Improving Test Coverage with Stubs: Best Practices
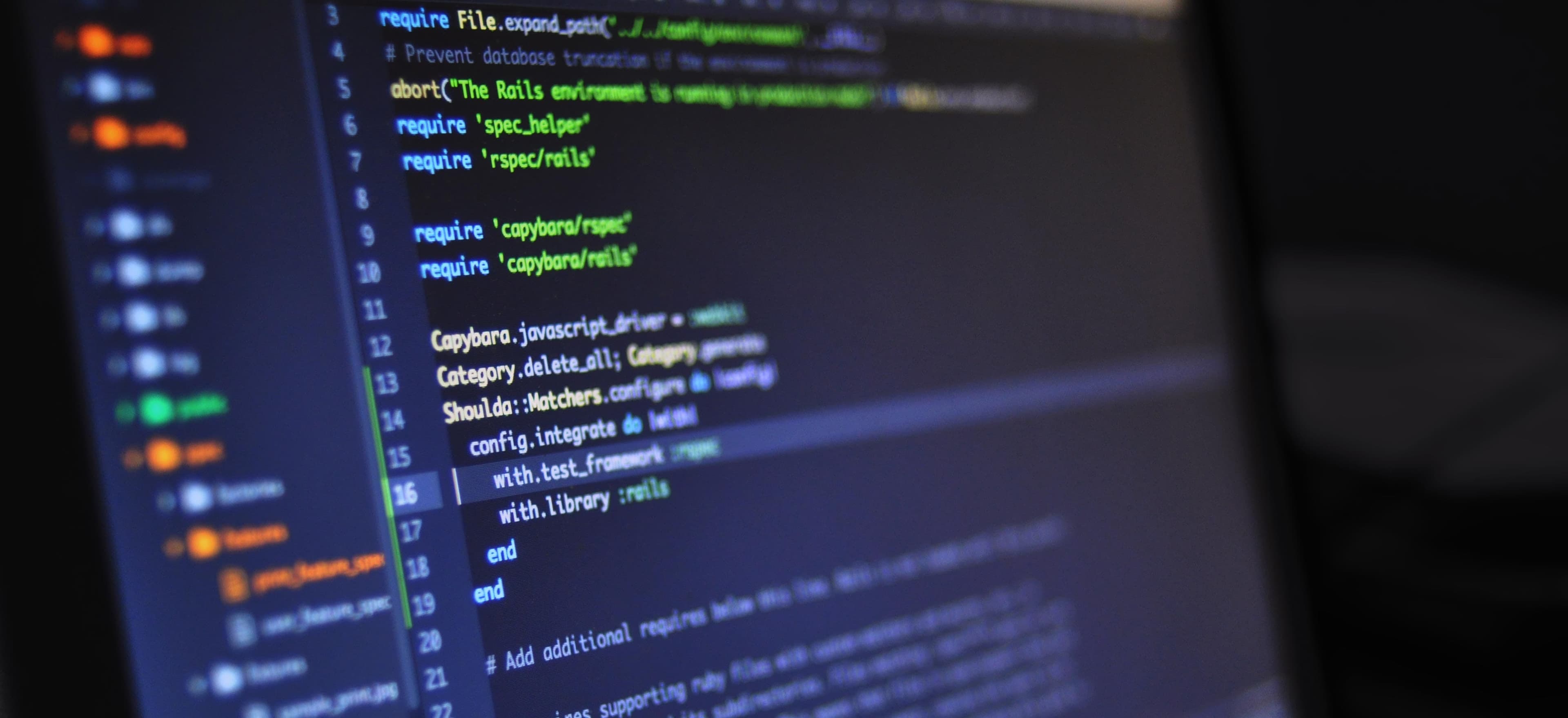
- Published on
Improving Test Coverage with Stubs: Best Practices
In today's software development landscape, writing tests for our code is fundamental. It not only helps in catching bugs early but also ensures the reliability and robustness of our applications. However, achieving high test coverage, especially for complex systems, can be challenging. One effective way to improve test coverage is by using stubs. In this article, we will explore the best practices for using stubs to enhance test coverage in Java applications.
