Optimizing Operations with Automated ETL Workflows
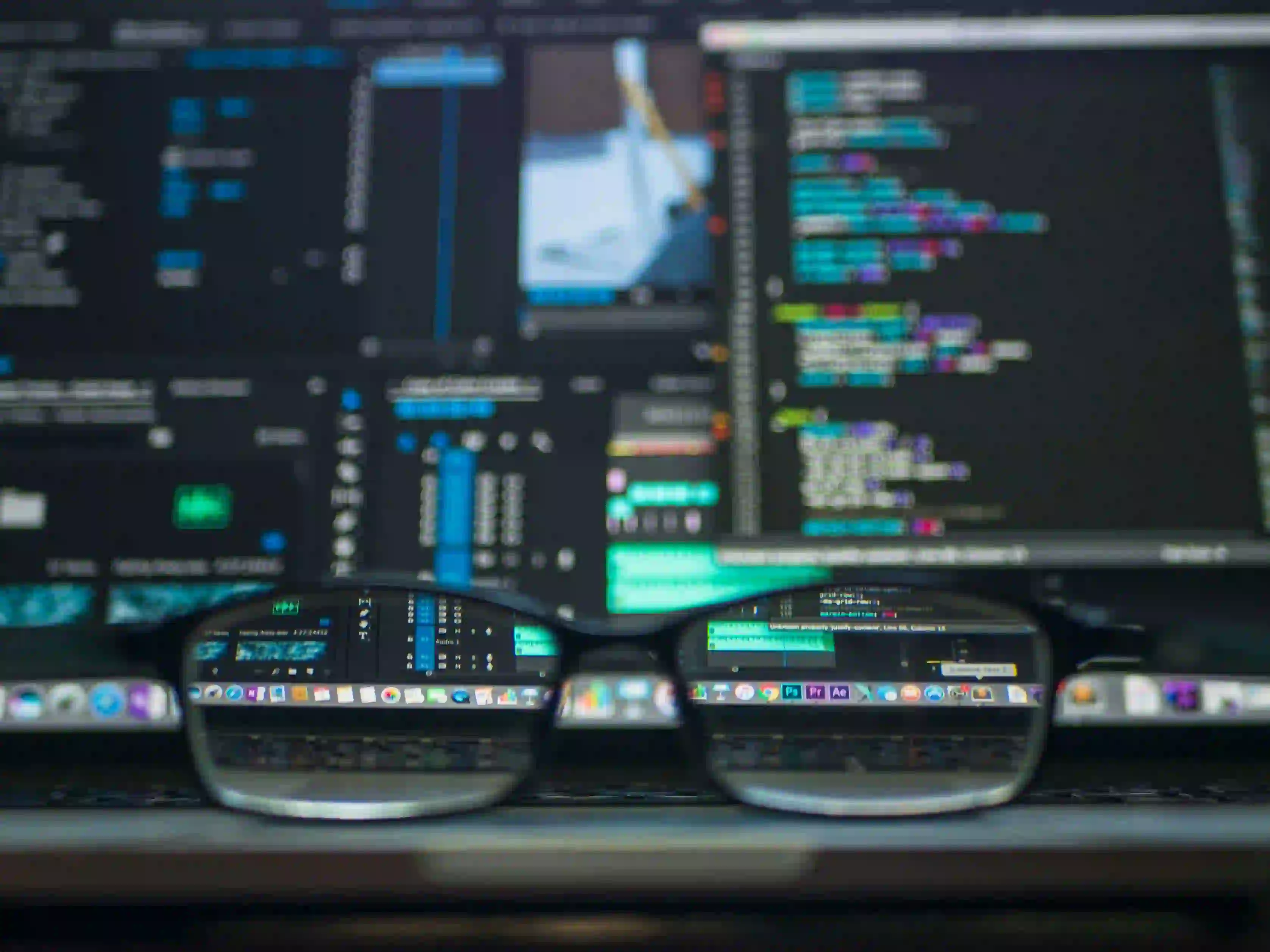
Optimizing Operations with Automated ETL Workflows
In today's data-driven world, businesses rely on efficient data pipelines to extract, transform, and load (ETL) data from various sources into their analytics and reporting systems. Java, as a versatile programming language, offers powerful tools and frameworks to streamline ETL processes. In this article, we'll explore how to optimize operations with automated ETL workflows using Java.
