Optimizing Redis Data Storage Performance
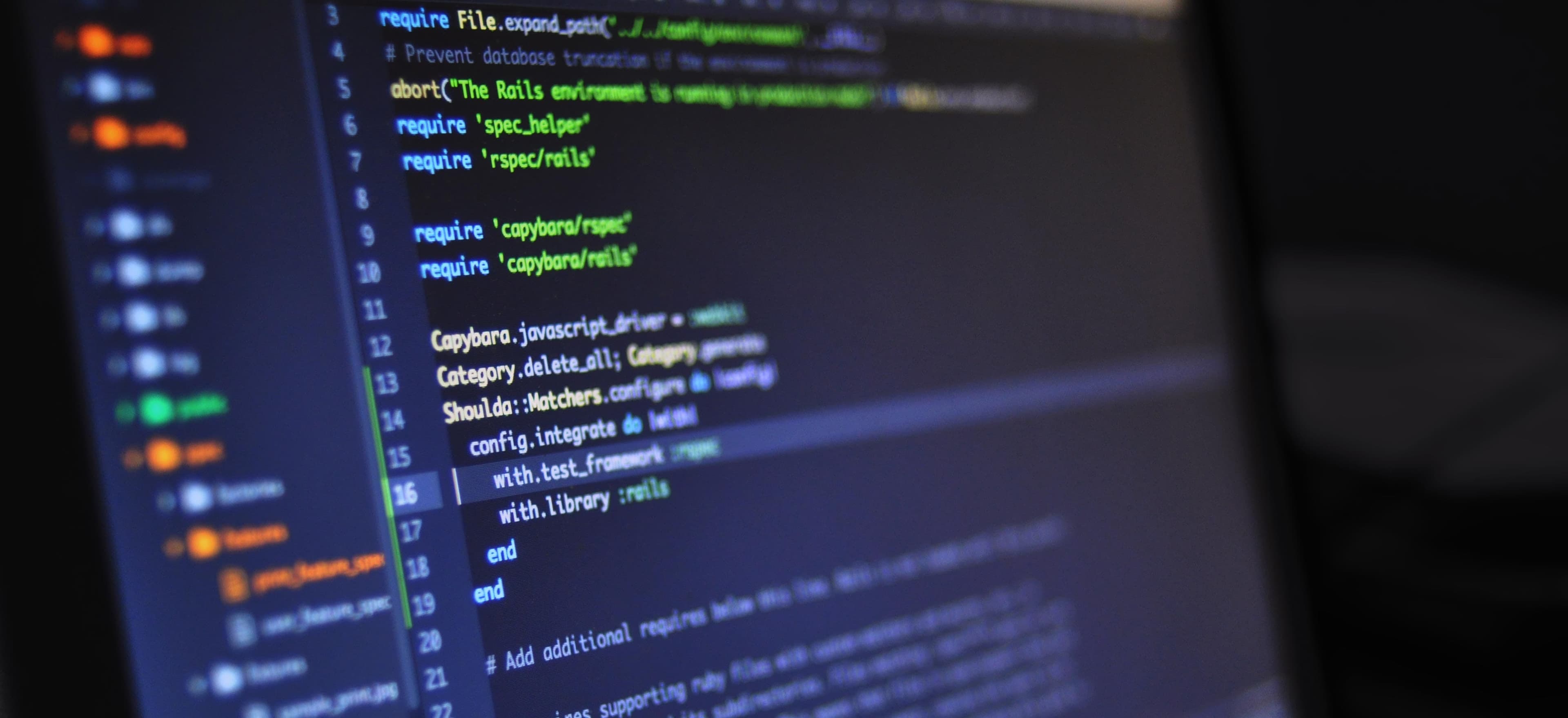
- Published on
Optimizing Redis Data Storage Performance
In the world of modern application development, optimizing data storage performance is crucial for ensuring the efficiency and scalability of an application. Redis, as an in-memory data store, offers exceptional performance, but there are specific strategies and techniques that can be employed to further optimize its data storage performance. In this article, we will delve into some of the best practices for optimizing Redis data storage performance using Java.
