularly beneficial for continuous integration and large test suites.
Best Practices for Docker in Testing
Use Minimal Base Images
When creating Docker images for testing, it's advisable to use minimal base images to reduce the image size and minimize potential vulnerabilities. For Java applications, using official OpenJDK images with the desired version is a common best practice.
Leverage Caching for Dependencies
Docker's layer-based architecture allows for efficient caching of image layers. To expedite the build process, dependencies such as Maven or Gradle artifacts can be cached by leveraging Docker's layer caching mechanism.
Automate Test Workflows with CI/CD
Integrating Docker-based test environments into continuous integration and continuous delivery (CI/CD) pipelines helps automate test workflows. This ensures that tests are run in consistent environments and facilitates the adoption of DevOps practices.
The Last Word
In conclusion, Docker provides a powerful solution for improving test environment stability in software development. By containerizing test environments, leveraging Docker Compose for managing dependencies, and adhering to best practices, developers can create consistent, isolated, and scalable test environments. This ultimately leads to higher code quality, fewer environment-related issues, and a smoother development workflow.
To delve deeper into Docker-based testing strategies, check out this insightful article on Testing Java Applications with Docker. Additionally, for practical guidance on Docker Compose usage, refer to the official Docker Compose documentation.
Implementing Docker in testing isn't without its challenges, but the benefits far outweigh the initial effort. With Docker, software teams can lay the foundation for reliable and reproducible testing environments, setting the stage for improved software quality and faster delivery cycles.