Cracking the Code: Top Spring Boot Interview Pitfalls
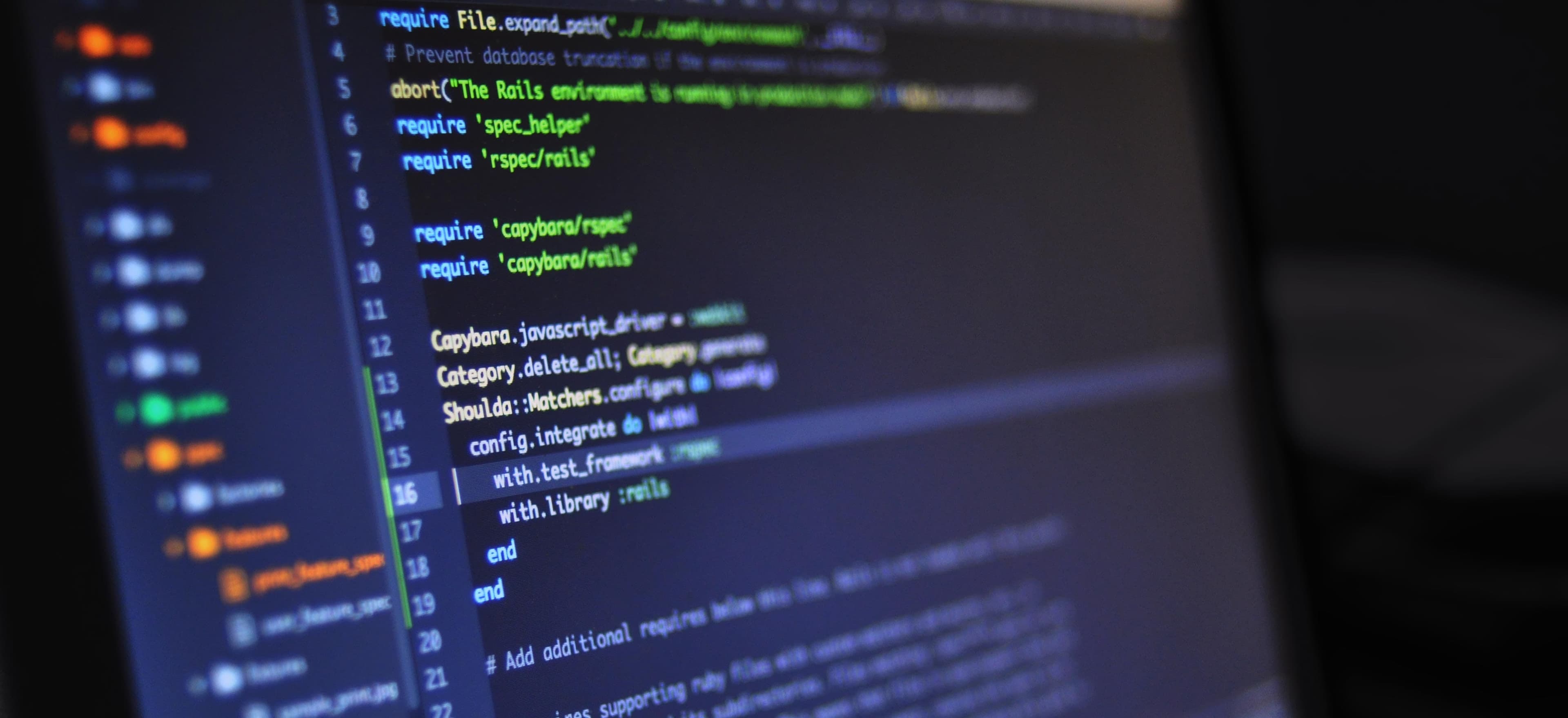
- Published on
Cracking the Code: Top Spring Boot Interview Pitfalls
Spring Boot has emerged as a popular framework for building enterprise-level applications with Java. Its simplicity and productivity benefits make it a go-to choice for developers. However, when transitioning into a Spring Boot developer role, interviews can be challenging. In this post, we will explore common pitfalls in Spring Boot interviews and provide actionable insights to help you navigate them effectively. We’ll also include code snippets to illustrate important concepts and best practices.
Understanding Spring Boot
Spring Boot is a convention-over-configuration solution that allows developers to create stand-alone, production-grade Spring applications quickly. It simplifies the setup and development of new Spring applications, handling much of the configuration work behind the scenes.
Key Features of Spring Boot:
- Auto-configuration: Automatically configures your application based on the dependencies present.
- Embedded servers: Allows you to run applications as standalone services without external web servers.
- Production-ready: Built-in metrics and monitoring for production environments.
The Importance of Understanding Fundamentals
In many interviews, candidates stumble due to a lack of understanding of the foundational principles of Spring and Spring Boot. It's crucial to articulate how those principles translate into actionable coding practices.
