Optimizing ADF Faces ClientComponent Attributes
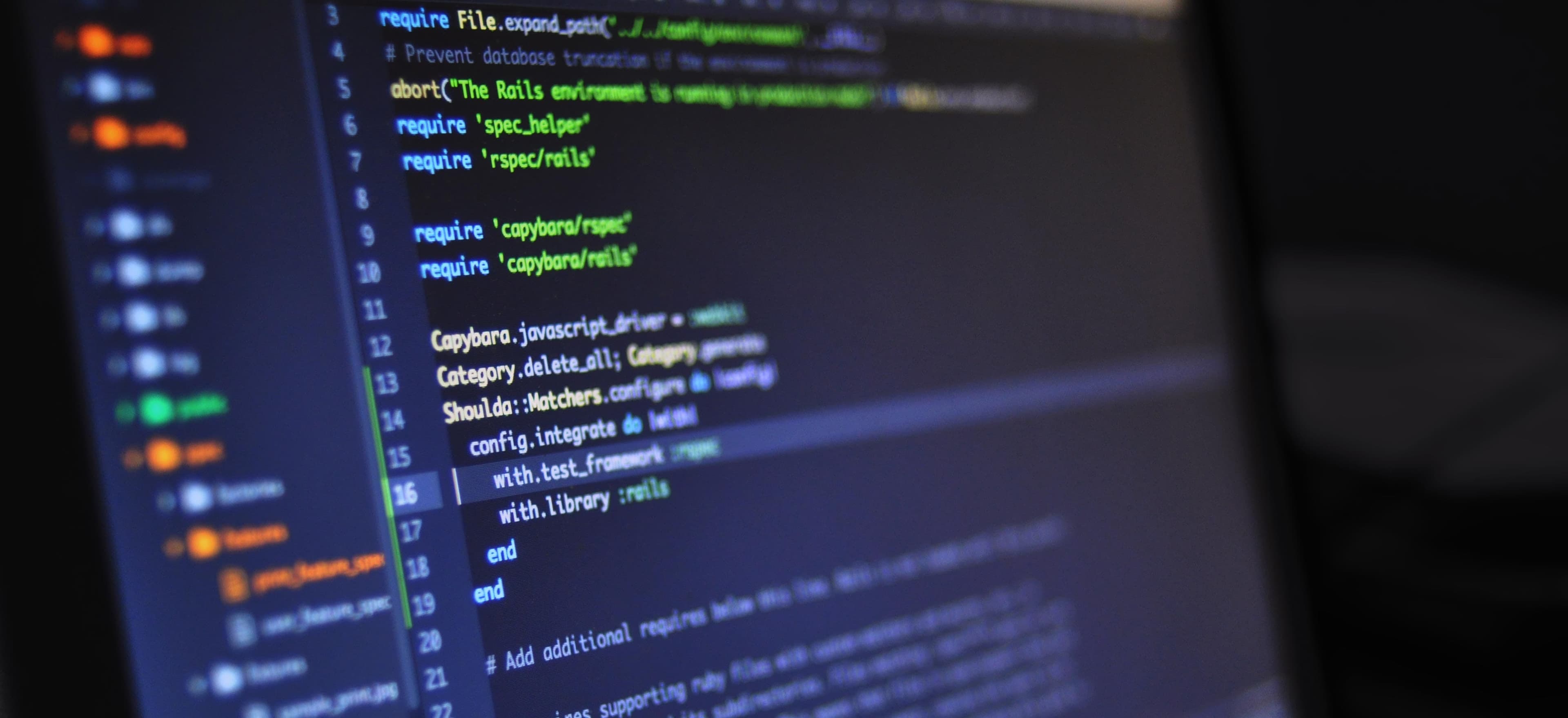
- Published on
Optimizing ADF Faces ClientComponent Attributes
When it comes to developing web applications, optimizing the client-side performance is crucial for delivering a seamless user experience. In Oracle ADF (Application Development Framework) Faces, the ClientComponent class provides a way to optimize the attributes that are rendered on the client side. By understanding and leveraging the capabilities of ClientComponent attributes, developers can significantly enhance the performance of ADF Faces applications.
