Optimizing State Management in Clojure Web Development
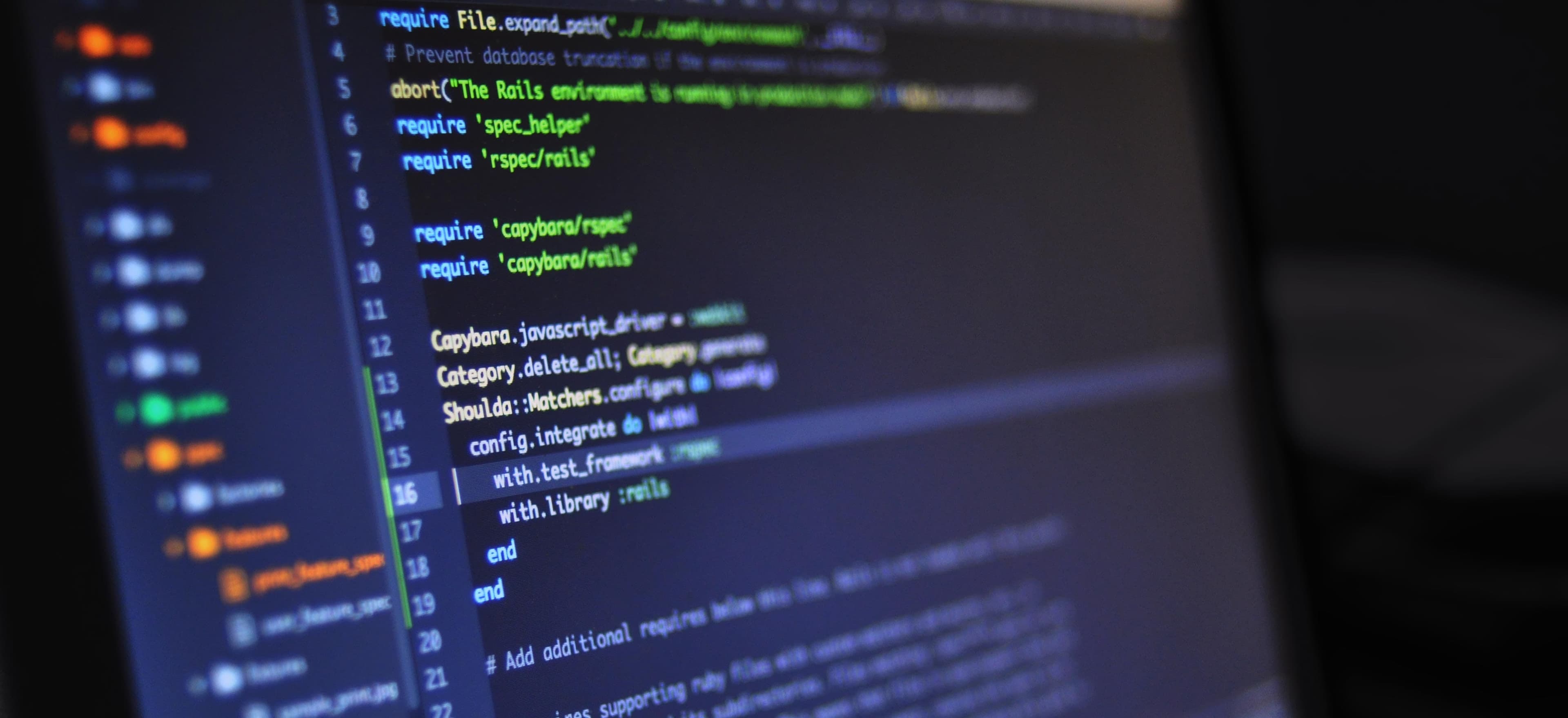
- Published on
Understanding State Management in Clojure Web Development
When it comes to building web applications in Clojure, managing state effectively is crucial for maintaining application stability and responsiveness. In this blog post, we'll delve into the intricacies of state management in Clojure web development, exploring best practices and optimization techniques to ensure a seamless user experience.
The Importance of State Management
In web development, state refers to the data that is stored and manipulated by the application. This can include user input, session information, and the current application state. Effective state management is essential for ensuring that the web application behaves predictably and maintains a coherent user interface.
Clojure, being a functional programming language, adopts a different approach to managing state compared to traditional imperative languages. Instead of mutable state and shared memory, Clojure emphasizes immutable data and pure functions. This paradigm shift presents both challenges and opportunities for state management in web development.
