Efficient AJAX CRUD Operations in Play Framework for Twitter-Like App
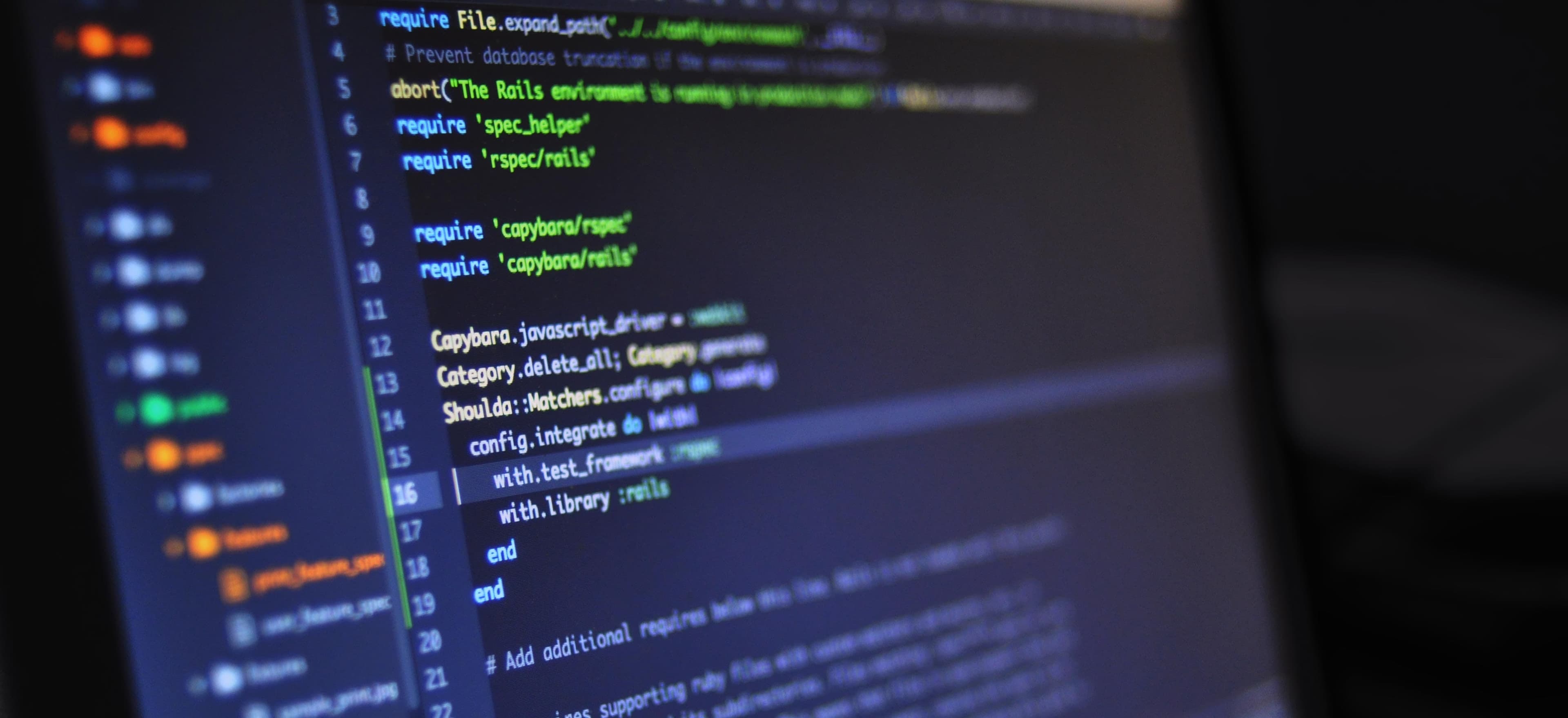
- Published on
Building Efficient AJAX CRUD Operations in Play Framework for a Twitter-Like App
When developing a modern web application, smooth and efficient user interactions are crucial. In the case of a Twitter-like app, the ability to create, read, update, and delete (CRUD) tweets via AJAX requests can significantly enhance the user experience. In this post, we will explore how to implement efficient AJAX CRUD operations in a Play Framework application using Java.
Setting the Stage with Play Framework
Play Framework, known for its high performance and developer-friendly features, provides an excellent foundation for building web applications. Its support for asynchronous programming and built-in HTTP server makes it a great choice for building modern web applications that require real-time interactions.
The AJAX approach allows us to communicate with the server in the background, without the need to reload the entire page. This results in a seamless user experience, making it an ideal choice for handling CRUD operations in a Twitter-like app.
Creating the Tweet Model
Let’s begin by setting up our model for tweets. In this example, we'll use a simple Tweet
model with an id
, username
, and content
field. First, create the Tweet
class:
public class Tweet {
private Long id;
private String username;
private String content;
// Getters and setters
}
The id
field serves as the unique identifier for each tweet, while username
and content
hold the user's name and the tweet content, respectively.
