Optimizing Transaction Synchronization in Spring
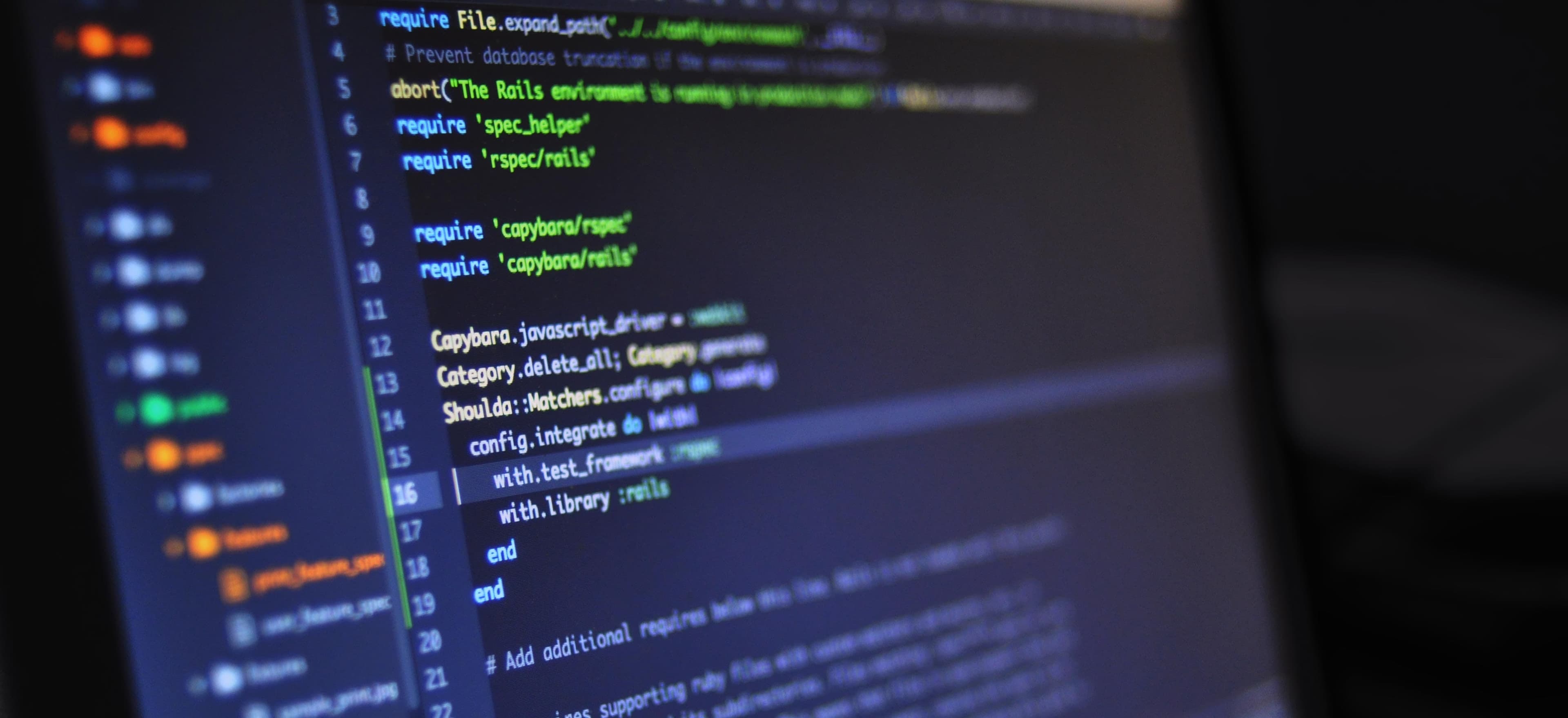
- Published on
Maximizing Transaction Synchronization in Spring: A Comprehensive Guide
When working with Spring applications, transaction management plays a crucial role in ensuring data integrity and consistency. One aspect of transaction management that often requires optimization is transaction synchronization. In this article, we'll delve into the concept of transaction synchronization in the context of Spring framework and explore strategies and best practices to maximize its efficiency.
Understanding Transaction Synchronization
In the world of Spring framework, transaction synchronization involves coordinating actions with the transaction lifecycle. These actions can include database operations, resource cleanup, and other tasks that need to be executed before, after, or during a transaction. Spring provides the TransactionSynchronization
interface which allows developers to register synchronization callbacks that are invoked at various points in the transaction lifecycle, such as before and after commit, before and after completion, and in case of rollback.
