Overcoming Challenges: Real Device Testing
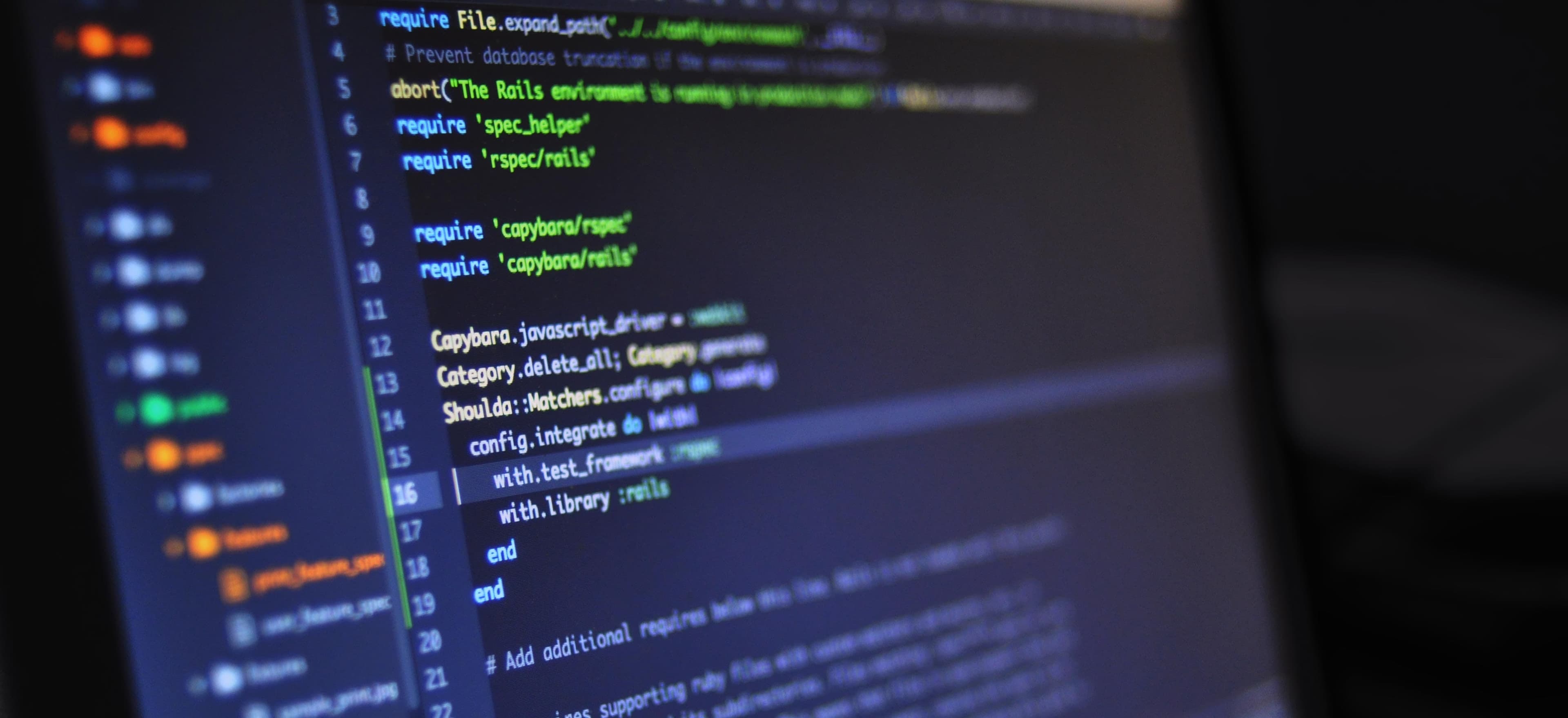
- Published on
Overcoming Challenges: Real Device Testing
Software testing is an integral part of the software development cycle. However, testing on real devices can present specific challenges which need to be addressed for successful testing and deployment. In this blog post, we will explore the importance of real device testing, the challenges it presents, and how to overcome them using Java.
The Importance of Real Device Testing
Real device testing is crucial for ensuring that an application behaves as expected across a wide range of devices, operating systems, and network conditions. Unlike testing on emulators or simulators, real device testing provides accurate insights into how an application performs in real-world scenarios.
By testing on real devices, developers can uncover device-specific issues such as performance bottlenecks, UI inconsistencies, and hardware compatibility issues that may not be apparent in simulated environments.
