Understanding Java Memory Model: Concurrency Simplified
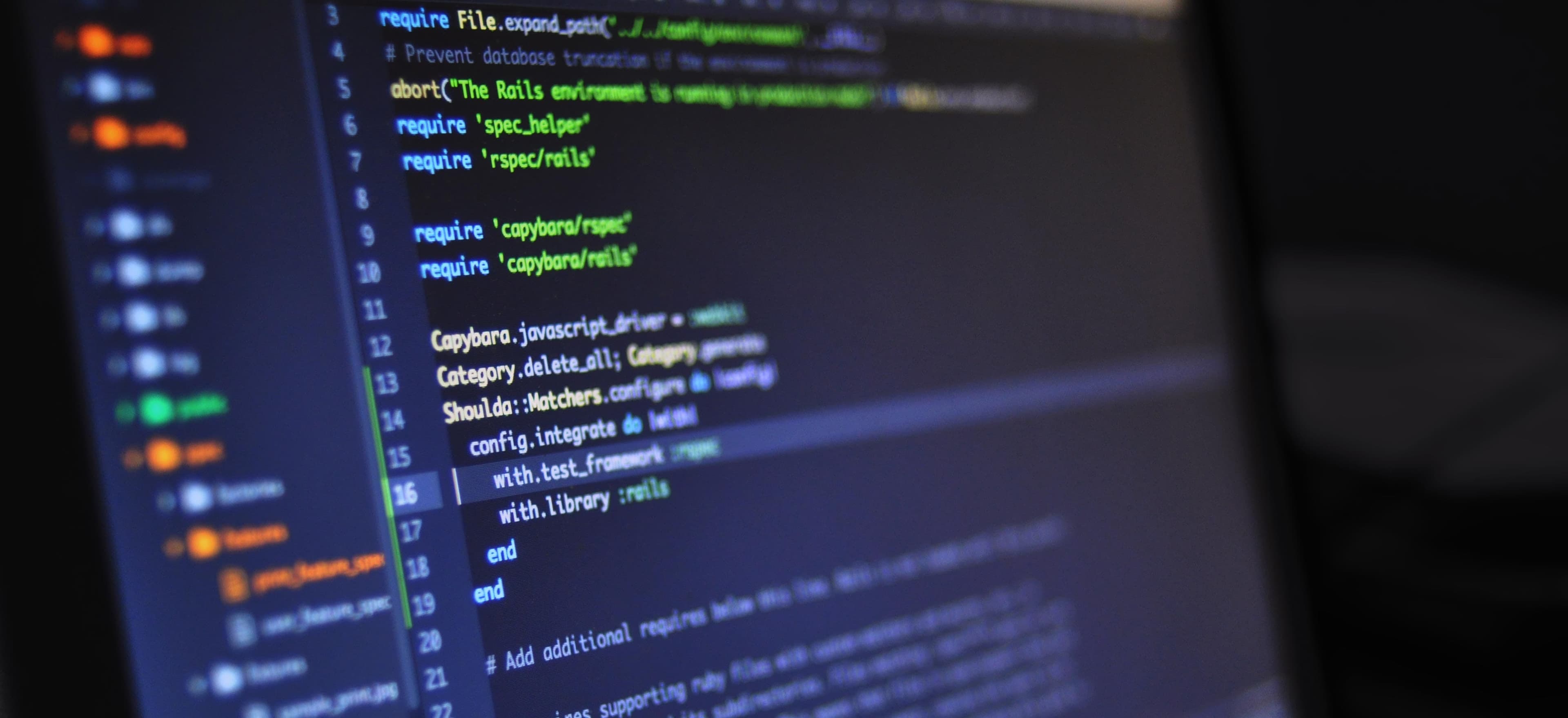
- Published on
Understanding Java Memory Model: Concurrency Simplified
Java is a versatile and powerful programming language known for its ability to handle complex and concurrent operations, thanks in part to its memory model. The Java Memory Model (JMM) defines the interactions of different threads through memory and provides the guidelines for writing concurrent code. In this article, we will explore the fundamentals of the Java Memory Model, its impact on concurrent programming, and best practices for writing efficient and safe concurrent code in Java.
What is the Java Memory Model?
