Streamlining Text Search in Batch Files
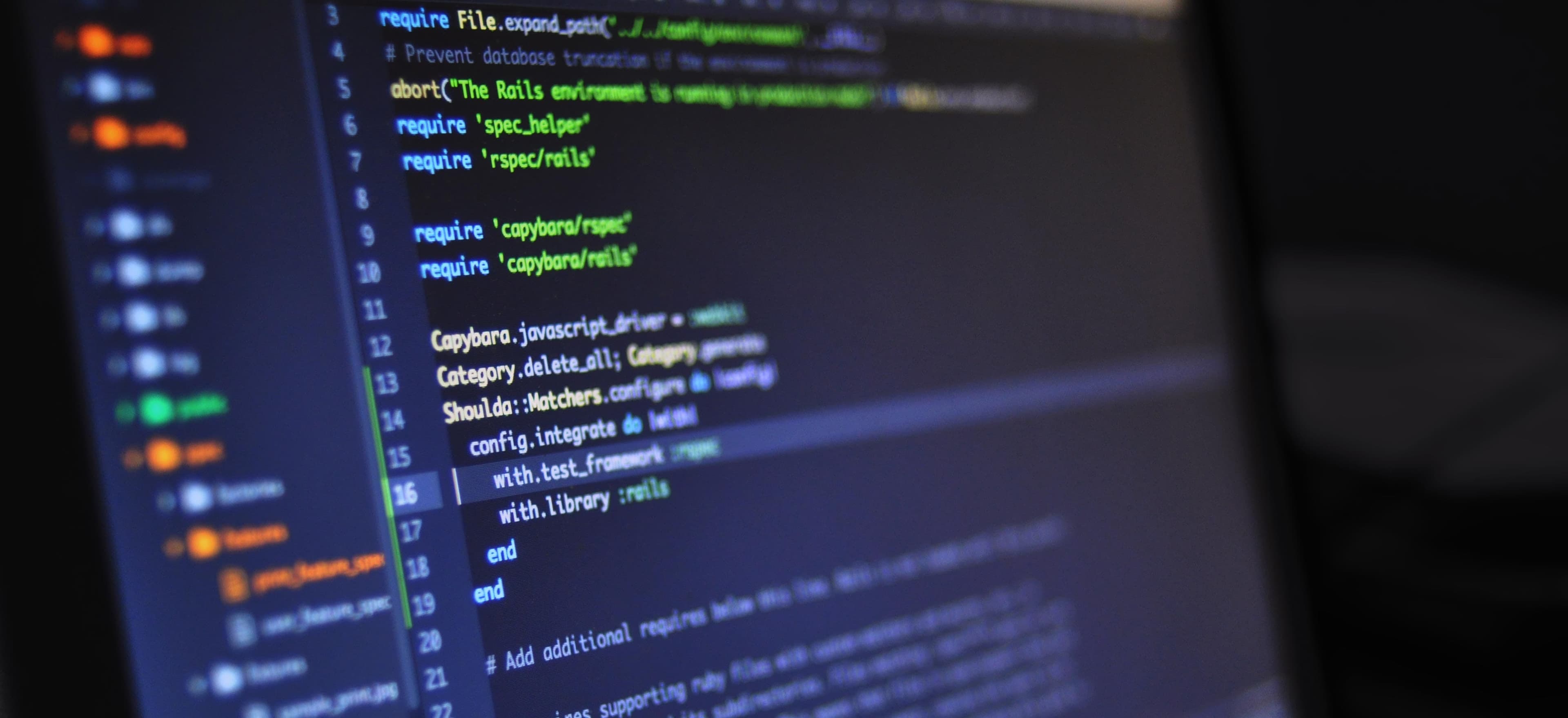
- Published on
Making Text Search Efficient with Java
Searching for specific text patterns within a file or a batch of files is a common operation in programming. While there are various ways to approach this task, using Java can offer a powerful and efficient solution. In this article, we'll explore how to streamline text search in batch files using Java, optimizing the process for both performance and simplicity.
Understanding the Problem
When dealing with a large number of files, traditional text search algorithms can become inefficient. The overhead of repeatedly opening and scanning each file can lead to performance bottlenecks, especially when processing a significant volume of data.
To address this challenge, we can leverage Java's input/output capabilities, combined with its powerful string manipulation functions, to streamline the text search process.