Leveraging Clojure's Immutable Data Structures
One of Clojure's core strengths lies in its immutable data structures. By default, data in Clojure is immutable, meaning that once created, it cannot be modified. This immutability simplifies concurrent programming and makes it easier to reason about the behavior of the code.
When managing state in Clojure web applications, leveraging immutable data structures such as maps, sets, and vectors allows for efficient and safe state manipulation. For example, consider the following code snippet:
(def user-state (ref {:name "John" :age 30}))
(dosync
(alter user-state assoc :age 31))
In this example, we define a user-state ref that contains user information. The alter
function is used within a dosync
block to atomically update the user's age. By using immutable data structures and ensuring that state updates are performed atomically, we minimize the risk of race conditions and unexpected side effects.
Managing Application State with Atoms
Clojure provides the atom
construct for managing application state. An atom is a reference type that holds a single, mutable value. While the value contained within an atom can be changed, the atom itself remains immutable.
Let's consider a scenario where we use an atom to manage the state of a user's shopping cart:
(def shopping-cart (atom #{}))
(swap! shopping-cart conj "Product A")
(swap! shopping-cart conj "Product B")
In this example, we define a shopping-cart atom that initially contains an empty set. We then use the swap!
function to update the shopping cart by adding products to it. The immutability of the atom ensures that concurrent state updates are managed safely.
Dealing with Global State Using Vars
In Clojure, vars are used to define and reference global and dynamic bindings. While vars are typically used for managing global state, it's important to exercise caution when employing them, as global state can introduce complexity and make code harder to reason about.
When dealing with global state using vars, consider encapsulating the state within a namespace and providing well-defined interfaces for manipulating the state. This encapsulation helps in maintaining modularity and isolating the impact of state changes.
Asynchronous State Updates with Agents
Clojure's agent construct provides a mechanism for managing state in an asynchronous and non-blocking manner. Agents encapsulate a single state value and manage the state updates asynchronously, making them suitable for scenarios where immediate state consistency is not critical.
Here's an example of using an agent to process incoming user requests asynchronously:
(def processing-agent (agent {}))
(send processing-agent
(fn [state request]
(process-request state request)))
In this example, we define a processing-agent that encapsulates the application's state. We use the send
function to asynchronously process incoming user requests, updating the state as necessary. By leveraging agents, we can offload non-critical state updates to separate threads, improving the responsiveness of the web application.
Optimizing State Management for Performance
While Clojure's emphasis on immutable data structures and pure functions contributes to robust state management, optimizing state operations for performance is equally important. Clojure's persistent data structures provide efficient ways to create new versions of data without copying the entire structure, but understanding the underlying algorithms and performance implications is crucial for optimizing state management.
Persistent Data Structures
Clojure's persistent data structures, such as vectors, maps, and sets, utilize structural sharing to efficiently create new versions of data without duplicating the entire structure. This persistent and efficient approach to state manipulation minimizes memory overhead and enhances performance.
Transients for Mutable State Updates
In scenarios where performance is critical and a series of mutable state updates are required, Clojure provides the concept of transients. Transients allow for transient mutation of persistent data structures, providing a way to perform efficient and mutable state updates within a controlled scope.
(defn update-state [data]
(let [transient-state (transient data)]
(-> transient-state
(assoc! :key1 "value1")
(assoc! :key2 "value2")
(persistent!))))
In this code snippet, we define a function update-state
that takes a persistent data structure as an argument. Within the function, we use transients to efficiently perform multiple mutable state updates before converting the transient state back to a persistent data structure. This approach minimizes the overhead of creating intermediate data copies, leading to improved performance.
My Closing Thoughts on the Matter
Effective state management is a fundamental aspect of developing robust and performant web applications in Clojure. By leveraging immutable data structures, reference types, and asynchronous state management constructs, developers can ensure that state is managed safely and efficiently. Furthermore, optimizing state operations for performance through persistent data structures and transients enhances the responsiveness of Clojure web applications.
In conclusion, understanding the intricacies of state management in Clojure web development and applying optimization techniques is essential for delivering a seamless user experience and maintaining the stability of web applications.
Now that you have a comprehensive understanding of state management in Clojure web development, you're well-equipped to tackle the challenges of handling state effectively and optimizing performance in your web applications.