Optimizing Communication in Chain of Responsibility
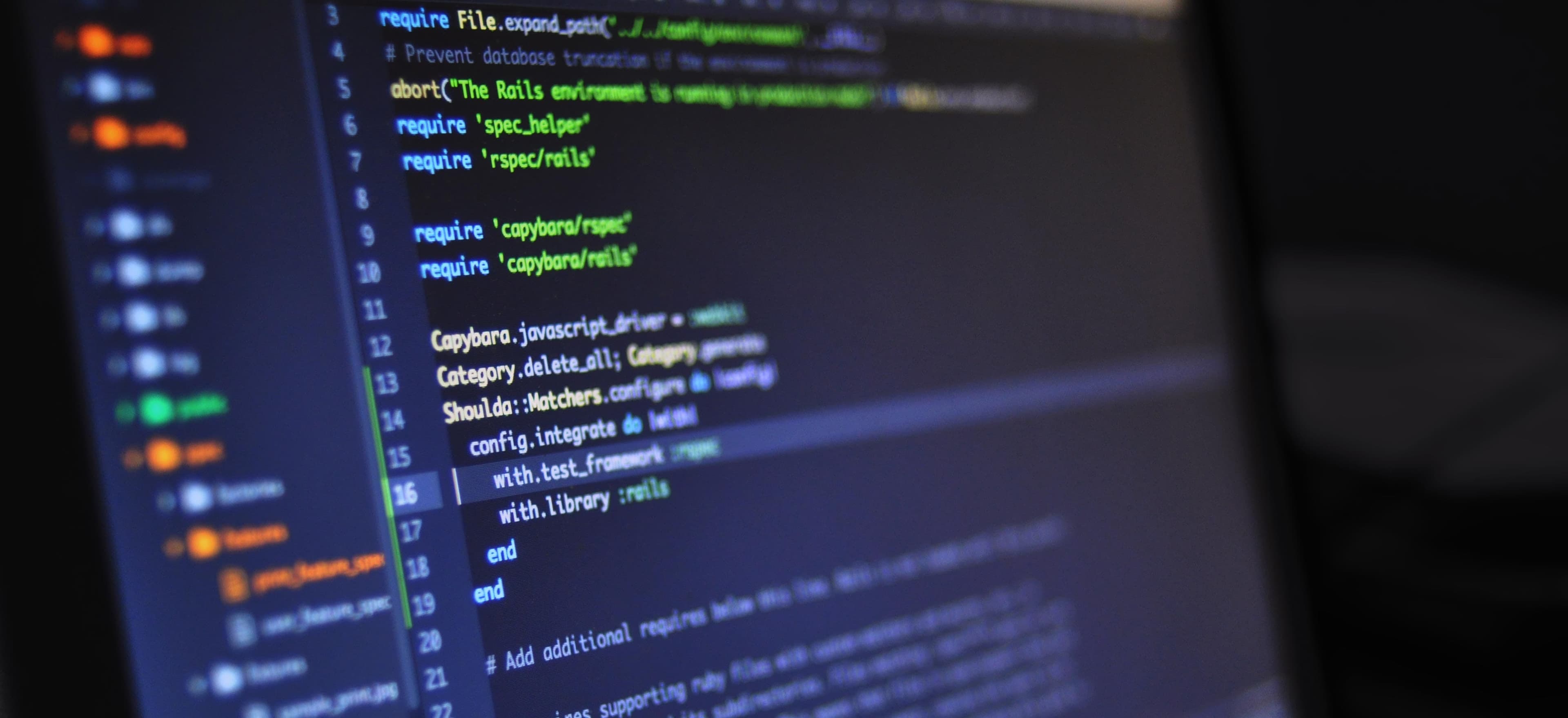
- Published on
Optimizing Communication in Chain of Responsibility Design Pattern
In the world of software development, design patterns play a crucial role in enhancing the structure, reusability, and maintainability of code. One such design pattern is the Chain of Responsibility, which allows a series of processing objects to handle a request in a seamless and decoupled manner. However, as with any pattern, there are opportunities for optimization, and communication within the chain is a critical area to address.
Understanding the Chain of Responsibility Design Pattern
The Chain of Responsibility pattern is a behavioral design pattern where a request is passed along a chain of handlers. Each handler decides whether to process the request or pass it along to the next handler in the chain. This pattern promotes loose coupling and flexibility, as the sender of a request is unaware of which handler will ultimately process it.
