The Challenge of Managing Dependency Injection in Spring Framework
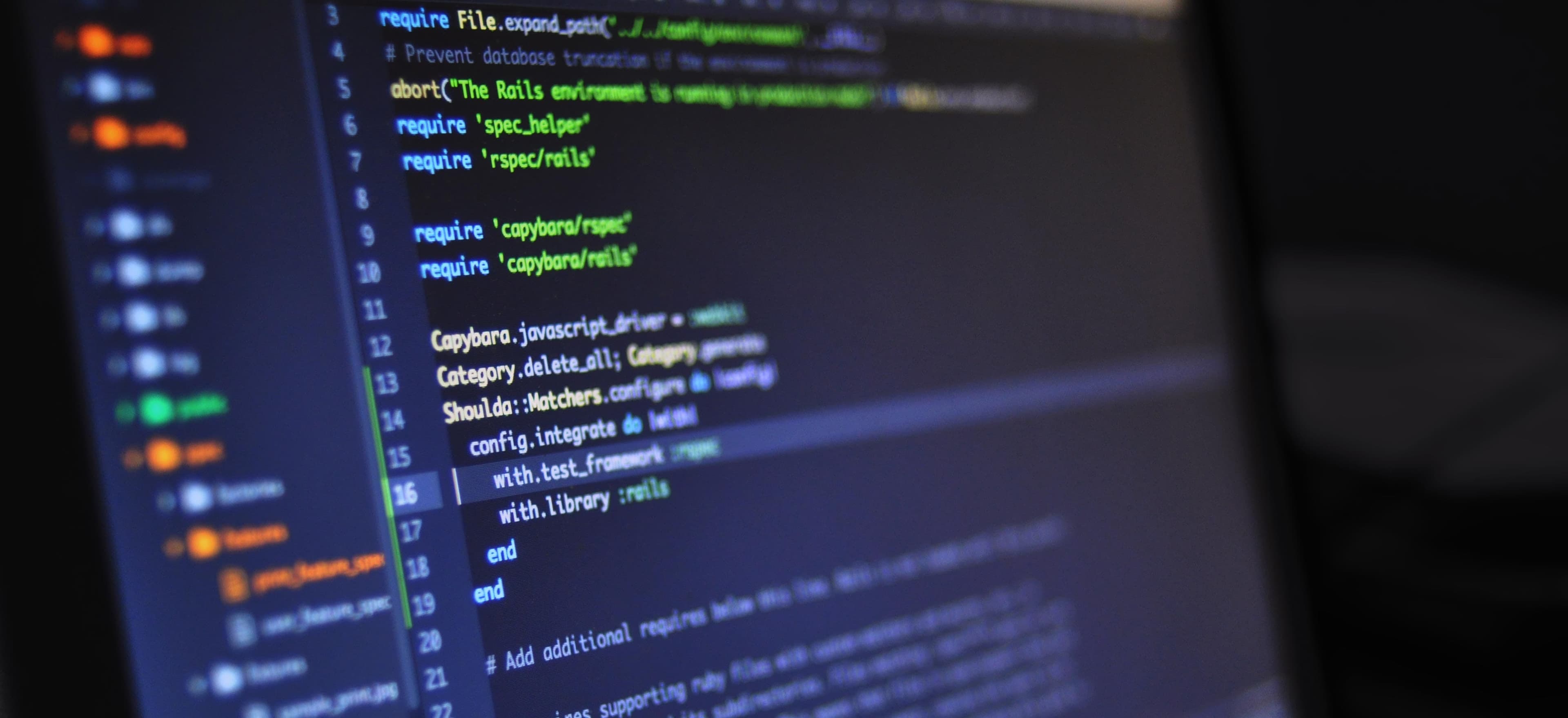
- Published on
The Challenge of Managing Dependency Injection in Spring Framework
Dependency Injection (DI) is a powerful design pattern that is widely used in the development of Java applications, and the Spring Framework is a popular choice for implementing DI. While DI offers numerous benefits such as improved modularization, easier testing, and reduced coupling, managing dependencies in a Spring application can become complex as the project grows in size and complexity.
In this blog post, we will explore the challenges associated with managing dependency injection in the Spring Framework and discuss strategies to address them.
Understanding Dependency Injection in Spring
At its core, the Spring Framework leverages dependency injection to allow the components of an application to be loosely coupled. This means that the components do not need to know the details of how their dependencies are created or configured, as the framework takes care of injecting them at runtime.
public class OrderService {
private final OrderRepository orderRepository;
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
// ...
}
In the example above, the OrderService
class has a dependency on the OrderRepository
, which is injected via its constructor. This allows the OrderService
to be easily tested and reused without being tightly coupled to the specific implementation of OrderRepository
.
The Challenge
1. Managing Configuration
As an application grows, managing the configuration of dependencies becomes increasingly challenging. In a large Spring application, there may be numerous components with various dependencies, and managing the configuration of these dependencies can become complex and error-prone.
2. Circular Dependencies
Circular dependencies, where two or more beans depend on each other directly or indirectly, can pose a significant challenge in Spring applications. Resolving circular dependencies manually can be tricky and may lead to runtime issues.
3. Complexity in Wiring
As the number of components and their dependencies grows, the process of wiring them together becomes more complex. This complexity can make it difficult to understand and maintain the application's architecture.
4. Unit Testing Dependencies
In some cases, unit testing dependencies injected by the Spring container can be cumbersome. For example, when testing a service that relies on multiple dependencies, mocking or stubbing these dependencies for testing purposes can become complex and lead to brittle test suites.
Strategies for Managing Dependency Injection
1. Modularization and Component Scanning
Organizing the application into smaller, modular components and leveraging component scanning can help simplify the management of dependencies. By defining clear boundaries between components and leveraging Spring's component scanning capabilities, the configuration of dependencies can be streamlined.
2. Constructor Injection
Prefer using constructor injection over other forms of DI such as setter or field injection. Constructor injection makes dependencies explicit and enforces their presence, making the application more predictable and easier to test.
public class OrderService {
private final OrderRepository orderRepository;
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
// ...
}
3. Using Qualifiers and Profiles
Spring provides qualifiers and profiles to help disambiguate dependencies and conditionally activate certain beans based on the application's environment or requirements. Leveraging qualifiers and profiles can help manage complex configurations more effectively.
4. Applying Design Patterns
Applying design patterns such as the Factory pattern or the Strategy pattern can help manage complex dependencies and provide more flexibility in how dependencies are created and injected.
5. Integration Testing
In addition to unit testing, integration testing can provide a comprehensive view of how the application's components and their dependencies work together. Integration testing can help uncover issues related to dependency wiring and circular dependencies.
6. Using Dependency Injection Containers
Consider using advanced DI containers such as Google Guice or Spring's own advanced features like Bean Scopes and Conditional Annotations. These containers offer additional features for managing and controlling dependency injection that can help address complex scenarios.
A Final Look
While managing dependency injection in Spring applications can be challenging, employing effective strategies and best practices can help mitigate these challenges. By emphasizing modularization, explicit dependency management, and leveraging advanced features of the Spring Framework, developers can create well-structured and maintainable applications.
In conclusion, as applications grow in complexity, the management of dependency injection becomes crucial for maintaining the scalability, testability, and maintainability of the codebase.
For more information on dependency injection in Java and the Spring Framework, you can refer to the following resources:
Happy coding!
Checkout our other articles