Financial Customer Evaluation: Streamlining Cloud App Development
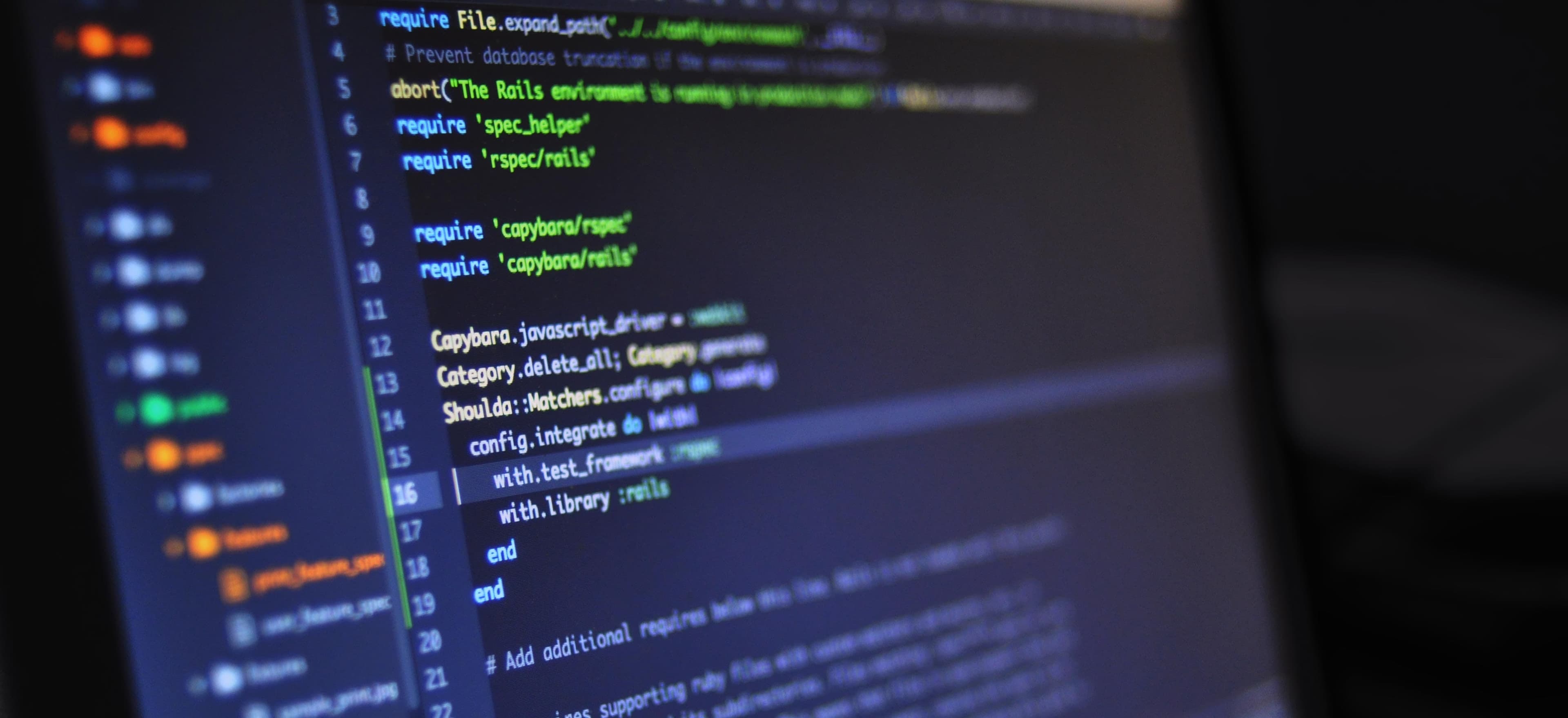
- Published on
Streamlining Cloud App Development for Financial Customer Evaluation using Java
In the competitive landscape of financial services, providing efficient and secure customer evaluation is crucial. With the increasing demand for cloud-based applications, Java has emerged as a go-to language for developing scalable and robust solutions. In this article, we'll explore how to streamline cloud app development for financial customer evaluation using Java.
Efficient Data Handling with Java
Java offers a rich set of libraries and tools for efficient data handling. When dealing with financial customer evaluation, data security and integrity are of paramount importance. Java provides robust features for encryption, data serialization, and secure communication, making it an ideal choice for handling sensitive financial data.
// Example of data encryption in Java
public class DataEncryption {
public static void main(String[] args) {
String dataToEncrypt = "Sensitive financial data";
// Use Java Cryptography Architecture (JCA) for encryption
// Implement proper key management and encryption algorithms
}
}
By leveraging Java's capabilities for data handling, developers can ensure that customer data is securely processed and stored in cloud-based applications.
Asynchronous Processing for Scalability
Scalability is a key consideration for cloud-based applications, especially in the context of financial customer evaluation where the volume of data can vary significantly. Java's support for asynchronous programming through features like CompletableFuture and Executor framework allows for efficient utilization of resources, enabling the application to handle multiple concurrent requests without sacrificing performance.
// Example of asynchronous processing in Java
import java.util.concurrent.CompletableFuture;
public class AsynchronousProcessing {
public static void main(String[] args) {
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// Asynchronous tasks for processing customer evaluation data
});
// Continue with other processing tasks
}
}
By incorporating asynchronous processing in Java, developers can design cloud applications that can seamlessly scale to meet the demands of financial customer evaluation processes.
Microservices Architecture with Spring Boot
When developing cloud-based applications, adopting a microservices architecture provides flexibility, resilience, and scalability. Java, in combination with the Spring Boot framework, offers a powerful ecosystem for building and deploying microservices. By breaking down the customer evaluation process into smaller, independent services, developers can iterate and scale each component individually, leading to a more agile and resilient application architecture.
// Example of defining a microservice endpoint with Spring Boot
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class CustomerEvaluationController {
@GetMapping("/evaluate")
public String evaluateCustomer() {
// Implement customer evaluation logic
return "Evaluation result";
}
}
Utilizing Spring Boot for microservices development in Java enables financial institutions to create a modular and robust architecture for customer evaluation applications in the cloud.
Integration with Cloud Services
Java provides extensive support for integrating with various cloud services and platforms. Whether it's leveraging Amazon Web Services (AWS) for storage and computing, or integrating with Microsoft Azure for AI and machine learning capabilities, Java's ecosystem offers libraries and SDKs to seamlessly connect with these cloud services.
// Example of AWS S3 integration in Java
import software.amazon.awssdk.services.s3.S3Client;
import software.amazon.awssdk.services.s3.model.PutObjectRequest;
public class S3Integration {
public static void main(String[] args) {
S3Client s3Client = S3Client.create();
// Perform operations such as uploading customer evaluation data to S3
}
}
By integrating with cloud services using Java, developers can harness the full potential of cloud computing for financial customer evaluation, encompassing features like scalability, security, and advanced analytics.
To Wrap Things Up
In the realm of financial services, the need for efficient and secure customer evaluation is non-negotiable. Java's robust features for data handling, asynchronous processing, microservices development, and cloud service integration make it an optimal choice for streamlining cloud app development for financial customer evaluation. By leveraging Java's capabilities, financial institutions can build scalable, resilient, and secure applications that meet the evolving demands of customer evaluation in the cloud.
When developing cloud applications in Java, it's essential to stay updated with the latest trends and best practices. This ensures that your solutions remain competitive and efficient in the dynamic landscape of financial services and cloud computing.
So, are you ready to streamline cloud app development for financial customer evaluation using Java? Share your thoughts and experiences with us!
Organizations like Oracle and IBM offer significant insights into the latest advancements and best practices for Java development in the financial services sector.