Optimizing REST API Performance for JQGrid in Spring MVC
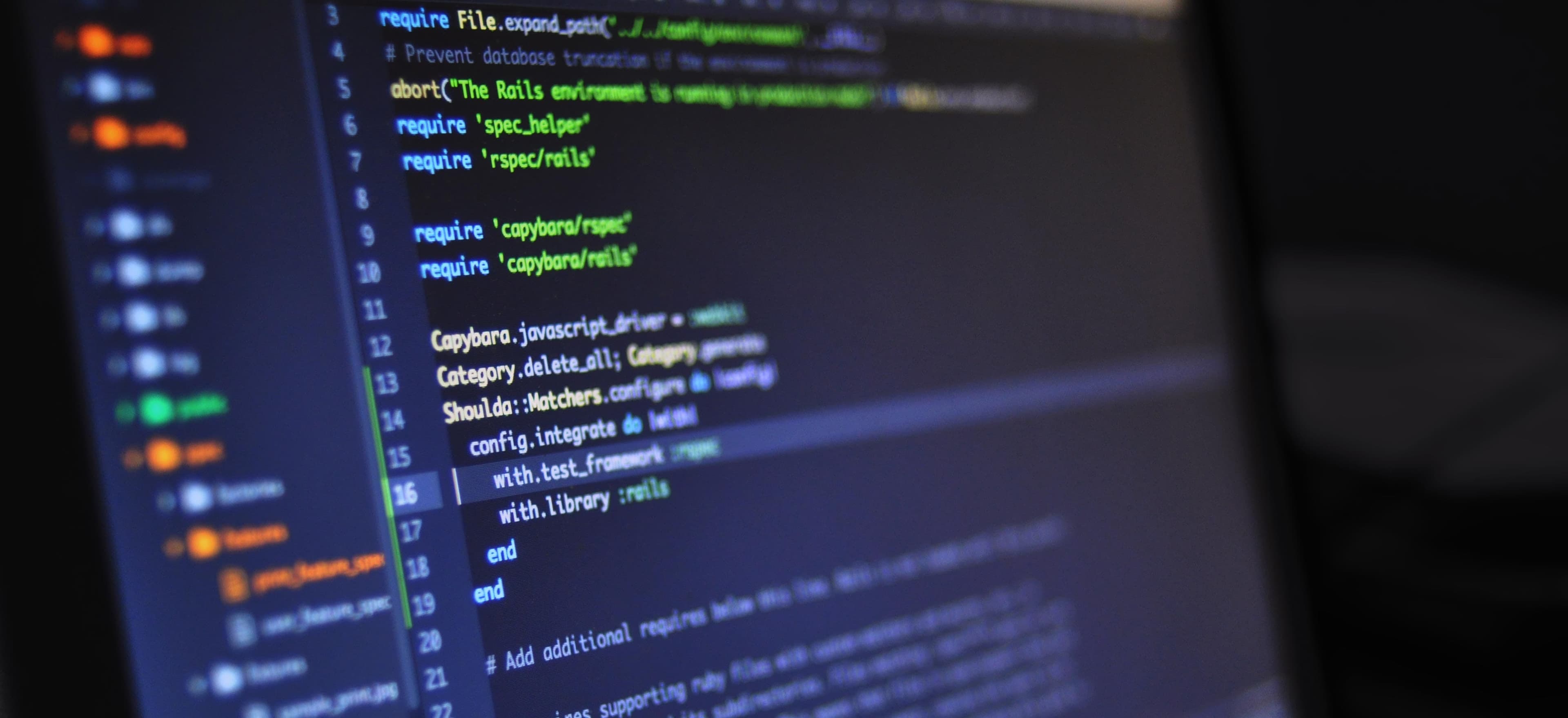
- Published on
Optimizing REST API Performance for JQGrid in Spring MVC
When building a web application using Java and Spring MVC, it's crucial to ensure the performance of the REST APIs that communicate with the frontend. In this blog post, we'll focus on optimizing the performance of REST APIs for JQGrid, a popular jQuery plugin for displaying and interacting with tabular data. We'll explore various techniques and best practices to enhance the performance of your Spring MVC application when working with JQGrid.
