Improving File Serialization Performance in Java
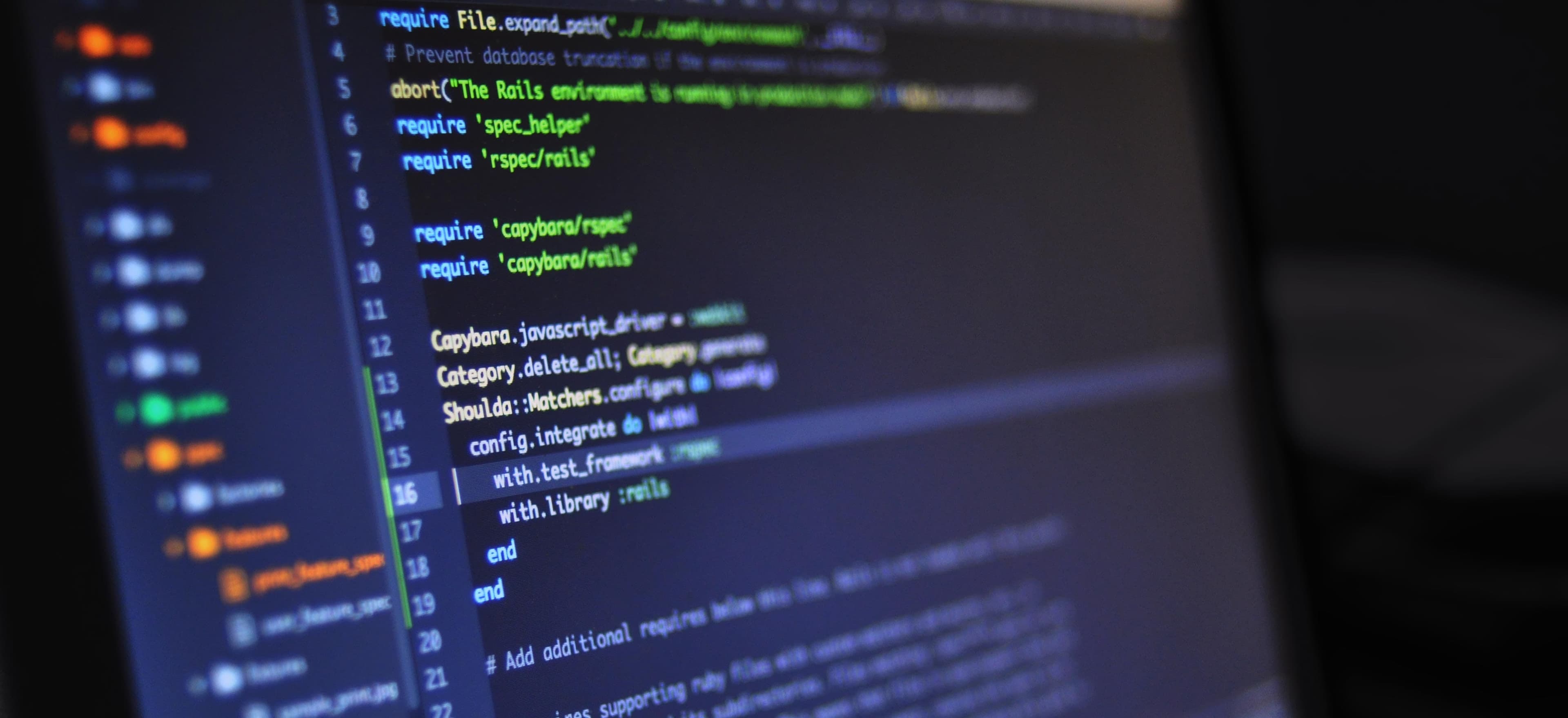
- Published on
Improving File Serialization Performance in Java
Serialization is the process of converting an object into a stream of bytes to store the object or transmit it to memory, a database, or a file. In Java, the serialization process can be performance-intensive and may lead to inefficiencies, especially when dealing with large datasets. In this article, we will explore some techniques to improve file serialization performance in Java.