Implementing the Controller
Next, we need to implement the controller to handle the AJAX requests for CRUD operations. We'll create methods for creating, reading, updating, and deleting tweets. Let's take a look at the TweetController
:
public class TweetController extends Controller {
public Result createTweet() {
// Handle AJAX request to create a new tweet
}
public Result getTweet(Long id) {
// Handle AJAX request to retrieve a tweet by id
}
public Result updateTweet(Long id) {
// Handle AJAX request to update a tweet by id
}
public Result deleteTweet(Long id) {
// Handle AJAX request to delete a tweet by id
}
}
Here, each method corresponds to a specific CRUD operation. For example, createTweet
handles the creation of a new tweet, getTweet
retrieves a tweet by its ID, updateTweet
updates a tweet, and deleteTweet
removes a tweet from the system.
Leveraging AJAX with jQuery
Now, let’s dive into the client-side implementation using AJAX requests powered by jQuery. We'll use jQuery to make asynchronous requests to our Play Framework application and update the UI based on the server's response.
To create a new tweet using AJAX, we can utilize the following jQuery code:
$('#tweetForm').submit(function(event) {
event.preventDefault();
var tweetData = {
username: $('#username').val(),
content: $('#content').val()
};
$.ajax({
type: 'POST',
url: '/createTweet',
contentType: 'application/json',
data: JSON.stringify(tweetData),
success: function(data) {
// Handle success, e.g., update UI
},
error: function(err) {
// Handle error, e.g., display error message
}
});
});
In this snippet, we intercept the form submission, serialize the form data into a JSON object, and send it to the server using a POST request. Upon a successful response from the server, we can update the UI to reflect the new tweet. In the case of an error, we can notify the user accordingly.
Making the Tweets Load Dynamically
Loading tweets dynamically as the user scrolls down the page can enhance the user experience. To achieve this, we can leverage infinite scrolling using AJAX. Let's take a look at how we can implement this behavior with jQuery:
var isLoadingTweets = false;
$(window).scroll(function() {
if ($(window).scrollTop() + $(window).height() >= $(document).height() - 100 && !isLoadingTweets) {
isLoadingTweets = true;
$.ajax({
type: 'GET',
url: '/loadTweets',
success: function(data) {
// Append loaded tweets to the UI
isLoadingTweets = false;
},
error: function(err) {
// Handle error, e.g., display error message
isLoadingTweets = false;
}
});
}
});
In this code, as the user scrolls down, we detect when they are close to the bottom of the page. At this point, we trigger an AJAX request to load more tweets. Once the response is received, we append the newly loaded tweets to the UI. The isLoadingTweets
flag prevents multiple simultaneous requests.
Updating Tweets with AJAX
When a user wishes to edit a tweet, an AJAX request can be used to update the tweet content without needing to navigate away from the page. Here's an example of how we can achieve this using jQuery:
$('.editTweetButton').click(function() {
var tweetId = $(this).data('id');
var newContent = prompt('Enter the new content for the tweet:');
if (newContent) {
var tweetData = {
content: newContent
};
$.ajax({
type: 'PUT',
url: '/updateTweet/' + tweetId,
contentType: 'application/json',
data: JSON.stringify(tweetData),
success: function(data) {
// Update the tweet content in the UI
},
error: function(err) {
// Handle error, e.g., display error message
}
});
}
});
In this snippet, when the user clicks on the edit button for a tweet, they are prompted to enter the new content. Upon submitting the new content, an AJAX PUT request is sent to update the tweet. After a successful response, we can update the tweet content in the UI.
Deleting Tweets via AJAX
Finally, let's address the deletion of tweets through asynchronous requests. This ensures that the user can remove tweets without experiencing any page reloads. Here is an example of how we can handle tweet deletion with AJAX:
$('.deleteTweetButton').click(function() {
var tweetId = $(this).data('id');
if (confirm('Are you sure you want to delete this tweet?')) {
$.ajax({
type: 'DELETE',
url: '/deleteTweet/' + tweetId,
success: function(data) {
// Remove the deleted tweet from the UI
},
error: function(err) {
// Handle error, e.g., display error message
}
});
}
});
Upon clicking the delete button for a tweet, the user is prompted to confirm the deletion. If confirmed, an AJAX DELETE request is sent to remove the tweet. Subsequently, the tweet is removed from the UI upon a successful response from the server.
Final Thoughts
Efficient and seamless handling of CRUD operations plays a pivotal role in enhancing user interactions within web applications. By leveraging AJAX with Play Framework and jQuery, we can achieve smooth and real-time CRUD operations, making the user experience in a Twitter-like app more engaging and responsive.
In summary, we have explored how to create, read, update, and delete tweets through efficient AJAX requests in a Play Framework application. This approach not only demonstrates modern development techniques but also showcases the potential to deliver a high-quality user experience.
With this newfound knowledge, you are well-equipped to implement efficient AJAX CRUD operations in your own Play Framework applications, providing users with a delightful and responsive web experience.
Happy coding!