Understanding JQGrid and REST APIs
JQGrid is a jQuery plugin that provides an easy-to-use grid system for displaying and manipulating tabular data. When using JQGrid in a web application, the frontend interacts with the backend through REST APIs to fetch, update, and delete data. This communication between the frontend and backend is vital for the overall performance and responsiveness of the application.
Optimizing REST APIs for JQGrid
1. Implementing Pagination and Sorting
When dealing with a large dataset in JQGrid, fetching and displaying all the data at once can significantly impact performance. Implementing pagination and sorting in your REST APIs allows the frontend to request data in smaller, manageable chunks, reducing the load on the server and improving the user experience.
In your Spring MVC controller, you can utilize the Pageable
interface along with Spring Data's repositories to easily implement pagination and sorting for your REST endpoints.
@GetMapping("/data")
public ResponseEntity<Page<Data>> getData(Pageable pageable) {
Page<Data> data = dataRepository.findAll(pageable);
return ResponseEntity.ok(data);
}
2. Utilizing Response Caching
Another effective way to enhance the performance of your REST APIs is by leveraging response caching. By caching the server's responses at the client or intermediary proxies, you can reduce the number of redundant requests to the server, thereby improving the overall responsiveness of the application.
In Spring MVC, you can use the @Cacheable
annotation from the Spring Framework to cache the responses of your REST endpoints. This annotation supports various caching providers, such as Ehcache, Redis, or Caffeine, allowing you to choose the one that best suits your application's requirements.
@Cacheable("dataCache")
@GetMapping("/data/{id}")
public ResponseEntity<Data> getDataById(@PathVariable Long id) {
// Fetch and return data by id
}
3. Employing Proper Data Transfer Objects (DTOs)
When designing your REST APIs for JQGrid, it's important to use proper Data Transfer Objects (DTOs) to efficiently transfer data between the frontend and backend. DTOs allow you to tailor the data exchanged with the frontend specifically for the requirements of JQGrid, minimizing unnecessary data transfer and improving the API's performance.
By creating DTOs that only include the fields required by JQGrid and using them for data exchange, you can optimize the payload size of the API responses and reduce the processing overhead on both the server and client sides.
4. Applying Compression for Data Transmission
Compressing the data transmitted between the server and the client can significantly improve the performance of your REST APIs. By reducing the size of the payload, compression techniques such as GZIP or DEFLATE can minimize network latency and bandwidth usage, leading to faster data transfers and improved overall responsiveness.
In a Spring MVC application, you can enable response compression by configuring the HttpComponentsMessageSender
with a GzipRequestCustomizer
for your REST template. This allows the server to compress the HTTP responses before sending them to the client, optimizing data transmission for improved performance.
RestTemplate restTemplate = new RestTemplate();
restTemplate.setRequestFactory(new HttpComponentsClientHttpRequestFactory(
HttpClientBuilder.create()
.addInterceptorFirst(new HttpRequestInterceptor() {
public void process(HttpRequest request, HttpContext context)
throws HttpException, IOException {
request.addHeader("Accept-Encoding", "gzip");
}
}).build()));
5. Implementing Efficient Error Handling
Proper error handling is crucial for the performance and user experience of your REST APIs. Instead of returning verbose and unstructured error messages, strive to implement efficient error handling that provides clear and concise information to the frontend, enabling the client to gracefully handle error conditions without unnecessary performance overhead.
By utilizing HTTP status codes along with meaningful error messages in your API responses, you can streamline error communication between the frontend and backend, promoting efficient error handling without compromising performance.
Lessons Learned
Optimizing the performance of your REST APIs for JQGrid in a Spring MVC application is essential for delivering a responsive and efficient user experience. By implementing pagination, response caching, efficient DTOs, data compression, and robust error handling, you can significantly enhance the performance of your application while seamlessly integrating with JQGrid.
Adhering to these best practices not only ensures the optimal performance of your REST APIs but also contributes to the overall success of your Java web application. By continuously evaluating and refining the performance optimizations, you can maintain a high-performing and reliable backend for your JQGrid-powered frontend.
Incorporating these strategies will undoubtedly elevate the responsiveness and efficiency of your Spring MVC application when interacting with JQGrid, providing a seamless user experience while handling large datasets with ease.