Building Real-Time Data Processing in Spring Integration
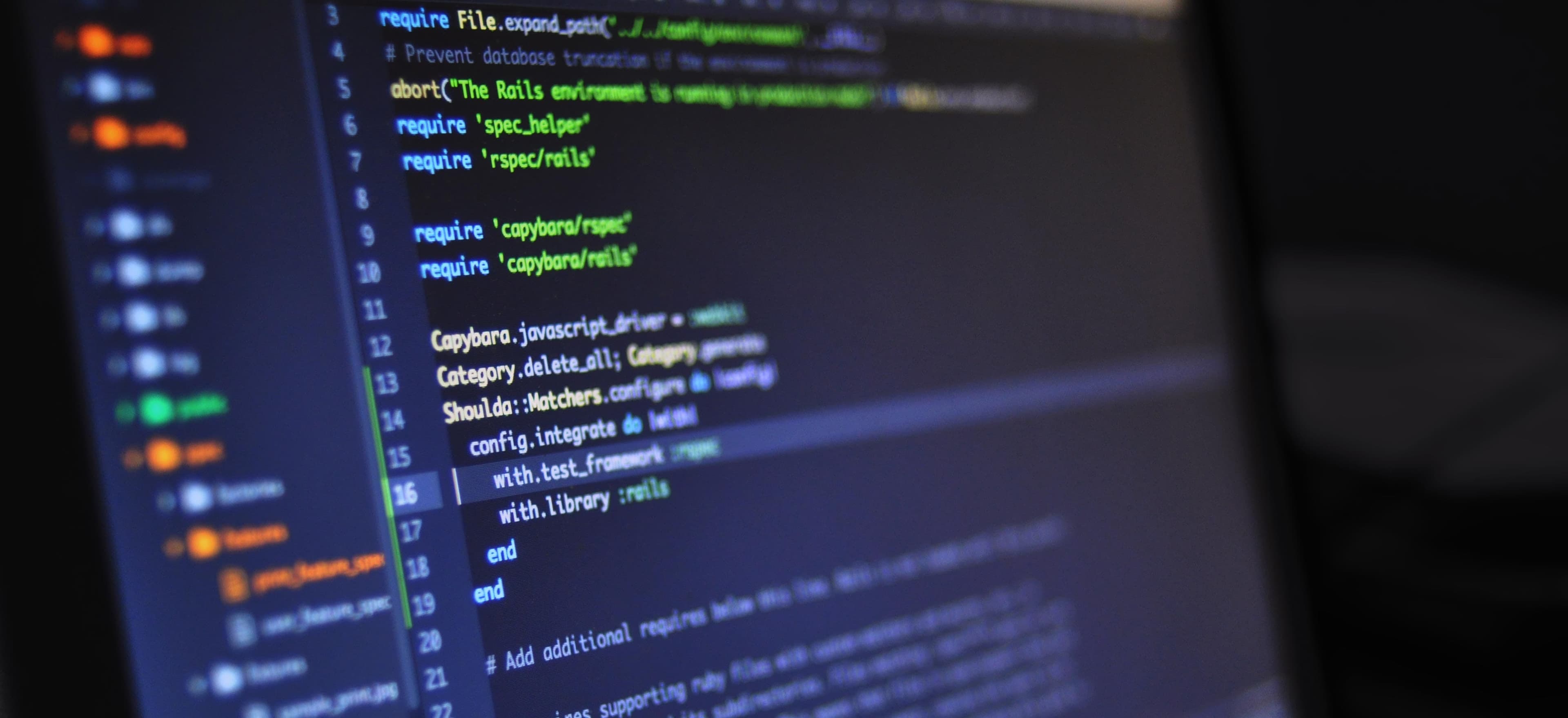
- Published on
Real-Time Data Processing with Spring Integration
Real-time data processing is crucial for many applications, especially in today's fast-paced, data-intensive environments. In this blog post, we will explore how to implement real-time data processing using Spring Integration, a lightweight messaging framework built on top of the Spring framework. We will delve into the core concepts of Spring Integration and demonstrate how it can be used to handle real-time data processing scenarios.
What is Spring Integration?
Spring Integration is an extension of the Spring programming model that aims to simplify the integration of enterprise applications. It provides a set of building blocks for writing message-driven applications, allowing seamless integration of various systems through messaging. These building blocks include message channels, message endpoints, message handlers, and message transformers.
