Tips for Efficiently Managing Big Data Streams
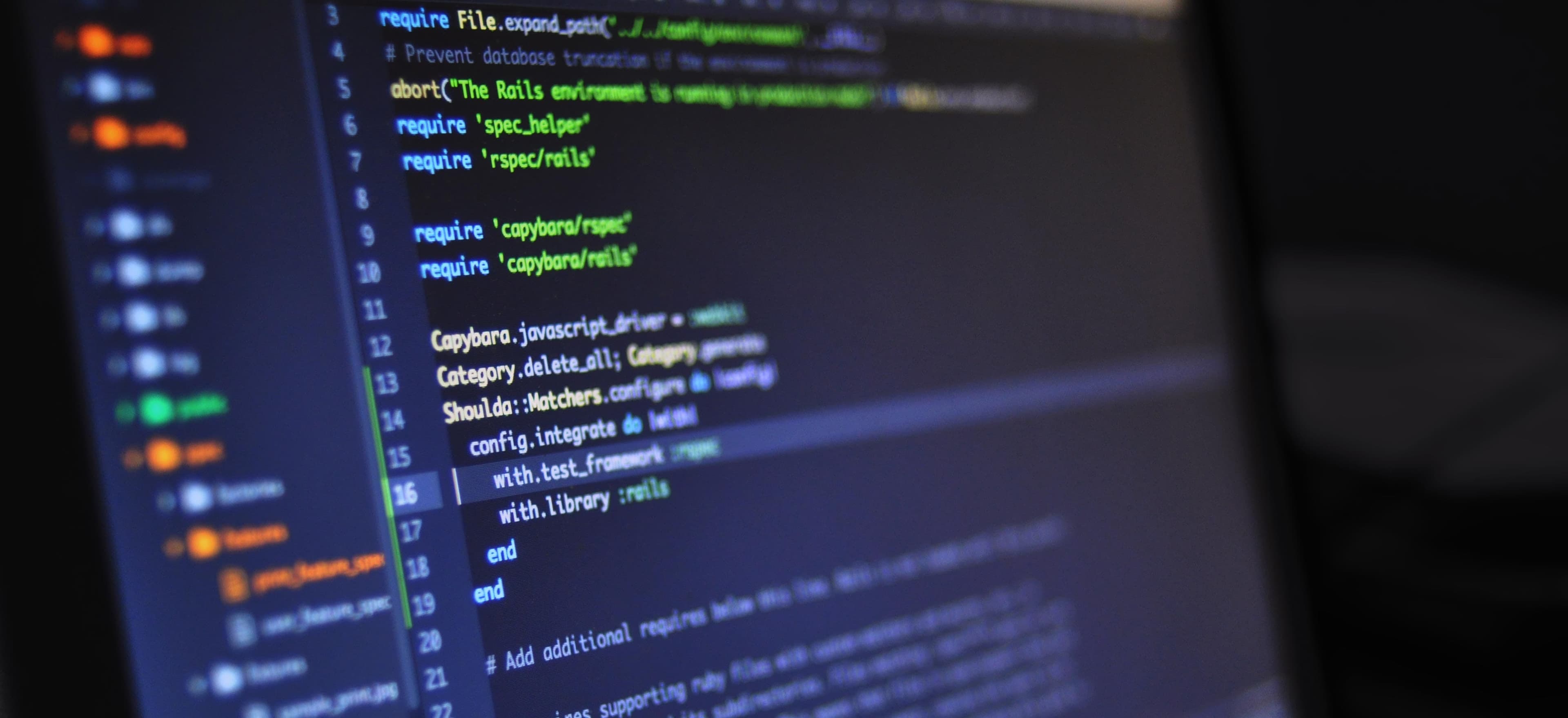
- Published on
Mastering Big Data Streams with Java
In the digital era, data is generated at an unprecedented rate. As technology advances, businesses need to efficiently process and analyze large volumes of data in real time. This is where big data streaming comes into play. Big data streaming involves processing and analyzing continuous streams of data, which can pose significant challenges in terms of scalability, real-time processing, and fault tolerance.
