Understanding the Impact of Garbage Collection on Java Application Performance
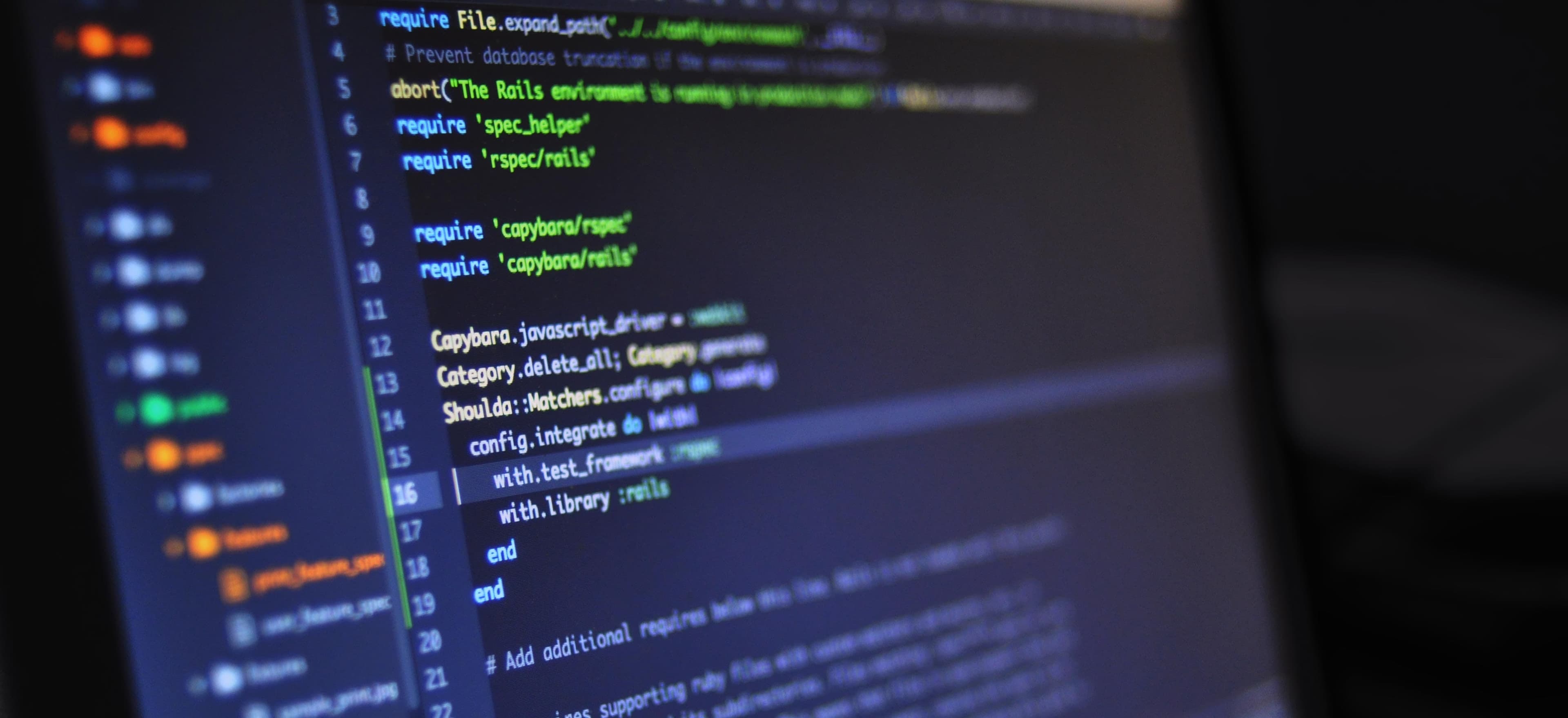
- Published on
Understanding the Impact of Garbage Collection on Java Application Performance
When it comes to building robust, high-performance Java applications, understanding how the Java Virtual Machine (JVM) manages memory is crucial. One of the key aspects of memory management in Java is the process of garbage collection. In this article, we'll delve into the impact of garbage collection on Java application performance, and examine strategies to optimize garbage collection for better application efficiency.
What is Garbage Collection?
In Java, memory allocation and deallocation are handled by the JVM through a process known as garbage collection. When objects are created in Java, memory is allocated to store them. Over time, as the program runs, objects that are no longer in use need to be removed to free up memory. Garbage collection is the process of identifying and deleting these unused objects, thus reclaiming memory for future use.
