Implementing Weather API in Android App
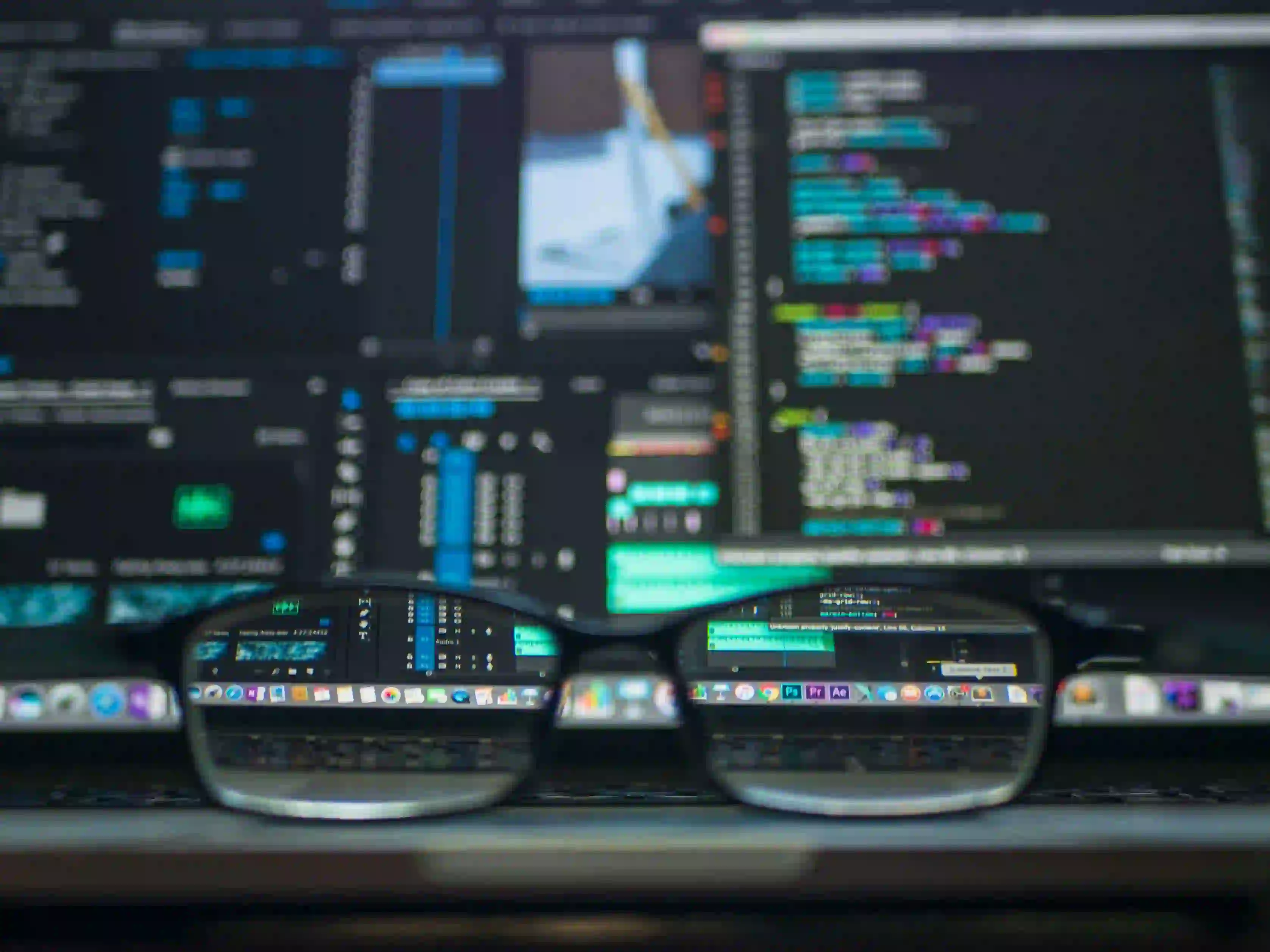
Integrating Weather API in Your Android App
Weather plays a crucial role in people’s daily lives, and integrating a weather API into your Android app can provide users with real-time weather updates, forecasts, and other essential information. In this blog post, we'll explore how to integrate a weather API into an Android app using Java. We'll focus on utilizing the OpenWeatherMap API, a popular choice for weather data.
Getting Started with OpenWeatherMap API
To get started, you'll need to sign up for a free account on the OpenWeatherMap website, which will provide you with an API key. This key will be essential for making requests to the OpenWeatherMap API and retrieving weather data for your app.
Making API Requests
In your Android app, you can use the HttpURLConnection
class to make API requests. Here's a simple example of making a GET request to the OpenWeatherMap API to fetch weather data for a specific city:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class WeatherApiClient {
private static final String API_KEY = "YOUR_API_KEY";
private static final String API_URL = "https://api.openweathermap.org/data/2.5/weather?q=London&appid=" + API_KEY;
public String getWeatherData() {
HttpURLConnection connection = null;
InputStream inputStream = null;
try {
URL url = new URL(API_URL);
connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
inputStream = connection.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
StringBuilder result = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
result.append(line);
}
return result.toString();
} catch (IOException e) {
e.printStackTrace();
return null;
} finally {
if (connection != null) {
connection.disconnect();
}
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
In the above code, we create a WeatherApiClient
class with a getWeatherData
method to fetch weather data for a specific city (in this case, "London"). We construct the API URL with the city name and our API key, make a GET request, and read the response.
Parsing API Response
Once you've made a request and received the weather data from the API, you'll need to parse the JSON response to extract the relevant information such as current temperature, humidity, and weather conditions. Here's an example of how you can parse the JSON response in your Android app:
import org.json.JSONObject;
import org.json.JSONException;
public class WeatherDataParser {
public static void parseWeatherData(String json) {
try {
JSONObject jsonResponse = new JSONObject(json);
JSONObject main = jsonResponse.getJSONObject("main");
double temperature = main.getDouble("temp");
int humidity = main.getInt("humidity");
JSONObject weather = jsonResponse.getJSONArray("weather").getJSONObject(0);
String description = weather.getString("description");
// Use the parsed data in your app
// ...
} catch (JSONException e) {
e.printStackTrace();
}
}
}
In this code snippet, we use the JSONObject
class from the org.json
package to parse the JSON response and extract the temperature, humidity, and weather description from the API. You can then use these parsed values to display weather information in your app's user interface.
Enhancing User Experience
Integrating weather data can greatly enhance the user experience of your Android app. You can display current weather conditions, forecasts, and other relevant information using custom UI components and visualizations. Additionally, you can use the user's location to provide localized weather information.
Wrapping Up
In this post, we've learned how to integrate a weather API into an Android app using Java. We made API requests to fetch weather data, parsed the JSON responses to extract relevant information, and discussed ways to enhance the user experience. By incorporating real-time weather updates, your app can provide valuable and timely information to its users.
Integrating a weather API can be a powerful addition to your app, bringing real-world data and relevant functionality to your users. Whether it's for planning outdoor activities, travel, or simply staying informed, weather data plays a significant role in many people's lives. By following the steps outlined in this post, you can effectively integrate weather information into your Android app, offering users a more comprehensive and engaging experience.
Give it a try and see how integrating weather data can elevate your app's utility and relevance to users!