1. Use Buffered I/O Streams
When performing file serialization in Java, it's essential to use buffered I/O streams such as BufferedInputStream
and BufferedOutputStream
. These streams reduce the number of I/O operations by reading or writing data in chunks, thus minimizing disk access and improving performance.
FileOutputStream fileOutputStream = new FileOutputStream("data.txt");
BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(fileOutputStream);
ObjectOutputStream objectOutputStream = new ObjectOutputStream(bufferedOutputStream);
objectOutputStream.writeObject(yourObject);
objectOutputStream.close();
In this example, we create a BufferedOutputStream
to wrap around the FileOutputStream
, and then we pass it to the ObjectOutputStream
. This chaining of streams increases the efficiency of serialization by reducing the frequency of write operations to the disk.
2. Implement Custom Serialization
Java provides default serialization behavior for objects, but in some cases, implementing custom serialization can significantly improve performance. By implementing the Serializable
interface and defining custom writeObject
and readObject
methods, you can have finer control over the serialization process.
public class CustomObject implements Serializable {
private void writeObject(ObjectOutputStream out) throws IOException {
// Custom serialization logic
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
// Custom deserialization logic
}
}
Custom serialization allows you to optimize the serialization process by selectively serializing object fields, handling transient data, and managing complex data structures more efficiently.
3. Use Externalizable Interface
Another approach to improve serialization performance is by implementing the Externalizable
interface. Unlike the Serializable
interface, which performs automatic serialization of all non-transient fields, the Externalizable
interface gives you complete control over the serialization and deserialization process.
public class CustomObject implements Externalizable {
@Override
public void writeExternal(ObjectOutput out) throws IOException {
// Custom serialization logic
}
@Override
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
// Custom deserialization logic
}
}
By implementing the writeExternal
and readExternal
methods, you can optimize the serialization process by directly manipulating the data output and input, leading to improved performance.
4. Consider Compression
In scenarios where the size of the serialized data is a concern, employing compression techniques can aid in improving file serialization performance. Java provides classes such as GZIPOutputStream
and GZIPInputStream
that allow you to apply gzip compression to the serialized data.
FileOutputStream fileOutputStream = new FileOutputStream("data.gz");
GZIPOutputStream gzipOutputStream = new GZIPOutputStream(fileOutputStream);
ObjectOutputStream objectOutputStream = new ObjectOutputStream(gzipOutputStream);
objectOutputStream.writeObject(yourObject);
objectOutputStream.close();
By utilizing compression, you can reduce the disk space and I/O operations required to store and retrieve the serialized data, thus enhancing the overall serialization performance.
5. Optimize Object Graphs
When dealing with complex object graphs, the serialization process can become resource-intensive. One way to address this is by optimizing the object graphs to minimize the amount of data being serialized. You can achieve this by selectively serializing only essential data and avoiding unnecessary inclusion of entire object graphs.
private void writeObject(ObjectOutputStream out) throws IOException {
out.writeObject(essentialField1);
out.writeObject(essentialField2);
// Serialize only essential fields
}
By carefully selecting the data to be serialized and avoiding redundant or unnecessary serialization, you can improve the performance of file serialization in Java when dealing with complex object graphs.
Bringing It All Together
In conclusion, by leveraging techniques such as using buffered I/O streams, implementing custom serialization, employing the Externalizable
interface, considering compression, and optimizing object graphs, you can significantly enhance file serialization performance in Java. These approaches not only improve the efficiency of serialization but also contribute to the overall optimization of file I/O operations in your Java applications.
By employing these techniques, you can tackle the challenges associated with performance bottlenecks in file serialization, thereby ensuring the smooth and efficient handling of data within your Java applications.
Remember, understanding the nuances of serialization and employing optimization techniques can lead to more robust and performant Java applications.
Incorporating these strategies into your Java programming toolkit can help you navigate the complexities of file serialization performance, ultimately contributing to the seamless operation and enhanced user experience of your applications.
For further insights into Java file handling and performance optimization, consider exploring the Java I/O Tutorial and Effective Java Programming to broaden your knowledge and skills in this domain.
Happy coding!