Challenges of Real Device Testing
While real device testing offers valuable insights, it comes with its own set of challenges. Some of the common challenges include:
-
Device Fragmentation: The Android ecosystem, for example, consists of a wide variety of devices with different screen sizes, resolutions, and hardware capabilities. Testing on all possible combinations is impractical.
-
Operating System Fragmentation: Similarly, there are multiple versions of operating systems in the market, each with its own set of features and limitations. Ensuring compatibility across these versions is a substantial challenge.
-
Network Variability: Real-world network conditions can significantly impact the performance and behavior of an application. Testing under different network conditions is essential for identifying and addressing potential issues.
Overcoming Challenges with Java
Java provides several tools and frameworks that can help in overcoming the challenges of real device testing.
1. Appium for Cross-Platform Testing
Appium is an open-source automation tool for testing mobile and web applications. It allows you to write tests using your preferred programming language, including Java, and run them on both emulators/simulators and real devices. Appium's cross-platform capabilities make it invaluable for testing across a wide range of devices and operating systems.
// Example of using Appium with Java for real device testing
import io.appium.java_client.AppiumDriver;
import io.appium.java_client.MobileElement;
import io.appium.java_client.android.AndroidDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import java.net.URL;
public class AppiumRealDeviceTest {
public static void main(String[] args) {
// Set up desired capabilities
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("deviceName", "Android Device");
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("appPackage", "your.app.package");
// Initialize the driver
AppiumDriver<MobileElement> driver = new AndroidDriver<>(new URL("http://127.0.0.1:4723/wd/hub"), capabilities);
// Write your test code here
// ...
// Close the driver
driver.quit();
}
}
2. Espresso for Android UI Testing
For Android-specific real device testing, Espresso is a powerful testing framework provided by Google. Espresso is designed to make UI testing more concise and reliable, especially when testing on real devices with different screen sizes and resolutions.
// Example of using Espresso for UI testing in Java
import androidx.test.ext.junit.rules.ActivityScenarioRule;
import static androidx.test.espresso.Espresso.onView;
import static androidx.test.espresso.action.ViewActions.click;
import static androidx.test.espresso.matcher.ViewMatchers.withId;
import org.junit.Rule;
import org.junit.Test;
import your.app.package.MainActivity;
public class EspressoRealDeviceTest {
@Rule
public ActivityScenarioRule<MainActivity> activityTestRule = new ActivityScenarioRule<>(MainActivity.class);
@Test
public void testButtonClick() {
// Perform a button click and assert the result
onView(withId(R.id.button)).perform(click());
// Add assertions here
}
}
3. Sikuli for Visual Testing
In scenarios where visual elements need to be tested on real devices, Sikuli can be a valuable tool. Sikuli allows you to automate visual workflows by using screenshots and image recognition, making it suitable for verifying UI elements across different devices and resolutions.
// Example of using Sikuli with Java for visual testing
import org.sikuli.script.FindFailed;
import org.sikuli.script.Pattern;
import org.sikuli.script.Screen;
public class SikuliVisualTest {
public static void main(String[] args) {
Screen screen = new Screen();
Pattern image = new Pattern("image.png");
try {
// Find and click the image on the screen
screen.click(image);
// Add assertions here
} catch (FindFailed e) {
e.printStackTrace();
}
}
}
4. Network Simulation with Traffic Parrot
Simulating real-world network conditions for testing can be achieved using tools like Traffic Parrot. Traffic Parrot allows you to emulate various network conditions such as latency, packet loss, and bandwidth limitations, enabling comprehensive testing of network-dependent applications.
// Example of using Traffic Parrot for network simulation in Java
import com.trafficparrot.traffic.Traffic;
public class TrafficParrotTest {
public static void main(String[] args) {
Traffic traffic = new Traffic.Builder()
.latency(100) // Set latency to 100ms
.packetLoss(0.2) // Simulate 20% packet loss
.build();
// Apply the network conditions to your test scenario
traffic.apply();
// Write your network-dependent test code here
// ...
// Reset the network conditions
traffic.reset();
}
}
Closing Remarks
Real device testing is essential for delivering a robust and reliable application that performs well across various devices and operating conditions. By leveraging the tools and frameworks available in Java, developers can overcome the challenges of real device testing and ensure high-quality software delivery.
In conclusion, while real device testing presents its own set of challenges, Java provides a rich ecosystem of tools and frameworks that empower developers to tackle these challenges effectively. Embracing real device testing with Java enables developers to deliver high-quality software that meets the diverse demands of the modern digital landscape.