Java, being a robust and versatile programming language, provides an array of tools and libraries to handle big data streaming effectively. In this blog post, we will delve into some essential tips and techniques for efficiently managing big data streams using Java.
Tip 1: Leverage Apache Kafka for Stream Processing
Ah Apache Kafka is a distributed streaming platform that enables the handling of real-time data feeds. It allows for the seamless integration of various data sources and sinks, making it an ideal choice for building scalable and fault-tolerant streaming applications. Kafka's distributed nature and high throughput capabilities make it a popular choice for managing big data streams.
Let's consider a simple producer-consumer scenario using Kafka in Java:
// Kafka producer
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
producer.send(new ProducerRecord<>("topicName", "key", "value"));
producer.close();
// Kafka consumer
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("group.id", "test-group");
props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
Consumer<String, String> consumer = new KafkaConsumer<>(props);
consumer.subscribe(Arrays.asList("topicName"));
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.printf("offset = %d, key = %s, value = %s%n", record.offset(), record.key(), record.value());
}
In this example, we demonstrate how to create a Kafka producer and consumer in Java. The producer sends a message to a specified topic, while the consumer subscribes to the same topic and processes incoming messages.
Tip 2: Use Apache Flink for Real-Time Stream Processing
Apache Flink is a powerful stream processing framework that provides efficient support for event time processing, state management, and fault tolerance. Flink's ability to handle both batch and stream processing makes it an excellent choice for building real-time, data-intensive applications.
Here's an example of using Flink's DataStream API for stream processing in Java:
// Flink stream processing
StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream<String> dataStream = env.addSource(new FlinkKafkaConsumer<>("topicName", new SimpleStringSchema(), properties));
dataStream
.map(new MapFunction<String, String>() {
@Override
public String map(String value) {
// Perform data transformation
return "Processed: " + value;
}
})
.print();
env.execute("Flink Stream Processing");
In this code snippet, we create a Flink stream processing job that consumes data from a Kafka topic, performs a mapping transformation on the incoming data, and prints the processed output.
Tip 3: Implement Parallel Processing with Java Streams
Java 8 introduced the java.util.stream
package, which enables parallel processing of data using streams. Leveraging parallel streams can significantly enhance the performance of data processing operations when dealing with large datasets.
Let's consider an example of parallel stream processing in Java:
List<String> data = Arrays.asList("apple", "banana", "cherry", "date", "elderberry", "fig", "grape");
long count = data.parallelStream()
.filter(s -> s.startsWith("a"))
.count();
System.out.println("Count: " + count);
In this example, we use parallel stream processing to filter elements from a list based on a predicate and calculate the count of filtered elements. Parallel streams automatically distribute the workload across multiple CPU cores, thereby improving processing efficiency.
Tip 4: Optimize Memory Management for Big Data Streams
Efficient memory management is crucial when dealing with big data streams to avoid performance bottlenecks and potential out-of-memory errors. Java provides several mechanisms to optimize memory usage, such as using efficient data structures, minimizing object creation, and managing garbage collection.
Consider the following memory optimization techniques for big data streams in Java:
- Use primitives instead of wrapper classes for better memory utilization.
- Employ object pooling to reuse objects and reduce memory allocation overhead.
- Tune the JVM garbage collection settings based on the application's memory requirements.
By adopting these memory optimization strategies, you can ensure smooth and efficient processing of big data streams in Java.
Wrapping Up
Effectively managing big data streams is essential for building scalable, real-time data processing systems. By leveraging tools like Apache Kafka and Apache Flink, implementing parallel processing with Java streams, and optimizing memory management, developers can streamline the processing of large volumes of streaming data. Java's robust ecosystem and versatile features make it a compelling choice for handling big data streams and developing high-performance streaming applications.
In conclusion, mastering big data streams with Java requires a combination of the right tools, efficient processing techniques, and careful memory management to ensure optimal performance and scalability.
By incorporating these tips and techniques into your big data streaming projects, you can harness the full potential of Java for seamless and efficient stream processing.
Remember, the key to successful big data stream management lies in leveraging the right tools and adopting best practices to extract meaningful insights from real-time data streams. Happy streaming!