Using Java Streams with Apache Spark RDDs
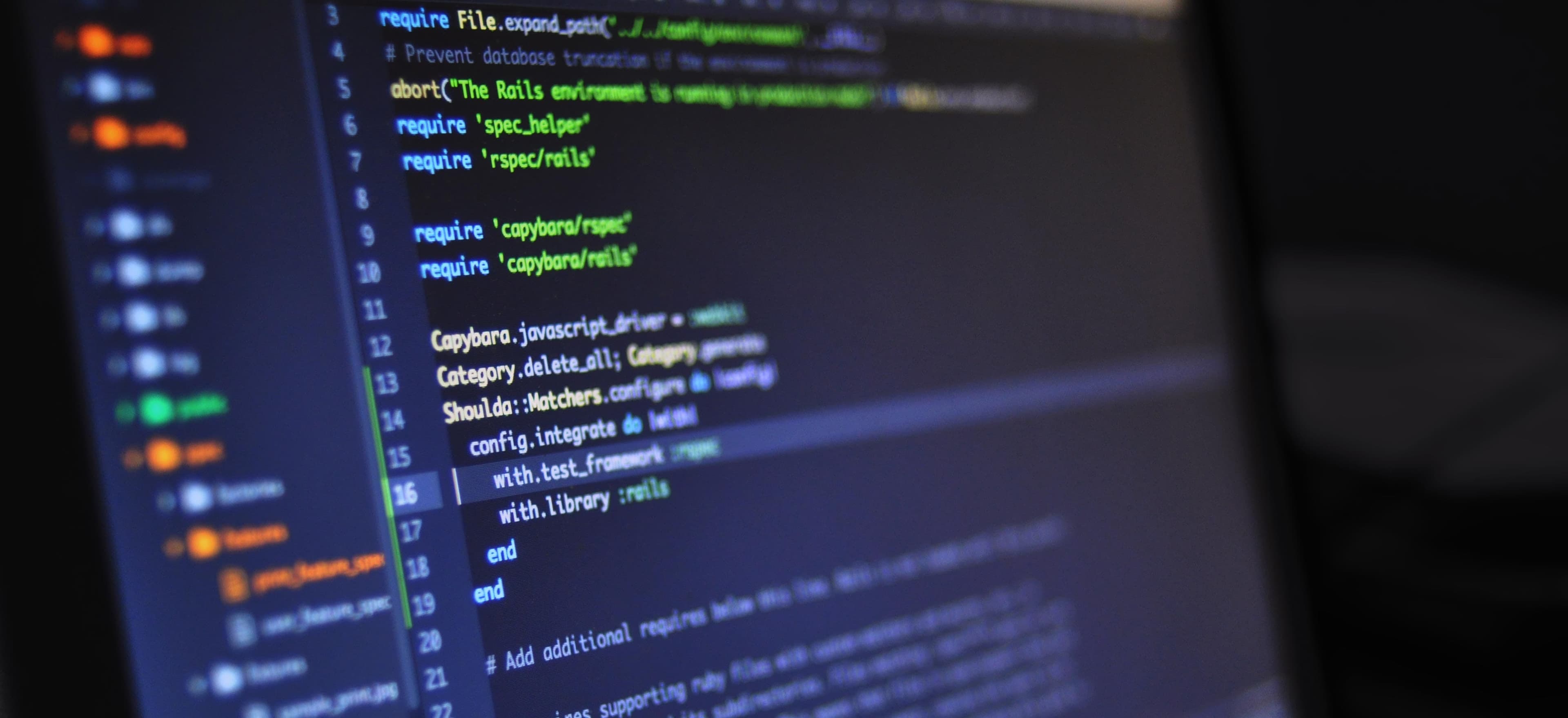
- Published on
When it comes to processing large-scale data, Apache Spark has become a ubiquitous tool due to its distributed computing capabilities. Java, as a powerhouse in the programming world, integrates seamlessly with Spark's Resilient Distributed Dataset (RDD) APIs, providing developers with the ability to leverage the power of functional programming through Java Streams.
Why Use Java Streams with Apache Spark RDDs?
Java Streams enable developers to write concise, readable, and expressive code for transforming collections of data. By using Java Streams in conjunction with Apache Spark RDDs, developers can apply this functional programming approach to the distributed datasets ingested and processed by Spark, resulting in more maintainable and understandable code.
Getting Started
Before delving into the integration of Java Streams with Apache Spark RDDs, it's important to set up the necessary dependencies. Ensure that the Apache Spark libraries are included in your Java project, and that you have a running Spark cluster or a local Spark installation for testing purposes. You can also use the Maven or Gradle build systems to include the Spark dependencies in your project.
Creating an RDD in Apache Spark
To illustrate the usage of Java Streams with Apache Spark RDDs, let's consider the simple task of calculating the sum of squares for a collection of integers using Spark. First, we'll create an RDD from a collection of integers:
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import java.util.Arrays;
public class JavaSparkIntegration {
public static void main(String[] args) {
SparkConf conf = new SparkConf().setAppName("JavaSparkIntegration").setMaster("local");
JavaSparkContext sc = new JavaSparkContext(conf);
JavaRDD<Integer> numbersRDD = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5));
}
}
In this example, we create an RDD numbersRDD
by parallelizing a collection of integers using JavaSparkContext
. This sets the stage for applying Java Streams operations to the RDD.
Applying Java Streams Operations
With the RDD created, we can apply Java Streams operations to transform and manipulate the data within the RDD. For instance, let's map each integer to its square, filter out the even squares, and then calculate their sum. The equivalent Java Streams operations for these transformations can be applied to the RDD as follows:
int sumOfEvenSquares = numbersRDD
.map(num -> num * num) // Map each number to its square
.filter(square -> square % 2 == 0) // Filter out the even squares
.reduce((x, y) -> x + y); // Calculate the sum of the even squares
In this code snippet, we use the map
, filter
, and reduce
operations to transform the RDD data just like we would with Java Streams on a local collection. This showcases the seamless integration of Java Streams operations with Apache Spark RDDs, allowing for clear and concise data processing logic.
Advantages of Using Java Streams with Apache Spark RDDs
-
Readability: Java Streams enable a more declarative and readable approach to data processing, facilitating easier comprehension of the data transformation logic.
-
Conciseness: By leveraging Java Streams operations, the code becomes more concise and expressive, reducing the verbosity often associated with traditional iterative approaches.
-
Functional Paradigm: Java Streams embrace the functional programming paradigm, allowing developers to focus on the "what" rather than the "how" of data transformations.
In Conclusion, Here is What Matters
Integrating Java Streams with Apache Spark RDDs empowers developers to wield the powerful combination of functional programming and distributed computing for large-scale data processing. This integration leads to code that is not only more expressive and concise but also more maintainable and comprehensible.
By adopting Java Streams in the context of Apache Spark, developers can harness the transformative potential of functional programming paradigms while leveraging the distributed computing capabilities of Spark RDDs.
In conclusion, the utilization of Java Streams enhances the prowess of Apache Spark, providing a clearer, more efficient, and more maintainable approach to distributed data processing. Its synergy with Spark RDDs demonstrates the potential for streamlining complex data transformations in a distributed computing environment.