Clearing stale data from the reference data cache in Singleton EJBs
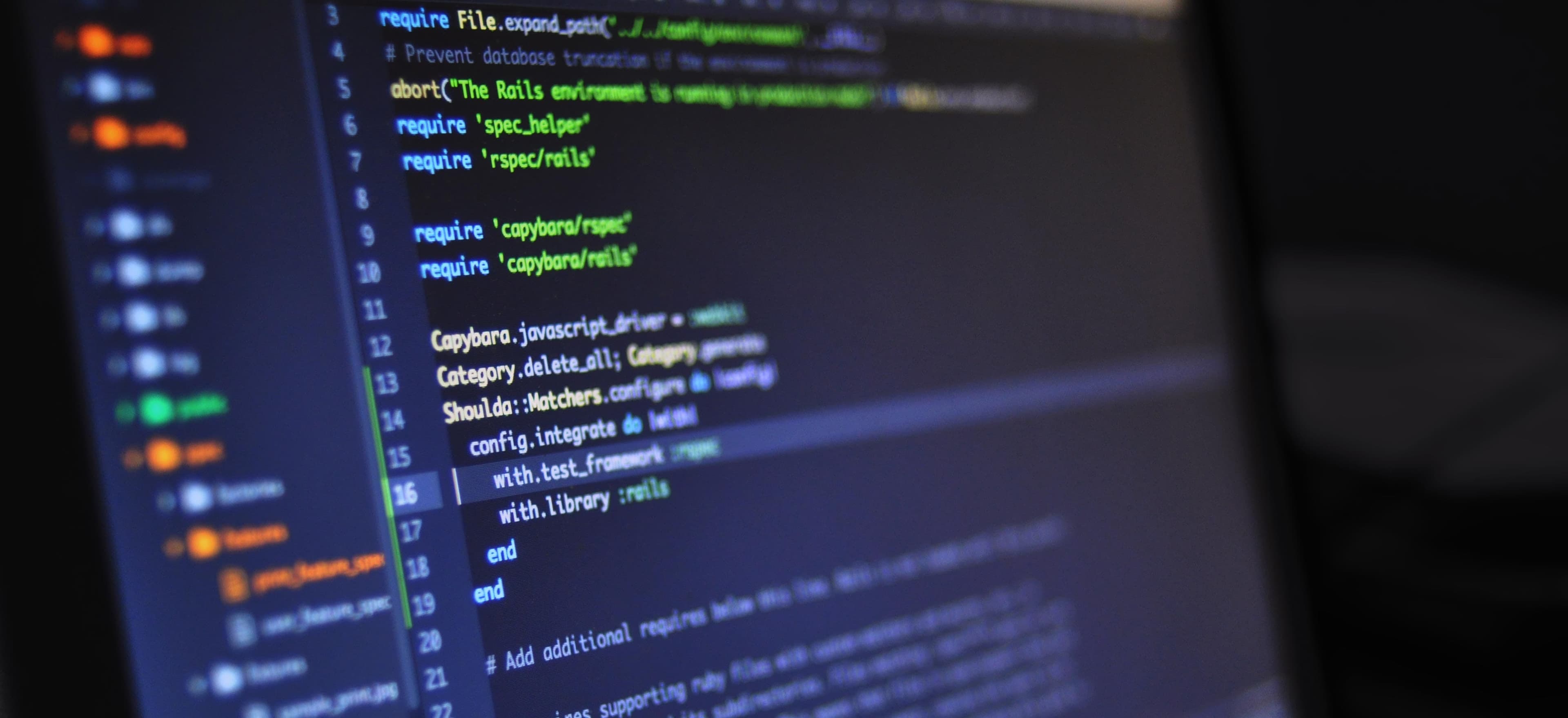
- Published on
In the world of enterprise Java, applications often rely on caching reference data to improve performance. However, this cached data can become stale over time, leading to inconsistencies and potentially incorrect behavior in the application. In this article, we'll explore how to clear stale data from the reference data cache in Singleton EJBs, ensuring the integrity and reliability of our Java applications.
Understanding Singleton EJBs
Singleton Enterprise JavaBeans (EJBs) are a special type of EJB that allows only one instance for a specific application in the same virtual machine. This makes them suitable for managing shared data and resources across the application. Singleton EJBs are often used to cache reference data, such as configuration settings, user roles, or other non-changing information that is frequently accessed by the application.
The Challenge of Stale Data
While caching reference data in Singleton EJBs can significantly improve application performance, it also introduces the challenge of dealing with stale data. As the cached data is not updated automatically, there is a risk that the information becomes outdated or inconsistent with the actual data source. This can lead to erroneous behavior and undermine the reliability of the application.
Clearing Stale Data
To address the issue of stale data in Singleton EJBs, it's important to implement a mechanism to periodically clear the cached data and refresh it from the data source. This process ensures that the application always has access to the most up-to-date reference data, maintaining correctness and consistency.
Using @Schedule Annotation for Scheduled Cache Refresh
One effective approach for clearing stale data in Singleton EJBs is to utilize the @Schedule
annotation in Java EE. This annotation allows us to specify a method that should be executed at regular intervals, effectively enabling us to schedule cache refresh operations.
Let's take a look at an example of using the @Schedule
annotation to periodically refresh the reference data cache in a Singleton EJB:
@Singleton
@Startup
public class ReferenceDataCacheManager {
@EJB
private ReferenceDataService referenceDataService;
private Map<String, Object> cache;
@Schedule(hour = "*", minute = "*/30", persistent = false)
public void refreshCache() {
// Clear the existing cache
cache.clear();
// Fetch fresh reference data from the data source
Map<String, Object> freshData = referenceDataService.loadReferenceData();
// Update the cache with the fresh data
cache.putAll(freshData);
}
// Other methods for accessing the cached data
}
In this example, the ReferenceDataCacheManager
is a Singleton EJB responsible for managing the reference data cache. The @Schedule
annotation on the refreshCache
method specifies that the method should be executed every 30 minutes. Within the method, the existing cache is cleared, and fresh reference data is retrieved from the ReferenceDataService
EJB and stored in the cache.
Why It Works
By using the @Schedule
annotation to periodically refresh the reference data cache in the Singleton EJB, we ensure that the cached data stays current and accurate. This proactive approach minimizes the risk of stale data negatively impacting the application's behavior and maintains the integrity of the cached reference data.
Handling Concurrent Access
While refreshing the cache, we need to consider potential concurrent access to the Singleton EJB, as multiple threads may attempt to access the cached data during the refresh process. To ensure thread-safety and consistency, it's important to implement proper synchronization mechanisms, such as using concurrent data structures like ConcurrentHashMap
for the cache.
private Map<String, Object> cache = new ConcurrentHashMap<>();
By utilizing ConcurrentHashMap
for the cache implementation, we guarantee thread-safe operations for concurrent access, avoiding potential data corruption or inconsistencies during cache refresh.
Closing Remarks
In conclusion, clearing stale data from the reference data cache in Singleton EJBs is crucial for maintaining the reliability and consistency of Java enterprise applications. By leveraging the @Schedule
annotation for scheduled cache refresh and addressing concurrent access concerns, we can ensure that the cached reference data remains up-to-date and accurate, ultimately enhancing the performance and correctness of our applications.
Implementing a robust cache management strategy in Singleton EJBs not only optimizes application performance but also demonstrates a proactive approach to maintaining data integrity, fostering a more reliable and efficient Java enterprise environment.
To delve deeper into the world of Singleton EJBs and caching strategies, consider exploring the official Oracle documentation on Enterprise JavaBeans and Java Concurrency in Practice by Brian Goetz and team. Happy coding!