Why Automated ETL Workflows Matter
Before delving into the technical aspects, let's understand why automated ETL workflows are crucial for modern businesses. Traditional ETL processes often involve manual intervention, leading to a higher risk of errors, longer processing times, and increased operational costs. By automating ETL workflows, organizations can achieve the following benefits:
- Faster Data Processing: Automation reduces the time taken to process large volumes of data, enabling real-time or near-real-time analytics.
- Improved Accuracy: Automated workflows minimize human errors, leading to more reliable data for decision-making.
- Cost Efficiency: By reducing manual effort, businesses can lower operational costs and allocate resources to more strategic initiatives.
With the importance of automated ETL workflows established, let's dive into how Java can facilitate this optimization.
Leveraging Java for Automated ETL Workflows
Java provides a wealth of libraries, frameworks, and tools that empower developers to build robust and scalable ETL workflows. Below are some key elements of Java's ecosystem that contribute to efficient ETL operations:
1. Apache NiFi for Data Flow Management
Apache NiFi is a powerful open-source tool for automating and managing the flow of data between systems. It provides a visual interface for designing data flows, making it an ideal choice for ETL workflow orchestration. Java developers can leverage NiFi's extensible architecture to create custom processors, enabling seamless integration with various data sources and destinations.
2. Spring Batch for Batch Processing
Spring Batch is a lightweight, comprehensive framework for building batch applications in Java. It simplifies the development of robust batch processes, including ETL jobs, through reusable components such as readers, processors, and writers. With Spring Batch, developers can define complex ETL workflows as a series of batch jobs, benefitting from features like transaction management and chunk-based processing.
3. Apache Spark for Big Data ETL
When dealing with large-scale data processing, Apache Spark stands out as a leading framework. Java developers can harness Spark's distributed computing capabilities to perform high-performance ETL operations on big data. By writing Spark applications in Java, teams can take advantage of Spark's rich APIs for data transformation, manipulation, and integration with various data sources.
Implementing Automated ETL Workflows in Java
Now that we've identified the tools and frameworks, let's walk through a simplified example of implementing an automated ETL workflow in Java. In this scenario, we'll use Spring Batch to orchestrate the ETL process, extracting data from a CSV file, transforming it, and loading it into a database.
Step 1: Define the Data Model
First, we need to define the data model for the input CSV file and the database table. Let's consider a simple Customer
entity with attributes for id
, name
, and email
.
public class Customer {
private Long id;
private String name;
private String email;
// Getters and setters
}
Step 2: Create ETL Components
Next, we'll create the ETL components using Spring Batch. This involves defining a reader to extract data from the CSV file, a processor to transform the data, and a writer to load it into the database.
@Configuration
@EnableBatchProcessing
public class ETLJobConfiguration {
@Autowired
private JobBuilderFactory jobBuilderFactory;
@Autowired
private StepBuilderFactory stepBuilderFactory;
@Bean
public FlatFileItemReader<Customer> customerItemReader() {
// Configure the reader for the CSV file
}
@Bean
public ItemProcessor<Customer, Customer> customerItemProcessor() {
// Implement data transformation logic
}
@Bean
public JdbcBatchItemWriter<Customer> customerItemWriter(DataSource dataSource) {
// Configure the writer to insert data into the database
}
@Bean
public Step etlStep() {
return stepBuilderFactory.get("etlStep")
.<Customer, Customer>chunk(100)
.reader(customerItemReader())
.processor(customerItemProcessor())
.writer(customerItemWriter())
.build();
}
@Bean
public Job etlJob() {
return jobBuilderFactory.get("etlJob")
.start(etlStep())
.build();
}
}
In this configuration, we define the components for reading, processing, and writing data within a batch job.
Step 3: Run the ETL Job
Once the ETL components are in place, we can run the job to trigger the automated ETL workflow.
public class MainApplication {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(ETLJobConfiguration.class);
JobLauncher jobLauncher = context.getBean(JobLauncher.class);
Job job = context.getBean(Job.class);
JobExecution jobExecution = jobLauncher.run(job, new JobParameters());
System.out.println("Job Status : " + jobExecution.getStatus());
}
}
By executing the main application, the ETL job will be triggered, automating the entire data workflow from extraction to loading.
Key Takeaways
Automated ETL workflows play a pivotal role in optimizing data operations, and Java offers a wealth of tools and frameworks to support this endeavor. By leveraging Apache NiFi, Spring Batch, Apache Spark, and other Java-based technologies, organizations can streamline their ETL processes for improved efficiency, accuracy, and cost savings. With the example and insights provided in this article, developers can embark on enhancing their data workflows through automated ETL with Java.