Impact of Garbage Collection on Performance
While garbage collection is an essential feature that simplifies memory management for developers, it is not without its drawbacks. The process of garbage collection can impact the performance of Java applications in several ways:
1. Pause Times
During garbage collection, the JVM halts the execution of the application to perform the necessary cleanup. This pause, known as a stop-the-world event, can lead to significant delays in the responsiveness of the application. For real-time and latency-sensitive applications, these pauses can be particularly detrimental.
2. Throughput
The frequency and duration of garbage collection cycles can directly impact the overall throughput of the application. If garbage collection occurs frequently or takes too long to complete, it can reduce the amount of time the application spends on actual useful work, thus reducing throughput.
3. Fragmentation
Garbage collection can also lead to memory fragmentation, where the available memory becomes divided into small, non-contiguous blocks. This can have implications for the efficiency of memory allocation and deallocation, potentially affecting performance.
Strategies to Optimize Garbage Collection
To mitigate the impact of garbage collection on Java application performance, it's important to employ strategies that optimize memory management and garbage collection behavior. Here are some effective approaches:
1. Object Reuse
Rather than creating and discarding objects frequently, consider reusing objects where possible. Object pools can be used to manage a set of reusable objects, reducing the frequency of object allocation and subsequently decreasing the load on garbage collection.
2. Tuning Garbage Collection Settings
The JVM provides a range of parameters and options to tune garbage collection behavior based on the specific requirements of the application. By adjusting settings such as heap size, garbage collector algorithms, and generation sizes, developers can tailor garbage collection to better suit their application's needs.
3. Monitoring and Analysis
Utilize tools and profilers to monitor garbage collection events and analyze their impact on application performance. This allows for the identification of potential bottlenecks and areas for improvement, guiding the optimization process.
4. Garbage Collection Algorithms
Java offers different garbage collection algorithms, each with its own characteristics and behaviors. Understanding the strengths and weaknesses of these algorithms can help in selecting the most suitable option for a given application.
Example: Garbage Collection Optimization in Java
Let's consider an example of how tuning garbage collection settings can impact application performance. Below is a snippet of Java code illustrating the use of JVM parameters to configure garbage collection behavior.
public class GarbageCollectionExample {
public static void main(String[] args) {
// Setting JVM heap size
// -Xms: Initial heap size
// -Xmx: Maximum heap size
// -Xmn: Size of the heap for the young generation
// Example: java -Xms512m -Xmx1024m -Xmn256m GarbageCollectionExample
// Other GC settings
// -XX:+UseG1GC: Enable the Garbage-First (G1) garbage collector
// -XX:MaxGCPauseMillis: Maximum desired pause time
// Example: java -Xms512m -Xmx1024m -Xmn256m -XX:+UseG1GC -XX:MaxGCPauseMillis=200 GarbageCollectionExample
}
}
In this example, the -Xms
, -Xmx
, and -Xmn
flags are used to set the initial heap size, maximum heap size, and the size of the young generation heap, respectively. Additionally, the -XX:+UseG1GC
flag enables the G1 garbage collector, while the -XX:MaxGCPauseMillis
flag sets the maximum desired pause time for garbage collection.
By tweaking these parameters based on the application's memory requirements and performance goals, developers can influence garbage collection behavior to align with the specific needs of the application.
Key Takeaways
Garbage collection plays a crucial role in managing memory in Java applications, but its impact on performance cannot be overlooked. The process of garbage collection can introduce latency, reduce throughput, and affect memory fragmentation if not managed effectively. By understanding the implications of garbage collection and employing optimization strategies such as object reuse, tuning garbage collection settings, monitoring, and selecting appropriate garbage collection algorithms, developers can enhance the performance of Java applications in the face of memory management challenges.
Optimizing garbage collection is a balancing act that requires a deep understanding of the application's memory usage patterns and performance requirements. Through careful analysis, tuning, and adaptation, the impact of garbage collection on Java application performance can be minimized, leading to more efficient and responsive software.
In summary, by acknowledging the significance of garbage collection in Java, and proactively addressing its potential performance implications, developers can ensure that their applications run smoothly and efficiently, even in the face of complex memory management demands.
By implementing these strategies, developers can ensure that their Java applications not only manage memory effectively but also deliver the responsiveness and throughput required for optimal performance in various use cases.
So, whether you are developing enterprise applications, data-intensive systems, or real-time solutions, being mindful of the impact of garbage collection and employing optimization strategies is key to unlocking the full potential of Java applications.
To delve deeper into this topic, refer to Paul Phillips' insightful discussion on "High-performance JVM", shedding light on critical aspects of Java performance optimization, including garbage collection strategies.
Remember, mastering memory management and garbage collection optimization is a crucial skill for any Java developer striving to build high-performance applications.