Effective Techniques for Java Testing
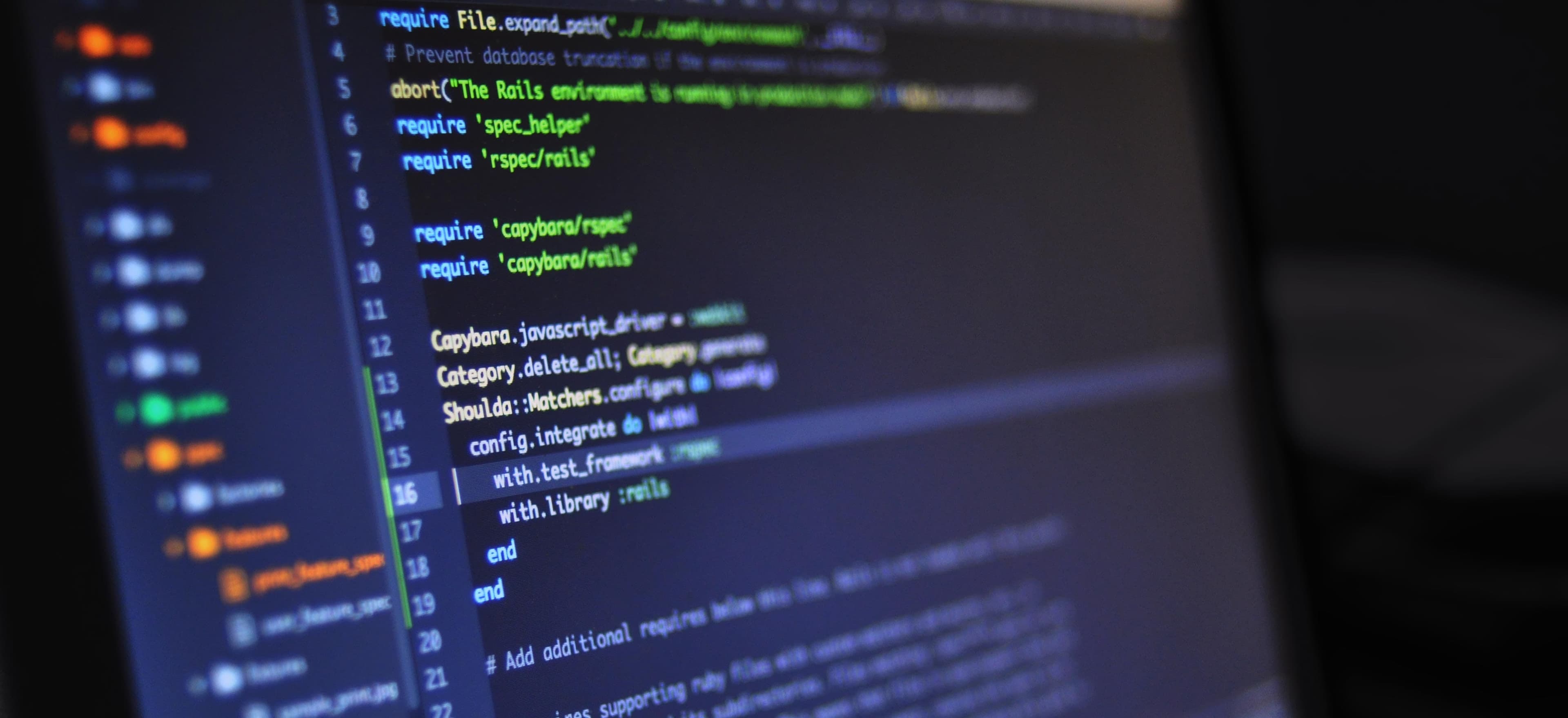
- Published on
Effective Techniques for Java Testing
Testing is an essential aspect of software development, ensuring the reliability and stability of applications. In Java, testing plays a crucial role in delivering high-quality software. This article will explore effective testing techniques in Java, including unit testing, integration testing, and mocking. We'll also delve into popular testing frameworks such as JUnit and Mockito, providing code examples and best practices.
Unit Testing in Java
Unit testing is the foundational level of testing where individual units or components of a software are tested in isolation. In Java, JUnit is the de facto framework for unit testing. Let's consider a simple example of testing a Calculator
class using JUnit.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 4);
assertEquals(7, result);
}
}
In this example, we are testing the add
method of the Calculator
class. We create an instance of the Calculator
, invoke the add
method with test data, and assert the expected result using assertEquals
.
Integration Testing in Java
Integration testing focuses on testing the interactions between different components or modules of an application. In Java, integration testing can be achieved using frameworks like Spring's TestContext framework or TestNG. Let's consider a simple integration test using Spring's TestContext framework.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.web.client.TestRestTemplate;
import org.springframework.boot.web.server.LocalServerPort;
import org.springframework.http.ResponseEntity;
import org.springframework.test.context.junit.jupiter.SpringJUnitConfig;
import org.junit.jupiter.api.Test;
@SpringJUnitConfig
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class UserControllerIntegrationTest {
@LocalServerPort
private int port;
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testGetUserById() {
ResponseEntity<String> response = restTemplate.getForEntity("http://localhost:" + port + "/users/1", String.class);
assertEquals("User 1", response.getBody());
}
}
In this example, we are testing the UserController
by sending an HTTP GET request to retrieve a user by ID.
Mocking in Java Testing
Mocking is a technique used to simulate the behavior of external dependencies or complex objects during testing. In Java, Mockito is a popular mocking framework. Let's consider an example of using Mockito to mock a UserService
dependency in a test for the UserController
.
import static org.mockito.Mockito.when;
import static org.mockito.ArgumentMatchers.anyLong;
@RunWith(MockitoJUnitRunner.class)
public class UserControllerTest {
@Mock
private UserService userService;
@InjectMocks
private UserController userController;
@Test
public void testGetUserById() {
when(userService.getUserById(anyLong())).thenReturn("User 1");
String result = userController.getUserById(1);
assertEquals("User 1", result);
}
}
In this example, we use Mockito to mock the UserService
dependency and define its behavior for the getUserById
method.
Best Practices for Java Testing
- Isolate Tests: Ensure that each test is independent and does not rely on the state from other tests.
- Use Descriptive Names: Name your test methods and classes descriptively to convey their purpose.
- Test Coverage: Aim for a high test coverage by testing critical paths and edge cases.
- Refactor Tests: Refactor your test code to keep it clean and maintainable.
Closing the Chapter
In this article, we explored effective testing techniques in Java, including unit testing, integration testing, and mocking. We also covered popular testing frameworks such as JUnit and Mockito, showcasing code examples and best practices. By following these techniques and best practices, Java developers can ensure the reliability and quality of their software applications.
Testing is crucial in software development to maintain the robustness and stability of applications. Proper testing techniques like unit testing, integration testing, and mocking in Java play a significant role in ensuring the quality of software. By leveraging tools like JUnit and Mockito, developers can effectively test their code and build more reliable and maintainable applications. If you want to learn more about Java testing, check out this comprehensive guide to Java testing for in-depth knowledge.