Overcoming Remote Testing Challenges
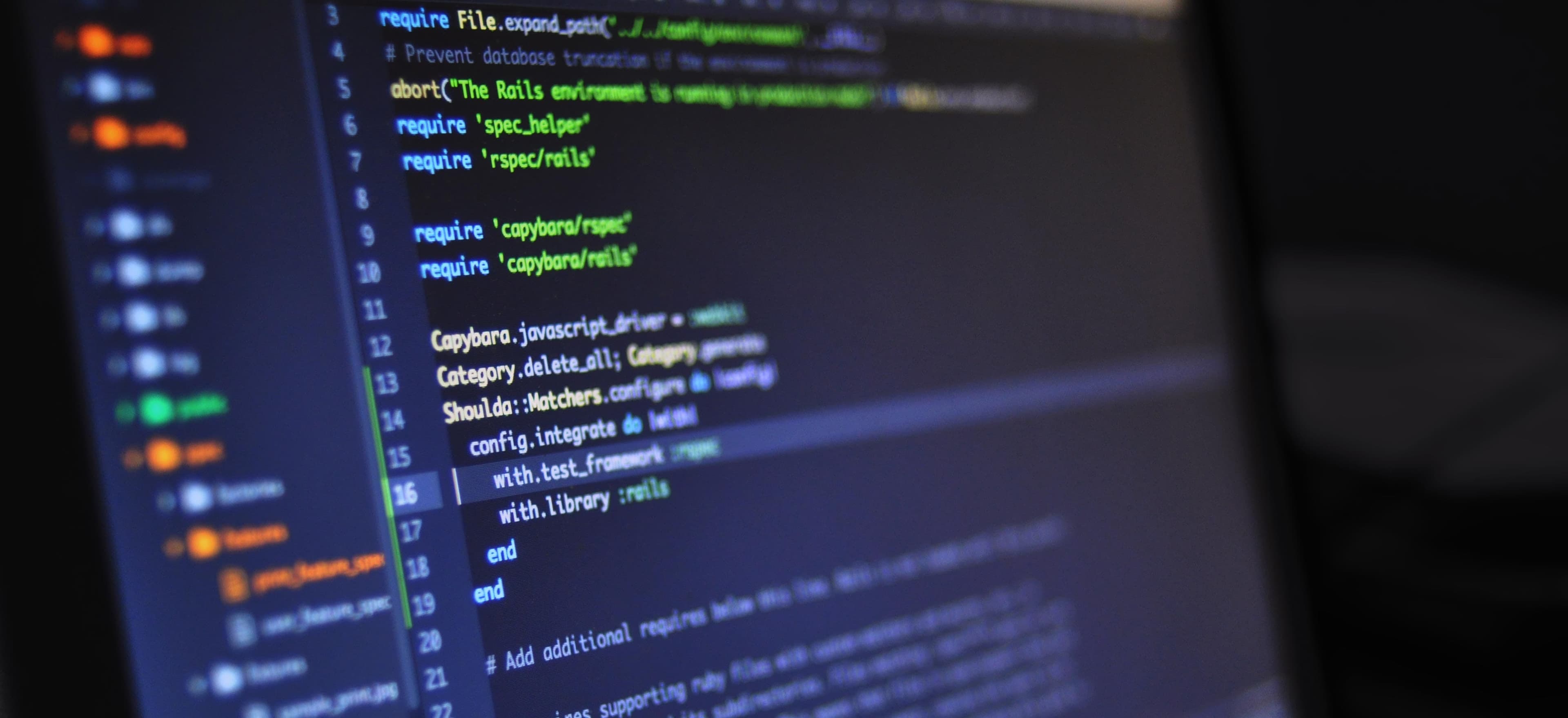
- Published on
Remote Testing in Java: Overcoming Challenges
Remote testing in Java has become an essential practice in the modern software development lifecycle. It allows teams to efficiently test their applications on various platforms and configurations without the need for physical infrastructure. However, remote testing comes with its own set of challenges that developers need to overcome to ensure the reliability and effectiveness of their testing efforts. In this post, we will explore some common challenges associated with remote testing in Java and discuss strategies to overcome them.
Challenge 1: Network Latency
One of the primary challenges of remote testing is network latency. When running tests on remote servers, the round-trip time for executing test cases and receiving results can be significantly higher compared to local testing. This can lead to longer feedback loops and slower test execution times, impacting overall productivity.
Solution:
To mitigate network latency issues, utilize tools like JUnit Lambda to parallelize test execution. By running tests in parallel, you can distribute the workload across multiple remote instances, reducing the impact of network latency on overall test execution time.
@Test
void testSomething() {
// Test logic
}
@Test
void testAnotherThing() {
// Test logic
}
Challenge 2: Resource Management
Managing resources such as databases, third-party services, and external dependencies during remote testing can be challenging. Ensuring that the required resources are available and properly configured on remote test environments is crucial for accurate testing.
Solution:
Utilize containerization tools like Docker to create reproducible test environments. By encapsulating dependencies within Docker containers, you can easily spin up and tear down isolated test environments, ensuring consistent resource availability across remote testing instances.
// Dockerfile for creating a test environment with required dependencies
FROM openjdk:11
// Add commands to set up the environment
Challenge 3: Test Data Management
Managing test data across remote testing environments, especially when dealing with sensitive or large datasets, can pose a significant challenge. Ensuring that test data remains consistent and secure across different testing instances is essential for reliable testing.
Solution:
Implement data anonymization and masking techniques to generate synthetic test data for remote testing environments. Tools like Mockaroo can be used to create realistic yet anonymized datasets, ensuring data consistency and security across remote testing instances.
// Code to generate anonymized test data using Mockaroo API
Challenge 4: Test Result Analysis
Analyzing test results from remote testing environments, especially when dealing with a large number of test cases and instances, can be overwhelming. Collating and interpreting test results to identify issues and trends is crucial for maintaining test efficiency.
Solution:
Utilize test reporting and analysis tools such as Allure Framework to generate comprehensive and interactive test reports. These reports can provide detailed insights into test execution across remote instances, aiding in identifying and addressing issues effectively.
// Integration of Allure Framework for generating test reports
A Final Look
Remote testing in Java presents unique challenges related to network latency, resource management, test data, and result analysis. By leveraging parallel execution, containerization, data anonymization, and advanced reporting tools, teams can overcome these challenges and establish efficient and reliable remote testing workflows. As software development continues to embrace remote and distributed practices, addressing these challenges becomes crucial for ensuring the quality and integrity of Java applications.
In conclusion, remote testing in Java is a vital aspect of modern software development that requires thoughtful consideration and proactive strategies to overcome its associated challenges. By implementing the solutions discussed in this post, teams can streamline their remote testing workflows and ensure the effectiveness of their testing efforts in distributed environments. Stay proactive and adaptive to emerging tools and practices to stay ahead in the remote testing game!
Checkout our other articles