Setting Up the Project
Let's start by setting up a new Spring Boot project with Spring Integration. You can use Spring Initializr to quickly generate a new project with the necessary dependencies. Make sure to include the Spring Integration
dependency when generating the project.
Defining Message Endpoints
In Spring Integration, message endpoints are the components that act as the starting point or the endpoint for messages. These endpoints can be inbound (to receive messages) or outbound (to send messages). We will define an inbound message endpoint to receive real-time data from a source.
@Component
public class DataReceiver {
@ServiceActivator(inputChannel = "inputChannel")
public void processData(String data) {
// Process the incoming data in real-time
// ...
}
}
In this example, the DataReceiver
class is annotated with @Component
to make it a Spring-managed bean. The processData
method is annotated with @ServiceActivator
, indicating that it is an inbound message endpoint that will receive messages from the inputChannel
. Upon receiving a message, the processData
method will be invoked to process the data in real-time.
Configuring Message Channels
Message channels act as the pathways for messages to flow within a Spring Integration application. We need to configure a message channel to connect the message source (e.g., a message queue, a WebSocket, etc.) to the message endpoint we defined earlier.
@Configuration
@EnableIntegration
public class IntegrationConfig {
@Bean
public MessageChannel inputChannel() {
return new DirectChannel();
}
}
In this configuration class, we define a bean of type DirectChannel
and name it inputChannel
. This channel will serve as the input channel for receiving real-time data. We annotate the configuration class with @EnableIntegration
to enable Spring Integration within the application.
Sending Real-Time Data
To complete the picture, let's consider an example of how real-time data can be sent to the input channel. For instance, in a web application, the incoming data can be obtained from an HTTP request and then pushed to the input channel for further processing.
@RestController
public class DataController {
@Autowired
private MessageChannel inputChannel;
@PostMapping("/data")
public void sendData(@RequestBody String data) {
inputChannel.send(MessageBuilder.withPayload(data).build());
}
}
In this example, the DataController
class contains a method to handle incoming HTTP POST requests. The sendData
method obtains the incoming data from the request body and then sends it to the inputChannel
using a MessageChannel
bean autowired into the controller. The MessageBuilder
class is used to create a message with the payload set to the incoming data.
Real-Time Data Processing Scenarios
Real-time data processing is essential in various scenarios, including:
- IoT Data Processing: Handling real-time data from sensors and devices in an IoT (Internet of Things) environment.
- Financial Data Processing: Processing real-time financial market data for trading and analysis.
- Log Monitoring: Analyzing and reacting to real-time log data for system monitoring and troubleshooting.
Messaging Patterns in Real-Time Data Processing
Spring Integration supports various messaging patterns that are vital in real-time data processing scenarios:
- Publish-Subscribe (Pub/Sub) Pattern: Distributing real-time data to multiple subscribers for parallel processing.
- Point-to-Point (P2P) Pattern: Sending real-time data to a specific recipient for exclusive processing.
- Message Routing: Routing real-time data to different processing components based on certain criteria.
Handling Real-Time Data Streams
In real-time data processing, it's common to deal with continuous streams of data rather than individual messages. Spring Integration provides support for handling data streams through its integration with reactive programming libraries such as Project Reactor.
@Component
public class DataStreamProcessor {
@Transformer(inputChannel = "streamInputChannel", outputChannel = "processedDataChannel")
public Flux<String> processStream(Flux<String> dataStream) {
// Perform real-time processing on the data stream using reactive operators
return dataStream.map(data -> data.toUpperCase());
}
}
In this example, the DataStreamProcessor
class is annotated with @Component
and contains a method to process a stream of real-time data. The @Transformer
annotation indicates that this method will transform the incoming data stream and produce an output stream. We use Project Reactor's Flux
to represent the input and output data streams, allowing for easy manipulation of the real-time data using reactive operators.
The Bottom Line
Real-time data processing is a fundamental requirement in many modern applications, and Spring Integration provides a powerful platform for building real-time data processing pipelines. By leveraging message endpoints, channels, and the support for reactive programming, developers can create robust, scalable, and reactive real-time data processing systems.
In this blog post, we've explored the basic concepts of real-time data processing with Spring Integration and demonstrated how to set up message endpoints, channels, and stream processing components to handle real-time data. By understanding these fundamental concepts and patterns, developers can build sophisticated real-time data processing solutions to meet the demands of modern, data-driven applications.
Start building your real-time data processing application with Spring Integration and unleash the power of real-time data processing in your projects!
References: