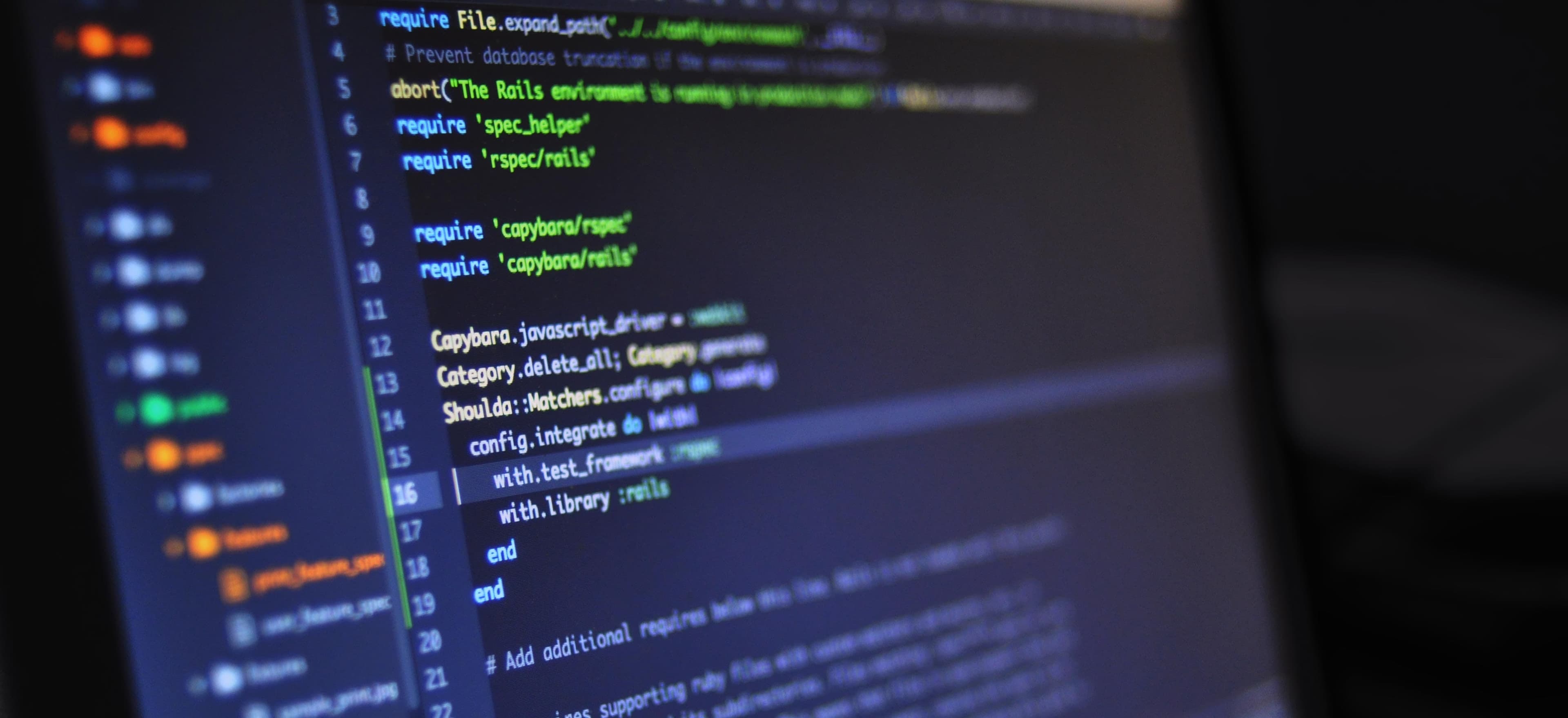
- Published on
In Android development, data binding plays a pivotal role in connecting the app's UI components with the app's data sources. This approach simplifies the code and allows for a more structured and efficient app design. In this blog post, we will explore the process of data binding in Android XML views, discussing its advantages and providing practical examples.
What is Data Binding?
In essence, data binding establishes a direct connection between the UI components of an Android app and the data sources, effectively eliminating the need for manual interaction between the view elements and the underlying data. This approach significantly reduces boilerplate code and makes the codebase more maintainable.
Advantages of Data Binding:
-
Reduced Boilerplate Code: Data binding eliminates the need for
findViewById
calls, resulting in cleaner and more concise code. -
Ease of Integration: It simplifies the process of connecting views with data sources, making UI updates more efficient.
-
Improved Code Readability: By keeping the data-related logic separate from the UI, data binding enhances code maintainability and readability.
Now, let's delve into the practical implementation of data binding in Android XML views.
Setting Up Data Binding in Android:
To enable data binding in an Android project, follow these steps:
-
Update build.gradle: Add the following lines to the
build.gradle
file in the app module to enable data binding:android { ... buildFeatures { dataBinding true } }
-
Enable Data Binding in Layout Files: In the root layout of the XML file, add the
layout
tag with thedata
tag as a child, specifying the variables that will be used for data binding.<layout ...> <data> <variable name="user" type="com.example.User" /> </data> <!-- Your UI layout here --> </layout>
With data binding set up, the next step is to bind the data to the XML views.
Binding Data to XML Views:
Binding Data Using <data>
Tag:
Inside the XML layout file, you can reference the variables specified in the data
tag to bind data to the UI components. For example, let's consider binding a user's name to a TextView:
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@{user.name}" />
In this example, @{user.name}
binds the name
property of the user
object to the TextView
.
Binding Click Handlers:
Data binding also facilitates binding click handlers directly in the XML layout. This eliminates the need for finding the view and setting the click listener in the code.
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"
android:onClick="@{() -> viewModel.onButtonClick()}" />
In this case, the onClick
attribute is bound to the onButtonClick
method of the viewModel
.
Using Binding Adapters:
Binding adapters allow custom behavior to be added to the bindings, enabling complex logic to be included in the XML layout. For example, you can create a binding adapter to load an image from a URL into an ImageView:
@BindingAdapter("imageUrl")
public static void loadImage(ImageView view, String url) {
// Code to load the image from the URL using libraries like Glide or Picasso
}
This binding adapter can then be used in the XML layout to load the image:
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:imageUrl="@{user.imageUrl}" />
Two-Way Data Binding:
Two-way data binding allows data to flow both from the source to the UI and from the UI back to the source. This is particularly useful for input fields where changes need to be reflected in the underlying data model.
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@={user.name}" />
In this snippet, @={user.name}
enables two-way binding, updating the name
property of the user
object when the text in the EditText is altered.
The Last Word:
Implementing data binding in Android XML views offers a more efficient and structured way of connecting UI components to data sources. By reducing boilerplate code and enhancing code readability, data binding simplifies the development process and contributes to a more maintainable codebase.
Data binding is a powerful tool that not only streamlines the UI development process but also improves the overall architecture of Android applications. Its seamless integration with XML views and support for advanced features, such as two-way data binding, make it an invaluable addition to the Android development toolkit.
In conclusion, data binding in Android XML views is a fundamental aspect of modern Android development, empowering developers to create robust, maintainable, and efficient applications with a clean separation between UI and data logic.
If you're interested in learning more about Android data binding, check out the official Android Data Binding Guide. Happy coding!