When dealing with complex business logic and data access requirements, it's essential to ensure that transaction synchronization is optimized to minimize the risk of data inconsistencies and resource leaks.
Leveraging Spring's TransactionSynchronizationManager
Spring's TransactionSynchronizationManager
serves as a vital component in managing transaction synchronization. It provides methods to register and notify synchronization callbacks, as well as to retrieve resources associated with the current transaction. When leveraging transaction synchronization in Spring, it's important to understand how to effectively use TransactionSynchronizationManager
in order to achieve optimal performance and reliability.
Let's consider an example scenario where we need to perform custom logic after a transaction is committed. We can achieve this by registering a synchronization callback using TransactionSynchronizationManager
:
public class CustomSynchronizationCallback implements TransactionSynchronization {
@Override
public void afterCommit() {
// Perform custom logic after transaction commit
}
// Implement other synchronization methods as needed
}
// Within a service or component
public class MyTransactionalService {
public void performTransactionalOperation() {
// Perform business logic within a transaction
// Register custom synchronization callback
TransactionSynchronizationManager.registerSynchronization(new CustomSynchronizationCallback());
}
}
In the above example, the CustomSynchronizationCallback
implements the TransactionSynchronization
interface and defines the custom logic to be executed after the transaction is committed. By registering this synchronization callback using TransactionSynchronizationManager
, we ensure that the custom logic is invoked at the appropriate phase of the transaction lifecycle.
Best Practices for Optimizing Transaction Synchronization
Optimizing transaction synchronization in Spring involves adhering to best practices that can enhance performance, maintainability, and reliability of the application. Let's explore some key strategies for optimizing transaction synchronization in Spring:
1. Use TransactionTemplate for Declarative Transaction Management
Spring's TransactionTemplate
provides a higher level of abstraction for managing programmatic transactions, allowing for cleaner and more concise code. By encapsulating the transactional code within a TransactionTemplate
instance, synchronization callbacks can be registered and executed in a more structured and readable manner.
public class TransactionalService {
private final TransactionTemplate transactionTemplate;
public TransactionalService(PlatformTransactionManager transactionManager) {
this.transactionTemplate = new TransactionTemplate(transactionManager);
}
public void performTransactionalOperation() {
transactionTemplate.execute(status -> {
// Perform business logic within a transaction
TransactionSynchronizationManager.registerSynchronization(new CustomSynchronizationCallback());
return null; // Return value as needed
});
}
}
Utilizing TransactionTemplate
not only simplifies the management of transaction synchronization, but also promotes consistent error handling and resource cleanup.
2. Consider Asynchronous Execution for Expensive Synchronization Tasks
In scenarios where synchronization tasks are computationally expensive or involve blocking operations, consider offloading these tasks to asynchronous execution mechanisms such as Spring's @Async
annotation or utilizing reactive programming paradigms. This can prevent transactional tasks from imposing unnecessary delays on the main transaction flow and enhance overall system responsiveness.
3. Implement Conditional Synchronization Logic
To minimize unnecessary overhead, carefully evaluate the conditions under which synchronization callbacks need to be executed. By implementing conditional logic within synchronization callbacks, developers can avoid redundant operations and optimize the use of resources.
4. Leverage Resource Cleanup and Transactional Resource Management
In addition to custom logic execution, transaction synchronization serves as a vital mechanism for cleaning up resources associated with the transaction. This includes releasing database connections, closing file handles, and releasing external resources. By leveraging Spring's transaction synchronization capabilities, these resource cleanup tasks can be seamlessly integrated into the transaction lifecycle, ensuring efficient resource utilization and preventing resource leaks.
To Wrap Things Up
In the realm of Spring framework, optimizing transaction synchronization is imperative for maintaining data consistency, efficient resource management, and robust application behavior. By harnessing the capabilities of Spring's transaction synchronization mechanism and adhering to best practices, developers can elevate the performance and reliability of their transactional operations.
Enhancing transaction synchronization not only contributes to the overall efficiency of Spring applications but also fosters a more resilient and maintainable codebase.
In conclusion, mastering transaction synchronization in Spring facilitates the creation of highly efficient and reliable transaction management processes. By incorporating the discussed best practices and techniques, developers can maximize the performance and maintainability of their Spring applications, ensuring seamless coordination of actions within the transaction lifecycle.
For more in-depth insights into Spring transaction management, refer to the official Spring Framework Documentation and Official Spring Blog.