Leveraging Java's File I/O
Java provides a rich set of tools for working with files. When handling batch text search, we can use the java.nio.file
package to efficiently traverse directories and access the contents of files.
Let's start by examining how we can traverse a directory and its subdirectories to locate all the files that we want to search. Below is an example of how this can be achieved in Java:
import java.io.IOException;
import java.nio.file.*;
import java.util.stream.Stream;
public class FileSearch {
public static void main(String[] args) {
Path directory = Paths.get("path_to_directory");
try (Stream<Path> paths = Files.walk(directory)) {
paths.filter(Files::isRegularFile)
.forEach(FileSearch::searchInFile);
} catch (IOException e) {
e.printStackTrace();
}
}
private static void searchInFile(Path file) {
// Perform text search within the file
// Your text search logic goes here
}
}
In this example, we use the Files.walk
method to traverse the directory and its subdirectories, filtering out only the regular files. For each file found, we then invoke the searchInFile
method to perform the text search.
Efficient Text Search Algorithm
To optimize the text search process, we need to implement a robust and efficient algorithm for locating the desired text patterns within each file. One popular approach is to use the Knuth-Morris-Pratt (KMP) algorithm, known for its fast and efficient string matching capabilities.
The KMP algorithm is particularly well-suited for batch text search, as it offers linear time complexity for pattern matching, making it highly efficient for processing large files or a significant number of files.
Below is an illustrative implementation of the KMP algorithm for text search in Java:
public class KMPTextSearch {
public static void main(String[] args) {
String text = "sample_text_to_search_within";
String pattern = "search_pattern";
int index = search(text, pattern);
if (index != -1) {
System.out.println("Pattern found at index " + index);
} else {
System.out.println("Pattern not found");
}
}
private static int search(String text, String pattern) {
int[] lps = calculateLPSArray(pattern);
int i = 0, j = 0;
while (i < text.length()) {
if (text.charAt(i) == pattern.charAt(j)) {
i++;
j++;
}
if (j == pattern.length()) {
return i - j;
} else if (i < text.length() && text.charAt(i) != pattern.charAt(j)) {
if (j != 0) {
j = lps[j - 1];
} else {
i++;
}
}
}
return -1;
}
private static int[] calculateLPSArray(String pattern) {
int[] lps = new int[pattern.length()];
int len = 0;
int i = 1;
lps[0] = 0;
while (i < pattern.length()) {
if (pattern.charAt(i) == pattern.charAt(len)) {
len++;
lps[i] = len;
i++;
} else {
if (len != 0) {
len = lps[len - 1];
} else {
lps[i] = 0;
i++;
}
}
}
return lps;
}
}
In this example, the search
method implements the KMP algorithm to efficiently locate the pattern within the text. The algorithm utilizes the concept of the Longest Prefix which is also a Suffix (LPS) array to efficiently skip unnecessary character comparisons, optimizing the search process.
Final Considerations
Efficiently searching for text patterns within a batch of files is vital for numerous applications, such as log analysis, data mining, and content indexing. By leveraging the robust file I/O capabilities and powerful string manipulation functions that Java offers, along with implementing an efficient text search algorithm like the Knuth-Morris-Pratt algorithm, we can streamline the text search process, making it both performant and scalable.
Java provides a solid foundation for addressing text search challenges in batch files, empowering developers to build reliable and high-performance solutions for their text processing needs.
By following the principles outlined in this article, you can create efficient and streamlined text search processes within your Java applications, contributing to improved performance and a better overall user experience.
Incorporating these techniques into your coding repertoire will not only enhance your software development skills but also enable you to deliver more effective and efficient solutions to the text search challenges you encounter.
In conclusion, mastering text search optimization in Java is a key aspect of software development, and by applying the techniques outlined in this article, you're well-equipped to tackle the most demanding text search requirements with confidence and efficiency.
Remember, efficient text search is not just about finding what you're looking for; it's about doing so in a way that is fast, scalable, and robust, and Java provides the tools and techniques to achieve just that.
So, go ahead, put these principles into practice, and take your text search capabilities to the next level with Java!
Start implementing these Java-driven text search optimizations in your projects, and witness the enhanced efficiency and performance they bring to your text processing tasks. Happy coding!
By implementing these practices and optimizing text search in Java, you can significantly enhance the efficiency and performance of your applications, ensuring a smoother and more responsive user experience.