What are Stubs?
Stubs, in the context of testing, are objects that simulate the behavior of real components in a controlled manner. When a real component is not available or its behavior is non-deterministic, stubs can be used to provide consistent and predictable responses for testing purposes. This enables us to isolate the code under test and focus solely on its behavior.
Why Use Stubs?
Using stubs in testing offers several benefits:
-
Isolation: Stubs help in isolating the code under test by providing controlled responses, thereby avoiding dependencies on external systems or complex components.
-
Deterministic Behavior: Stubs allow us to define specific behaviors, making the tests more predictable and reliable.
-
Improved Test Performance: By replacing slow or resource-intensive components with stubs, test execution time can be significantly reduced.
-
Enhanced Coverage: Stubs can be used to simulate edge cases and error scenarios, thereby improving test coverage.
Best Practices for Using Stubs
Now that we understand the advantages of using stubs, let's delve into the best practices for incorporating them into our Java projects.
1. Use Interfaces for Dependency Injection
When designing our classes, it's beneficial to use interfaces for dependencies. This promotes loose coupling and enables the use of stubs for testing. Let's consider an example:
public class UserService {
private UserDAO userDAO;
// Constructor-based injection
public UserService(UserDAO userDAO) {
this.userDAO = userDAO;
}
// ...
}
By using constructor-based dependency injection with interfaces, we can easily substitute the UserDAO
with a stub implementation during testing.
2. Leverage Frameworks for Stubbing
In Java, there are various frameworks that facilitate stubbing, such as Mockito and PowerMock. These frameworks provide convenient APIs for creating and configuring stubs, making the process more straightforward. For instance, using Mockito:
UserDAO userDAOStub = Mockito.mock(UserDAO.class);
Mockito.when(userDAOStub.findById(1)).thenReturn(new User("John"));
Here, we're stubbing the findById
method of UserDAO
to return a specific User
object when invoked with the argument 1
.
3. Focus on External Integrations
When our code interacts with external systems such as databases, APIs, or third-party services, stubs can be invaluable for testing these interactions. By stubbing the responses from these external systems, we can simulate different scenarios, including failures and timeouts, to validate the robustness of our code.
4. Parameterized Tests with Stubs
Stubs can be used in combination with parameterized tests to validate the behavior of a component under various input conditions. This approach can significantly improve test coverage by exploring different paths through the code.
@ParameterizedTest
@MethodSource("getUserData")
void testUserCreation(UserData userData) {
// Stub the UserDAO to validate user creation logic
UserDAO userDAOMock = Mockito.mock(UserDAO.class);
// ...
}
By providing various userData
inputs and stubbing the UserDAO
, we can test how the user creation logic behaves under different scenarios.
5. Maintain Readable and Maintainable Stubs
While creating stubs, it's essential to keep them readable and maintainable. Overly complex or convoluted stubs can hinder the understanding of tests. Where possible, use concise and clear stub definitions to enhance the comprehensibility of the tests.
6. Documentation and Collaboration
Document the usage and behavior of stubs, especially in cases where specific edge or error conditions are being simulated. This documentation aids collaboration among team members and ensures that the purpose of each stub is clear.
7. Integration with Continuous Integration (CI) Pipelines
Integrate the execution of tests using stubs into the CI pipelines. This ensures that the stubbed tests are run regularly, providing confidence in the functionality of the codebase.
Lessons Learned
In conclusion, using stubs is a powerful technique for enhancing test coverage in Java applications. By following best practices such as leveraging frameworks for stubbing, utilizing parameterized tests, and focusing on external integrations, we can effectively maximize the benefits of stubs in our testing strategies. Additionally, emphasizing readability, documentation, and integration with CI pipelines ensures that stubs remain an integral part of our testing practices.
By incorporating stubs into our testing arsenal, we can achieve comprehensive test coverage, leading to higher confidence in the quality and reliability of our Java applications.
Implementing stubs in Java testing - just one of the many techniques that can help ensure the seamless functioning of Java applications and software! If you're interested in learning more about Java testing, you can check out Java Testing Basics and JUnit 5 Tutorial for further insights and resources!