Understanding ClientComponent Attributes
The ClientComponent class in ADF Faces allows developers to specify which component attributes should be sent to the client. By default, all attributes of ADF Faces components are transmitted to the client, which can result in unnecessary overhead. With the ClientComponent class, developers have the ability to include only the essential attributes that are required for client-side rendering and functionality.
How to Optimize ClientComponent Attributes
Optimizing ClientComponent attributes involves identifying the specific attributes that need to be transmitted to the client and excluding the rest. This process can be achieved by extending the ClientComponent class and overriding the getClientComponentAttributes
method.
Here's an example of how to optimize ClientComponent attributes for an ADF Faces component:
import org.apache.myfaces.trinidad.component.UIXInput;
import org.apache.myfaces.trinidad.component.ClientComponent;
public class CustomInputText extends UIXInput implements ClientComponent {
@Override
public String getClientComponentType(boolean b) {
return "CustomInputText";
}
@Override
protected String getClientComponentAttributesScript() {
// Include only essential attributes
StringBuilder script = new StringBuilder();
script.append(super.getClientComponentAttributesScript());
script.append(",placeholder: this.placeholder");
return script.toString();
}
}
In this example, the CustomInputText
class extends UIXInput
and implements ClientComponent
. The getClientComponentAttributesScript
method is overridden to specify the essential attributes that need to be sent to the client. By doing so, unnecessary attributes are excluded, resulting in a more optimized client-side rendering.
Why Optimize ClientComponent Attributes
Optimizing ClientComponent attributes offers several benefits:
-
Improved Performance: By excluding unnecessary attributes, the payload sent to the client is reduced, leading to faster rendering and improved performance.
-
Reduced Network Traffic: Transmitting only essential attributes minimizes the amount of data sent over the network, resulting in reduced bandwidth usage.
-
Enhanced Security: Limiting the client-side exposure of attributes reduces the risk of exposing sensitive information or implementation details.
-
Customization and Extensibility: By extending the ClientComponent class, developers have the flexibility to tailor the attribute transmission based on specific requirements and optimize the application for better customization and extensibility.
Best Practices for Optimizing ClientComponent Attributes
When optimizing ClientComponent attributes in ADF Faces, it's important to follow best practices to ensure a successful implementation:
-
Identify Essential Attributes: Analyze the ADF Faces components used in the application and determine which attributes are essential for client-side functionality and rendering.
-
Customize as Needed: Extend the ClientComponent class for specific ADF Faces components and tailor the
getClientComponentAttributesScript
method to include only the required attributes. -
Testing and Validation: Thoroughly test the optimized ClientComponent attributes to ensure that the functionality and rendering remain intact while eliminating unnecessary attributes.
-
Performance Monitoring: Continuously monitor the client-side performance to measure the impact of optimized attributes and make further adjustments if necessary.
-
Documentation and Communication: Document the optimization process and communicate the benefits to the development team to ensure consistency and understanding of the approach.
Lessons Learned
Optimizing ADF Faces ClientComponent attributes is an effective strategy for improving the client-side performance of web applications. By selectively transmitting essential attributes to the client, developers can reduce overhead, enhance security, and deliver a more responsive user experience. Leveraging the capabilities of the ClientComponent class in ADF Faces empowers developers to optimize attribute transmission and elevate the performance of their applications.
Incorporating optimal practices for ClientComponent attribute optimization can lead to significant improvements in the overall efficiency of ADF Faces applications, ultimately resulting in enhanced user satisfaction and a competitive edge in the ever-evolving web development landscape.
For further exploration on Oracle ADF Faces, check out the official documentation. Additionally, understanding the fundamental concepts of JavaScript optimization can complement the efforts of optimizing client-side attributes in ADF Faces applications.