Preventing SQL Injection in Java Applications
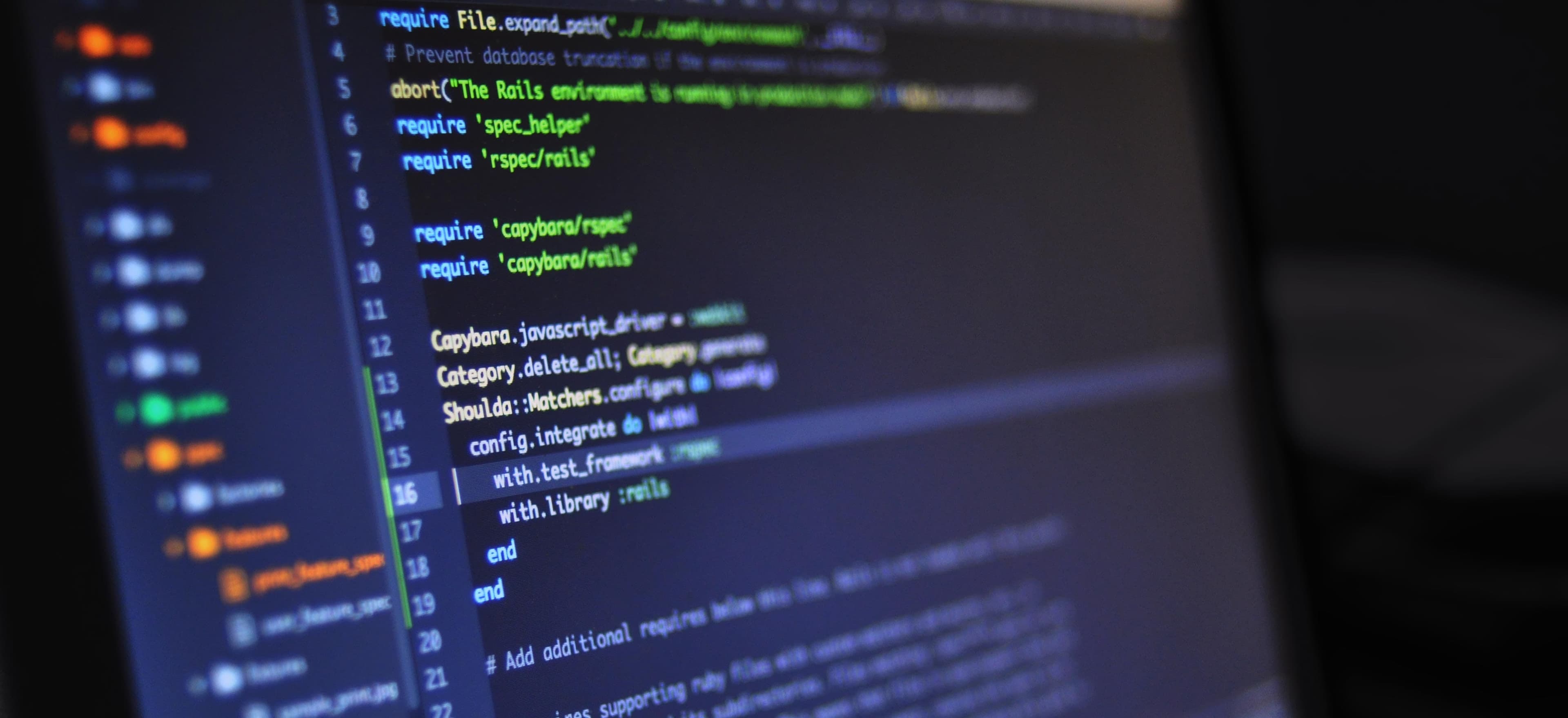
- Published on
Preventing SQL Injection in Java Applications
SQL injection is a common security vulnerability in web applications that can allow attackers to interfere with the queries that an application makes to its database. This can lead to unauthorized access, data manipulation, or even data loss. In this blog post, we will discuss how to prevent SQL injection in Java applications.
What is SQL Injection?
SQL injection is a technique where an attacker inserts malicious SQL code into a query, manipulating the original purpose of the query. This can happen when user input is directly concatenated into SQL statements without proper validation or sanitization. For example, consider the following Java code:
String username = request.getParameter("username");
String password = request.getParameter("password");
String query = "SELECT * FROM users WHERE username='" + username + "' AND password='" + password + "'";
If an attacker provides username
as admin';--
and password
as anything, the resulting SQL query would become:
SELECT * FROM users WHERE username='admin';--' AND password='anything'
The --
in SQL signifies a comment, effectively nullifying the rest of the original query. This would allow the attacker to bypass the login mechanism.