Let's consider a scenario where different levels of authorization need to be applied to a user's request. The Chain of Responsibility pattern can be employed to create a chain of authorization handlers, each responsible for a particular level of authorization. The request flows through the chain until it finds a handler capable of handling it.
Optimizing Communication in the Chain
While the basic implementation of the Chain of Responsibility pattern effectively allows the request to flow through the chain, optimizing the communication within the chain can improve its efficiency and flexibility.
1. Establishing a Clear Protocol
In order to optimize the communication within the chain, it's essential to establish a clear and consistent protocol that defines how handlers communicate with each other and process requests. This protocol should encompass the format of the request, the response, and any contextual information that needs to be passed along.
2. Standardizing Request and Response Objects
To streamline communication, it's beneficial to standardize the structure of the request and response objects that flow through the chain. This ensures that handlers can seamlessly process requests and interpret responses without ambiguity.
Let's consider a simplified example of a request and response object:
public class Request {
private String data;
public Request(String data) {
this.data = data;
}
public String getData() {
return data;
}
}
public class Response {
private boolean isAuthorized;
public Response(boolean isAuthorized) {
this.isAuthorized = isAuthorized;
}
public boolean isAuthorized() {
return isAuthorized;
}
}
By standardizing the request and response objects, handlers can confidently process requests and generate responses without being concerned with the intricacies of the request's origin or the subsequent handler's expectations.
3. Introducing Contextual Information
In certain scenarios, passing contextual information along with the request can aid handlers in making processing decisions. This contextual information can include metadata about the request, user-specific data, or any other relevant details that enhance the processing capabilities of the chain.
4. Utilizing Exceptions for Flow Control
In situations where a handler encounters an exceptional condition that prevents it from processing the request, leveraging exceptions for flow control can efficiently redirect the request to alternative paths within the chain. This approach can minimize nested conditional statements and promotes a cleaner, more streamlined implementation.
5. Logging and Monitoring
In complex chain of responsibility implementations, incorporating robust logging and monitoring mechanisms can provide invaluable insights into the flow of requests and the behavior of handlers. This visibility facilitates debugging, performance optimization, and the identification of potential bottlenecks within the chain.
Example Implementation
Now, let's illustrate the optimization of communication within the chain of responsibility with a Java code snippet that demonstrates a simplified authorization chain.
public interface Handler {
void setNext(Handler handler);
void handleRequest(Request request);
}
public class BaseHandler implements Handler {
private Handler next;
@Override
public void setNext(Handler handler) {
this.next = handler;
}
@Override
public void handleRequest(Request request) {
if (next != null) {
next.handleRequest(request);
}
}
}
public class AuthorizationHandler extends BaseHandler {
@Override
public void handleRequest(Request request) {
// Perform authorization logic based on the request data
// Set response based on authorization outcome
boolean isAuthorized = true; // Example authorization logic
Response response = new Response(isAuthorized);
// Process the response or pass it along to the next handler
// ...
super.handleRequest(request);
}
}
In this example, we see the utilization of a basic chain of responsibility structure with an AuthorizationHandler extending the BaseHandler. The optimization principles discussed earlier can be integrated into the implementation of handleRequest() to improve communication within the chain.
The Bottom Line
The Chain of Responsibility pattern is a powerful tool for managing the processing of requests in a flexible and decoupled manner. By optimizing communication within the chain, we can enhance its efficiency, maintainability, and robustness. Standardizing protocols, utilizing contextual information, and leveraging exceptions for flow control are essential practices to consider when fine-tuning the communication within the Chain of Responsibility pattern.
In conclusion, a well-optimized communication mechanism within the Chain of Responsibility pattern not only improves the overall design and performance but also promotes a clear and intuitive interaction between the processing objects. By incorporating these optimization techniques, software engineers can elevate the effectiveness of the pattern and its applicability to diverse real-world scenarios.
By implementing these thoughtful practices, you can enhance the communication within a Chain of Responsibility and unlock its full potential in your software projects!
For further reading on the Chain of Responsibility pattern and optimization techniques, refer to Refactoring Guru and Baeldung.
Remember, the key to optimizing the Chain of Responsibility pattern lies in clear communication and thoughtful design. By implementing these practices, you can unlock the full potential of this powerful design pattern in your software projects. Happy coding!