Effective Custom Exception Handling in JAX-RS
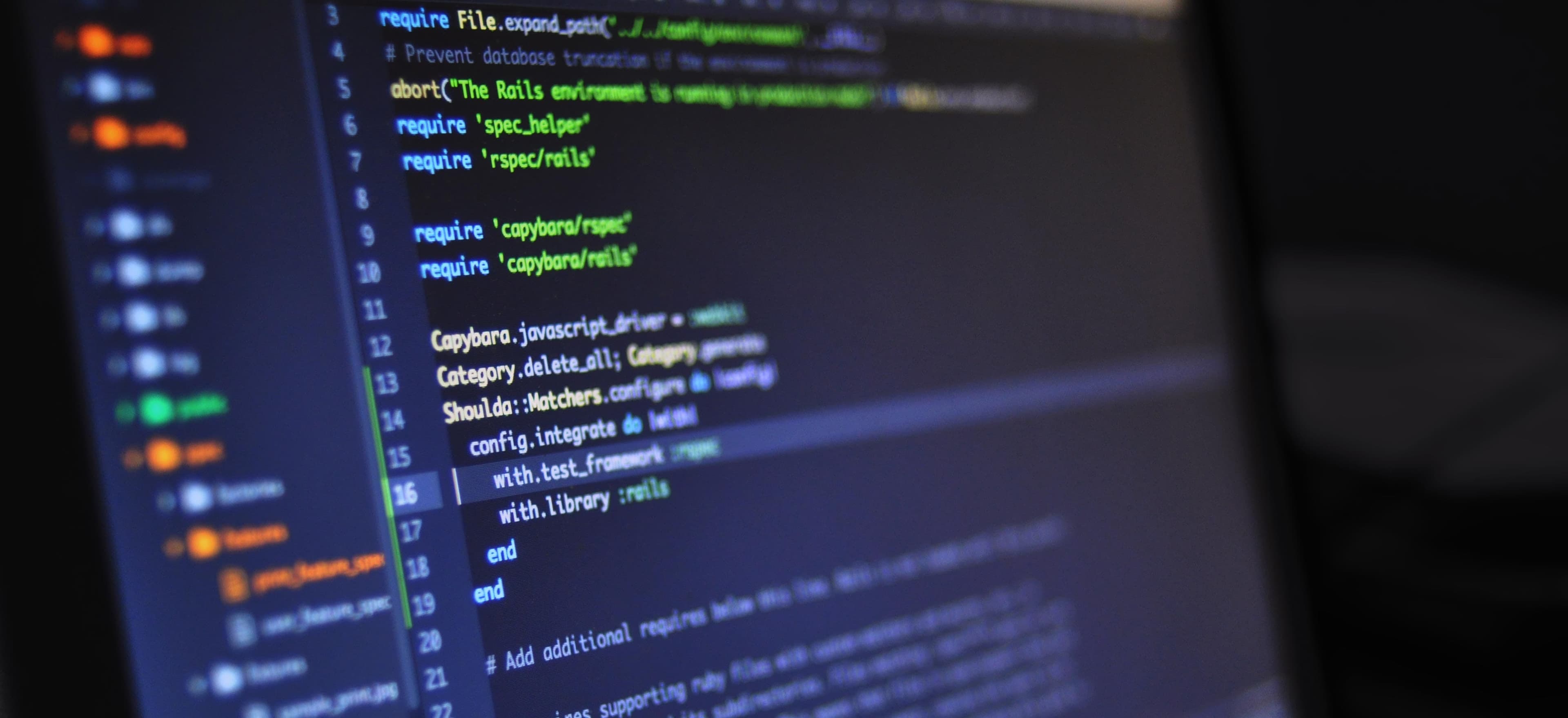
- Published on
Effective Custom Exception Handling in JAX-RS
In any Java application, handling exceptions effectively is crucial. When it comes to RESTful web services, JAX-RS provides a robust framework for building APIs. However, handling exceptions gracefully and returning appropriate error responses to clients is essential for a smooth user experience.
In this article, we'll explore how to implement custom exception handling in JAX-RS to provide meaningful error messages and HTTP status codes.
The Problem with Default Exception Handling
By default, when an exception occurs in a JAX-RS application, the framework will generate a standard error response with an HTTP 500 status code. While this is adequate for internal server errors, it doesn't provide specific details about the cause of the error, making it less than ideal for client consumption.
Creating Custom Exceptions
The first step in custom exception handling is to define specific exception classes that extend either WebApplicationException
or RuntimeException
. By creating custom exceptions, you can encapsulate detailed information about the error and tailor the response to suit your API’s requirements.
Let's consider a hypothetical scenario where we have a JAX-RS endpoint for retrieving a user by their ID. We want to throw a custom exception if the requested user ID is not found.
public class UserNotFoundException extends WebApplicationException {
public UserNotFoundException(String message) {
super(Response.status(Response.Status.NOT_FOUND)
.entity(message)
.type(MediaType.TEXT_PLAIN)
.build());
}
}
In the above code, we create a UserNotFoundException
class that extends WebApplicationException
and sets the response status to 404 (NOT FOUND). Additionally, we customize the error message and set the response type to plain text.
Exception Mapping with ExceptionMapper
To ensure these custom exceptions are caught and handled appropriately, we can utilize the ExceptionMapper
interface provided by JAX-RS. This interface allows us to map specific exception types to custom logic for generating error responses.
Let's create an ExceptionMapper
for our UserNotFoundException
:
@Provider
public class UserNotFoundExceptionMapper implements ExceptionMapper<UserNotFoundException> {
@Override
public Response toResponse(UserNotFoundException exception) {
return exception.getResponse();
}
}
In the above code, we have defined an ExceptionMapper
specifically for UserNotFoundException
. When this type of exception is thrown within our JAX-RS resource methods, this mapper will catch it and return the customized response provided by the exception.
Registering ExceptionMapper
with Application
To ensure that JAX-RS recognizes our custom ExceptionMapper
, we need to register it with the application. This can be done by adding it to the set of providers when configuring the JAX-RS application.
@ApplicationPath("/api")
public class MyApplication extends Application {
@Override
public Set<Object> getSingletons() {
Set<Object> singletons = new HashSet<>();
singletons.add(new UserNotFoundExceptionMapper());
return singletons;
}
}
Triggering Custom Exceptions
Now that we have our custom exception and mapper in place, let's see how we can trigger the UserNotFoundException
within a JAX-RS resource method.
@Path("/users")
@Produces(MediaType.APPLICATION_JSON)
public class UserResource {
@GET
@Path("/{id}")
public Response getUserById(@PathParam("id") String id) {
User user = userService.getUserById(id);
if (user == null) {
throw new UserNotFoundException("User with ID " + id + " not found");
}
return Response.ok(user).build();
}
}
In the above code, if the userService.getUserById(id)
method returns null
, indicating that the user with the specified ID doesn't exist, we throw our custom UserNotFoundException
. This triggers the UserNotFoundExceptionMapper
we defined earlier, resulting in a customized error response being sent to the client.
A Final Look
In conclusion, custom exception handling in JAX-RS empowers developers to deliver more meaningful error messages and appropriate HTTP status codes, enhancing the overall user experience when interacting with RESTful APIs.
By creating custom exceptions, mapping them to specific error responses using ExceptionMapper
, and registering those mappers with the JAX-RS application, developers can effectively manage error scenarios and communicate issues clearly to clients.
With the ability to provide detailed error information, clients can better understand and handle exceptional scenarios, leading to more resilient and user-friendly RESTful APIs.