Utilizing Connection Pools for Efficient Resource Management
When working with Redis in a Java application, it is imperative to utilize connection pooling to efficiently manage resources. Creating a new connection for every Redis operation can lead to overhead and decreased performance. By using connection pooling libraries such as Lettuce or Jedis, developers can maintain a pool of reusable connections to Redis, thereby reducing the overhead of connection establishment and teardown.
Incorporating Lettuce for connection pooling in a Java application involves adding the following Maven dependency:
<dependency>
<groupId>io.lettuce.core</groupId>
<artifactId>lettuce-core</artifactId>
<version>6.1.4.RELEASE</version>
</dependency>
By leveraging connection pooling, you can significantly enhance the performance of your Redis data storage operations, especially in scenarios with high concurrency.
Efficient Data Serialization and Deserialization
Efficient serialization and deserialization of data payloads being stored or retrieved from Redis can greatly impact performance. In Java, the use of a fast and efficient serialization framework such as Kryo or FST can noticeably accelerate data transfer to and from Redis.
For instance, let's consider the serialization of a Java object using Kryo:
public byte[] serializeObject(Object obj) {
Kryo kryo = new Kryo();
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
Output output = new Output(outputStream);
kryo.writeClassAndObject(output, obj);
output.close();
return outputStream.toByteArray();
}
By employing efficient serialization techniques, you can minimize the size of data being stored in Redis and reduce the CPU overhead associated with serialization and deserialization. This directly contributes to improved data storage performance.
Pipeline and Batch Operations for Reduced Round-Trips
Redis supports efficient pipeline and batch operations, which can significantly reduce the number of round-trips between the client and the Redis server. In a Java application, you can leverage the pipeline feature provided by Lettuce to batch multiple commands together and send them to the Redis server in a single round-trip.
Consider the following example of using the pipeline feature in Lettuce:
RedisClient client = RedisClient.create("redis://localhost");
StatefulRedisConnection<String, String> connection = client.connect();
RedisStringCommands<String, String> syncCommands = connection.sync();
RedisFuture<String> set1 = syncCommands.set("key1", "value1");
RedisFuture<String> set2 = syncCommands.set("key2", "value2");
List<RedisFuture<String>> futures = new ArrayList<>();
futures.add(set1);
futures.add(set2);
// Execute all commands in a single round-trip
LettuceFutures.awaitAll(5, TimeUnit.SECONDS, futures.toArray(new RedisFuture[futures.size()]));
By batching multiple operations into a single round-trip, you can significantly reduce the latency associated with individual Redis operations, leading to improved data storage performance.
Effective Key and Data Structure Design
One of the fundamental aspects of optimizing Redis data storage performance is the effective design of keys and data structures. When using Redis as a key-value store, it is essential to carefully design the keys to facilitate efficient retrieval and manipulation. Additionally, choosing the appropriate data structures provided by Redis, such as Strings, Lists, Sets, Hashes, and Sorted Sets, based on the nature of the data and the operations to be performed, is crucial for achieving optimal performance.
For example, when storing time-series data, utilizing Redis' Sorted Sets can be highly advantageous due to their efficient range queries and aggregation capabilities.
Monitoring and Performance Tuning
Monitoring and performance tuning are indispensable components of optimizing Redis data storage performance. Implementing proper monitoring using tools like RedisInsight or Redis Live can provide valuable insights into the performance of Redis data storage operations. By analyzing metrics such as command latency, memory consumption, and throughput, developers can pinpoint areas that require optimization and fine-tuning.
Furthermore, Redis provides configurable options such as persistence mechanisms, eviction policies, and memory optimization settings, which can be adjusted to align with specific performance requirements.
Wrapping Up
Optimizing Redis data storage performance in a Java application is a multifaceted endeavor that encompasses various aspects, including efficient resource management, serialization, pipeline operations, data structure design, and performance monitoring. By adopting the best practices discussed in this article and leveraging the robust features of Redis in conjunction with Java, developers can achieve remarkable enhancements in data storage performance, thereby ensuring the scalability and responsiveness of their applications.