Handling Circular References in Custom JSON Deserialization
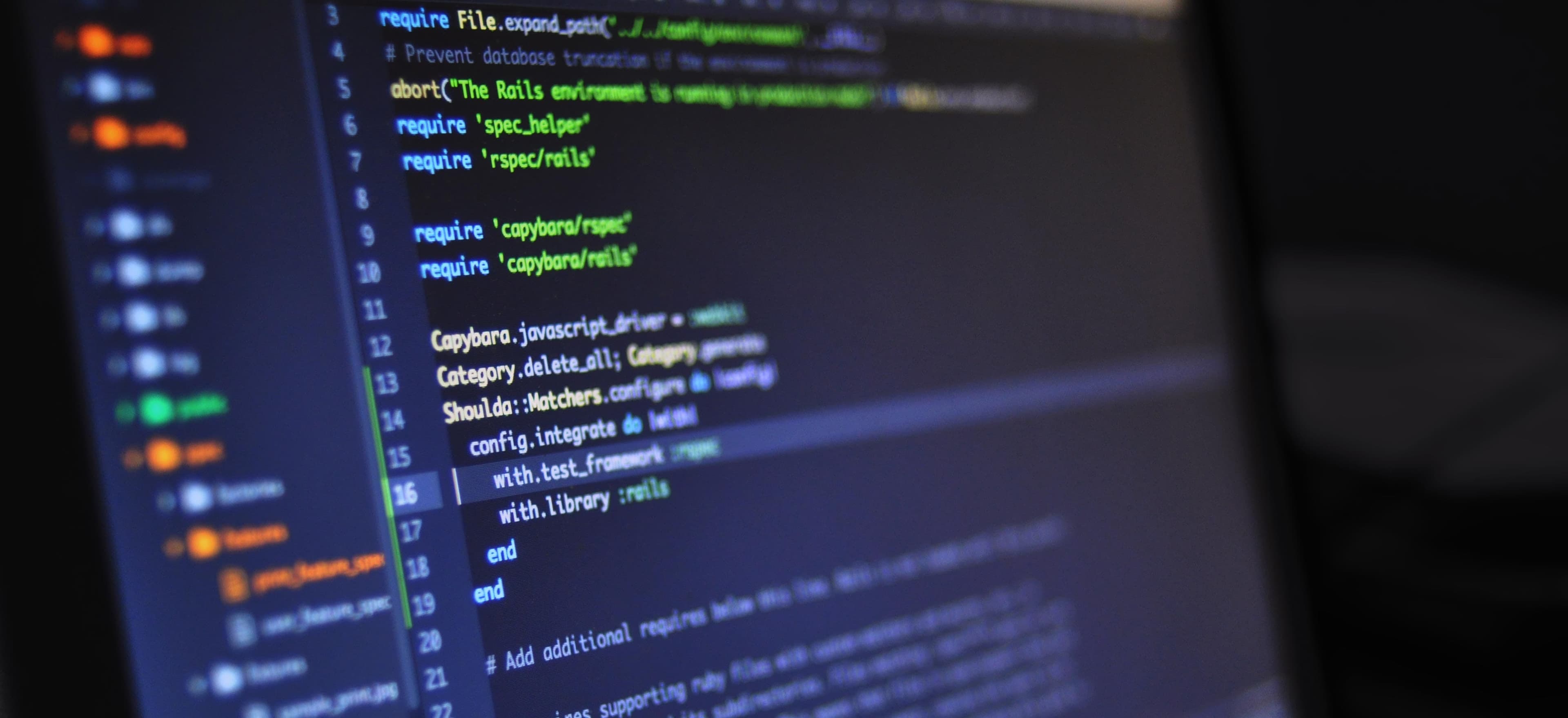
- Published on
How to Handle Circular References in Custom JSON Deserialization
When working with JSON data in Java, it's common to encounter situations where circular references exist. Circular references occur when an object refers back to itself either directly or indirectly through a chain of references.
In this article, we'll explore how to handle circular references during custom JSON deserialization in Java, using Jackson as our JSON processing library.
Understanding the Issue
Circular references can cause issues during deserialization because they can lead to infinite loops or stack overflow errors if not handled properly.
Consider the following example:
public class Employee {
private String name;
private Employee manager;
// getters and setters
}
In this scenario, an Employee
object has a reference to another Employee
object, creating a circular reference when serializing and deserializing this data.
Using Jackson's @JsonIdentityInfo
Annotation
Jackson provides a solution to handle circular references using the @JsonIdentityInfo
annotation. This annotation helps in serializing and deserializing objects with circular references by using an identifier to represent the object and its references.
Let's see how we can use @JsonIdentityInfo
in a custom deserializer.
@JsonIdentityInfo(generator = ObjectIdGenerators.PropertyGenerator.class, property = "id")
public class Employee {
private String name;
private Employee manager;
// getters and setters
}
In the above example, we have annotated the Employee
class with @JsonIdentityInfo
. We've specified that the PropertyGenerator
should be used to generate identifiers based on the id
property of the object.
Implementing a Custom Deserializer
To handle circular references effectively, we can create a custom deserializer that leverages the @JsonIdentityInfo
annotation.
Here's an example of how we can implement a custom deserializer for the Employee
class:
public class EmployeeDeserializer extends JsonDeserializer<Employee> {
@Override
public Employee deserialize(JsonParser p, DeserializationContext ctxt) throws IOException, JsonProcessingException {
ObjectCodec codec = p.getCodec();
JsonNode node = codec.readTree(p);
// Instantiate the Employee using the id
long id = node.get("id").asLong();
Employee employee = findEmployeeById(id); // Custom method to find employee by id
// Set other properties of the employee
employee.setName(node.get("name").asText());
// Return the deserialized employee
return employee;
}
}
In the EmployeeDeserializer
, we override the deserialize
method to customize the deserialization process. We read the JSON tree using the provided JsonParser
, extract the necessary information, and construct the Employee
object with the help of a custom method findEmployeeById
.
Registering the Custom Deserializer
Once we have the custom deserializer, we need to register it with the ObjectMapper
provided by Jackson.
ObjectMapper mapper = new ObjectMapper();
SimpleModule module = new SimpleModule();
module.addDeserializer(Employee.class, new EmployeeDeserializer());
mapper.registerModule(module);
In the above code, we create a SimpleModule
and add our custom deserializer for the Employee
class. Then, we register this module with the ObjectMapper
to handle the deserialization of Employee
objects.
Serializing Objects with Circular References
When serializing objects with circular references, Jackson will use the identifiers generated by @JsonIdentityInfo
to represent the references. This ensures that circular references are properly handled during serialization as well.
A Final Look
Handling circular references in custom JSON deserialization is crucial to avoid infinite loops and stack overflow errors. Jackson's @JsonIdentityInfo
annotation along with a custom deserializer provides a powerful solution to this problem.
By leveraging these techniques, you can effectively handle circular references in your Java applications, ensuring smooth JSON deserialization without running into unexpected issues.
For further reading on Jackson's features and capabilities, check out the official Jackson documentation.
Happy coding!
Checkout our other articles