Prepared Statements
One of the most effective ways to prevent SQL injection in Java applications is to use prepared statements. Instead of concatenating user input directly into the SQL query, prepared statements use placeholders and parameterized queries. Here's how the previous example can be rewritten using prepared statements:
String username = request.getParameter("username");
String password = request.getParameter("password");
String query = "SELECT * FROM users WHERE username=? AND password=?";
PreparedStatement pstmt = connection.prepareStatement(query);
pstmt.setString(1, username);
pstmt.setString(2, password);
ResultSet rs = pstmt.executeQuery();
In this example, ?
is used as a placeholder for the parameters. The setString
method then sets the actual values for the placeholders. Using prepared statements automatically escapes the input, preventing SQL injection attacks.
Input Validation and Sanitization
While prepared statements provide a strong defense against SQL injection, input validation and sanitization should also be performed as an additional layer of security. This ensures that the input meets certain criteria, such as length, format, or character encoding.
For example, when expecting a numerical input, you can use:
int id = 0;
try {
id = Integer.parseInt(request.getParameter("id"));
} catch (NumberFormatException e) {
// Handle the error
}
Furthermore, you can use libraries like OWASP's ESAPI to sanitize input and perform validation against a set of predefined rules. For example, to sanitize input for a SQL query, you can use:
String username = ESAPI.encoder().encodeForSQL(new OracleCodec(), request.getParameter("username"));
Least Privilege Principle
Another important practice in preventing SQL injection is to follow the principle of least privilege. This means that the database user account used by the application should have the minimal set of permissions required to perform its tasks. It's a good practice to create separate user accounts with different access levels for different parts of the application, and only grant the necessary permissions to each account.
By following the least privilege principle, even if an SQL injection attack is successful, the potential damage is limited to the permissions of the targeted database user.
Hibernate and JPA
If you're using an ORM (Object-Relational Mapping) framework like Hibernate or JPA (Java Persistence API), you're already benefiting from a certain level of protection against SQL injection. These frameworks often handle the translation of Java objects to database queries, effectively mitigating the risk of direct SQL injection.
Here's an example of using Hibernate to perform a parameterized query:
String username = request.getParameter("username");
String password = request.getParameter("password");
String query = "FROM User u WHERE u.username = :username AND u.password = :password";
Query<User> q = session.createQuery(query, User.class);
q.setParameter("username", username);
q.setParameter("password", password);
List<User> users = q.getResultList();
In this case, Hibernate takes care of parameter binding and sanitization behind the scenes.
Web Application Firewalls
In addition to secure coding practices, utilizing a Web Application Firewall (WAF) can provide an extra layer of defense against SQL injection and other common web application vulnerabilities. A WAF can inspect and filter HTTP requests to block malicious traffic before it reaches the application.
Popular WAF solutions include ModSecurity and Cloudflare WAF. These can be configured to detect and block SQL injection attempts based on predefined rules or custom rule sets.
Regular Security Training and Code Reviews
It's crucial to ensure that everyone involved in the development process, from developers to quality assurance engineers, is aware of the risks associated with SQL injection and is trained to write secure code. Conducting regular security training and code reviews can significantly reduce the likelihood of introducing vulnerabilities into the codebase.
Code reviews provide an opportunity to catch potential SQL injection vulnerabilities before they make their way into production. For example, by ensuring that all database interactions are handled using prepared statements or ORM frameworks.
To Wrap Things Up
Preventing SQL injection in Java applications requires a combination of secure coding practices, the use of prepared statements, input validation, least privilege principle, and possibly the incorporation of additional security measures such as WAFs. By following these practices, you can significantly reduce the risk of SQL injection vulnerabilities and protect your application and its data from potential attacks.
In summary:
- Always use prepared statements or parameterized queries to interact with databases.
- Validate and sanitize user input to ensure that it meets expected criteria and is free from malicious content.
- Follow the principle of least privilege when granting database permissions.
- Consider leveraging ORM frameworks like Hibernate and incorporate additional security measures such as WAFs.
- Regularly train and educate developers on secure coding practices and conduct thorough code reviews.
By incorporating these strategies into your Java applications, you can bolster their security and minimize the risk of SQL injection vulnerabilities.