Pitfall #1: Skipping the Basics
Example Question: What is Spring Dependency Injection, and how does it apply to Spring Boot?
Avoid this response: "I don't know."
Better Answer: "Spring Dependency Injection (DI) is a design pattern used to manage dependencies in an application. In Spring Boot, DI allows us to define a class's dependencies outside of the class itself. Here is an example of how DI works in Spring Boot."
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id).orElseThrow(() -> new UserNotFoundException(id));
}
}
This code snippet demonstrates constructor injection, a key feature of Spring's DI. The @Autowired
annotation specifies that UserRepository
should be injected, promoting loose coupling and enhancing testability.
Common Spring Boot Interview Scenarios
Understanding common scenarios you may face can improve your confidence and performance in interviews.
Pitfall #2: Ignoring Configuration Options
Many developers underestimate the role of application properties or YAML configuration files in Spring Boot. Be prepared to discuss how configurations influence application behavior.
Example Question: How do you manage different environments (development, testing, production) in Spring Boot?
Answer: "Spring Boot allows us to manage different configurations using profile-specific properties files. For example, we can create an application-dev.properties
for development settings and an application-prod.properties
for production. We activate these profiles using the spring.profiles.active
property."
# application-dev.properties
spring.datasource.url=jdbc:mysql://localhost:3306/devdb
spring.datasource.username=dev
spring.datasource.password=dev123
# application-prod.properties
spring.datasource.url=jdbc:mysql://prodserver:3306/proddb
spring.datasource.username=prod
spring.datasource.password=prod123
Utilizing profiles ensures that your application runs in the correct configuration context, avoiding potential pitfalls related to incorrect settings.
Pitfall #3: Not Grasping the Role of Spring Boot Starters
Spring Boot Starters are essential for streamlining the dependency aspect of Spring applications. Failing to know how they work can be a red flag in an interview.
Example Question: What are Spring Boot Starters, and why should you use them?
Answer: "Spring Boot Starters are a set of convenient dependency descriptors you can include in your application to simplify dependency management. For instance, if you're building a RESTful API, you would use the spring-boot-starter-web
, which bundles all necessary libraries for web development."
Pitfall #4: Overlooking Error Handling
Proper error handling is crucial for building robust applications. Interviews often touch on this aspect to gauge your understanding of application reliability.
Example Question: How do you handle exceptions in a Spring Boot application?
Answer: "In Spring Boot, we can make use of @ControllerAdvice
to manage exceptions globally. This allows us to centralize our error handling strategy and avoid duplicating code."
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public String handleUserNotFoundException(UserNotFoundException ex) {
return ex.getMessage();
}
}
In this code, we intercept UserNotFoundException
and return an appropriate HTTP status code without cluttering our controllers with error handling logic.
Pitfall #5: Needing Database Integration Knowledge
Interviews for Spring Boot positions often focus on integrating the application with databases—something every developer should be prepared to discuss.
Example Question: How do you configure a database connection in Spring Boot?
Answer: "Spring Boot simplifies database connection configuration through properties files. With spring.datasource
properties, I can easily connect to various databases like MySQL, PostgreSQL, etc."
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
spring.jpa.hibernate.ddl-auto=update
The spring.jpa.hibernate.ddl-auto
property is crucial—it manages the database schema. Setting it to update
allows Hibernate to update the schema without dropping existing data.
Preparing for Advanced Questions
As you progress in your interview process, be ready to encounter more advanced questions that might not just concern Spring Boot but also the broader Java ecosystem.
Pitfall #6: Missing Out on Microservices Concepts
Since many Spring Boot applications involve microservices, reviewing microservices architecture is vital.
Example Question: What are some approaches to achieve inter-service communication in a microservices architecture?
Answer: "Inter-service communication can be achieved through:
- REST APIs, allowing services to communicate over HTTP.
- Message brokers like RabbitMQ or Kafka for asynchronous communication."
You can delve deeper into these concepts by referring to the Spring Cloud documentation for practical examples and best practices.
Pitfall #7: Failing to Showcase Real-World Experience
Interviews are as much about demonstrating your experience as they are about technical knowledge. Be prepared to share project experiences where you successfully implemented Spring Boot solutions.
Example Discussion: "In my last project, I developed a microservices-based e-commerce application using Spring Boot. I utilized Spring Security for authentication and PostgreSQL for database management. This experience honed my skills in building scalable applications."
Bringing It All Together
Navigating a Spring Boot interview can be daunting, but being aware of common pitfalls can significantly enhance your chances of success. Focus on understanding key concepts, prepare for application scenarios, and be ready to showcase your real-world experience. Armed with these insights, you're now one step closer to cracking the code in your Spring Boot interviews.
Good luck!
Additional Resources
- Spring Boot Documentation
- Understanding Dependency Injection in Spring
By preparing effectively and familiarizing yourself with the common pitfalls, you will elevate your interview performance and boost your confidence, making you a desirable candidate in the job market.
Checkout our other articles