The Java Memory Model specifies the allowed behaviors for shared memory in a multithreaded program. It defines how and when changes to memory made by one thread become visible to other threads. Understanding the JMM is crucial for writing reliable concurrent programs in Java.
Visibility and Atomicity
One of the key concepts of the Java Memory Model is visibility. When one thread modifies shared data, other threads must eventually see the modifications. Failing to ensure visibility can lead to subtle bugs in concurrent programs. The use of the volatile
keyword in Java ensures visibility of changes to variables across threads.
Consider the following example:
public class SharedResource {
private volatile boolean flag = false;
public void toggleFlag() {
flag = !flag;
}
public boolean isFlag() {
return flag;
}
}
In this example, the volatile
keyword ensures that when the toggleFlag
method modifies the flag
variable, the change is immediately visible to other threads. Without the volatile
keyword, the change might not be propagated to other threads promptly.
Another critical aspect of JMM is atomicity. An operation is atomic if it completes as a single, indivisible unit. In Java, the synchronized
keyword and classes in the java.util.concurrent.atomic
package are used to ensure atomicity of operations on shared data.
Happens-Before Relationship
The Java Memory Model also defines the happens-before relationship, which establishes a partial ordering on memory operations for the correct synchronization between threads. Understanding happens-before is crucial when dealing with concurrent code and ensuring the expected behavior of shared data access.
Consider the following example:
public class HappensBeforeExample {
private int x = 0;
private int y = 0;
public void writeValues() {
x = 1;
y = 1;
}
public void readValues() {
int i = y;
int j = x;
}
}
In this example, the happens-before relationship ensures that if writeValues
is called before readValues
, the changes to x
and y
made by writeValues
are visible to readValues
.
Understanding happens-before is essential for writing correct and efficient concurrent programs in Java.
Best Practices for Concurrent Programming in Java
When writing concurrent programs in Java, there are several best practices to consider to ensure the reliability and efficiency of the code.
Use Thread-Safe Classes
Java provides several thread-safe classes in the java.util.concurrent
package, such as ConcurrentHashMap
, CopyOnWriteArrayList
, and ConcurrentLinkedQueue
. Utilizing these thread-safe classes can eliminate the complexities of manual synchronization and reduce the risk of concurrency bugs.
Proper Use of Synchronization
When using synchronization, it's essential to synchronize on the smallest possible block of code to minimize contention and improve performance. Over-synchronization can lead to unnecessary contention and degrade the performance of the application.
Atomic Variables
Utilize atomic variables from the java.util.concurrent.atomic
package, such as AtomicInteger
and AtomicBoolean
, to ensure atomicity without the overhead of synchronization.
Volatile Keyword
Use the volatile
keyword when multiple threads access a variable, and its value will be modified by different threads. This ensures visibility of changes to the variable across threads without the need for explicit synchronization.
Immutable Objects
Consider using immutable objects to minimize the need for synchronization. Immutable objects are inherently thread-safe, as their state cannot be modified after construction.
Executor Framework
Prefer using the Executor
framework from the java.util.concurrent
package for managing threads and running concurrent tasks. It provides higher-level abstractions for managing concurrent tasks and thread pooling.
By following these best practices, developers can write robust and efficient concurrent programs in Java while leveraging the features provided by the Java Memory Model.
Closing the Chapter
The Java Memory Model plays a crucial role in defining how threads interact through shared memory, ensuring the reliability and correctness of concurrent programs. Understanding visibility, atomicity, happens-before relationship, and best practices for concurrent programming in Java is essential for writing efficient and safe concurrent code.
In conclusion, a solid grasp of the Java Memory Model, coupled with best practices for concurrent programming, empowers developers to harness the power of concurrency in Java effectively, producing robust and reliable applications.
In summary, the Java Memory Model forms the cornerstone of concurrent programming in Java, impacting the way threads interact through shared memory and shaping the reliability and correctness of concurrent programs.
Understanding the basics of the Java Memory Model, including visibility, atomicity, and the happens-before relationship, is vital for writing efficient and safe concurrent code.
Through the application of best practices such as using thread-safe classes, proper synchronization, atomic variables, and the volatile
keyword, developers can build robust concurrent programs in Java with confidence.