Configuring ExceptionMapper in JAX-RS
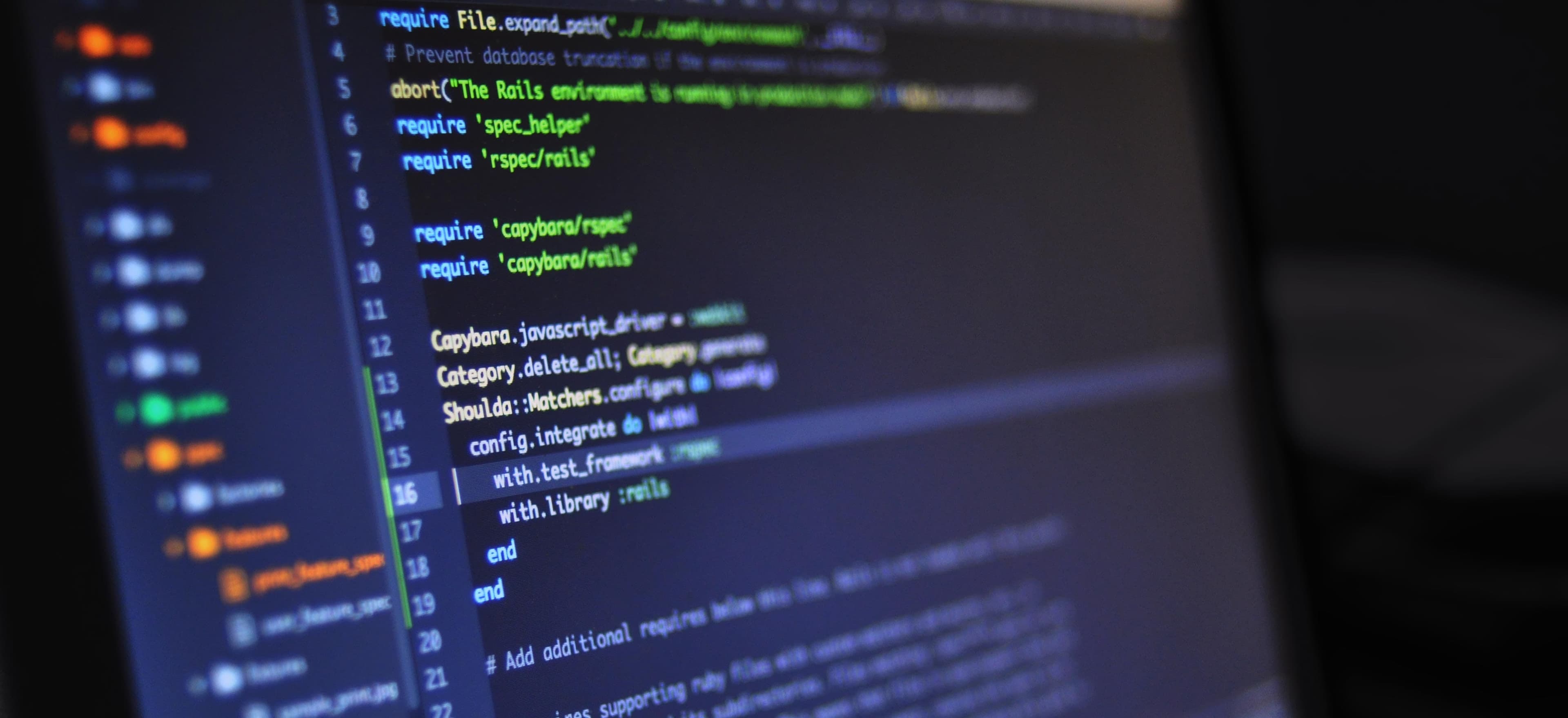
- Published on
Handling Exceptions in JAX-RS Using ExceptionMapper
Handling exceptions is an essential aspect of building robust and reliable applications. In a JAX-RS (Java API for RESTful Web Services) application, the ExceptionMapper interface provides a way to map application-specific exceptions to a response that is sent back to the client. This blog post will delve into the configuration and usage of ExceptionMapper in a JAX-RS application.
Understanding ExceptionMapper
In JAX-RS, the ExceptionMapper interface allows developers to map a given exception to a response. When an exception is thrown during the processing of a request, JAX-RS searches for an appropriate ExceptionMapper to handle the exception. If an ExceptionMapper is found that can handle the exception, the toResponse() method of the ExceptionMapper is invoked to create a Response object, which is then sent back to the client.
Implementing an ExceptionMapper
To implement an ExceptionMapper, you need to create a class that implements the ExceptionMapper interface and provides the necessary logic to map the exception to a Response object. Let's consider an example where we have a custom NotFoundException that we want to map to a 404 Not Found response.
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class NotFoundExceptionMapper implements ExceptionMapper<NotFoundException> {
@Override
public Response toResponse(NotFoundException exception) {
return Response.status(Response.Status.NOT_FOUND)
.entity("Resource not found")
.build();
}
}
In this example, we have created a NotFoundExceptionMapper class that implements the ExceptionMapper interface for the NotFoundException. The @Provider annotation marks this class as an extension that should be discovered and registered dynamically by JAX-RS.
The toResponse() method is where the logic for mapping the exception to a Response object resides. In this case, we are creating a 404 Not Found response with a custom message "Resource not found".
Registering an ExceptionMapper
To ensure that the ExceptionMapper is utilized by the JAX-RS runtime, it needs to be registered. This can be done in a number of ways, depending on the specific JAX-RS implementation being used. One common approach is to utilize the Application subclass to register the ExceptionMapper.
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
import java.util.HashSet;
import java.util.Set;
@ApplicationPath("/api")
public class MyApplication extends Application {
@Override
public Set<Class<?>> getClasses() {
Set<Class<?>> classes = new HashSet<>();
classes.add(NotFoundExceptionMapper.class);
return classes;
}
}
In this example, the MyApplication class extends javax.ws.rs.core.Application and is annotated with @ApplicationPath to specify the base URI for all resource URIs in the application. The getClasses() method returns a set of all the resource and provider classes for the application, where we add the NotFoundExceptionMapper class to ensure it is registered.
Utilizing ExceptionMappers
Once the ExceptionMapper is implemented and registered, it will be automatically invoked when an exception of the specified type is thrown. Let's take a look at how it works in a resource class.
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
@Path("/books")
public class BookResource {
@GET
@Path("/{id}")
public String getBookById(@PathParam("id") int id) {
// logic to retrieve book by id
if (/* book not found */) {
throw new NotFoundException("Book not found");
}
return "Book found";
}
}
In the BookResource class, when the getBookById() method is invoked with a specific book ID, if the book is not found, a NotFoundException is thrown. As the NotFoundExceptionMapper is registered, it will handle the exception and respond with a 404 Not Found along with the message "Resource not found".
Lessons Learned
In this blog post, we have explored the ExceptionMapper interface in JAX-RS and its role in handling exceptions by mapping them to appropriate responses. By creating custom ExceptionMappers, developers can effectively manage and communicate errors within their RESTful web services. Proper utilization of ExceptionMappers contributes to the overall reliability and stability of JAX-RS applications.
To expand your understanding of JAX-RS and ExceptionMapper, check out Oracle's official documentation and Jersey's ExceptionMapper guide.