Managing Method Complexity in Java
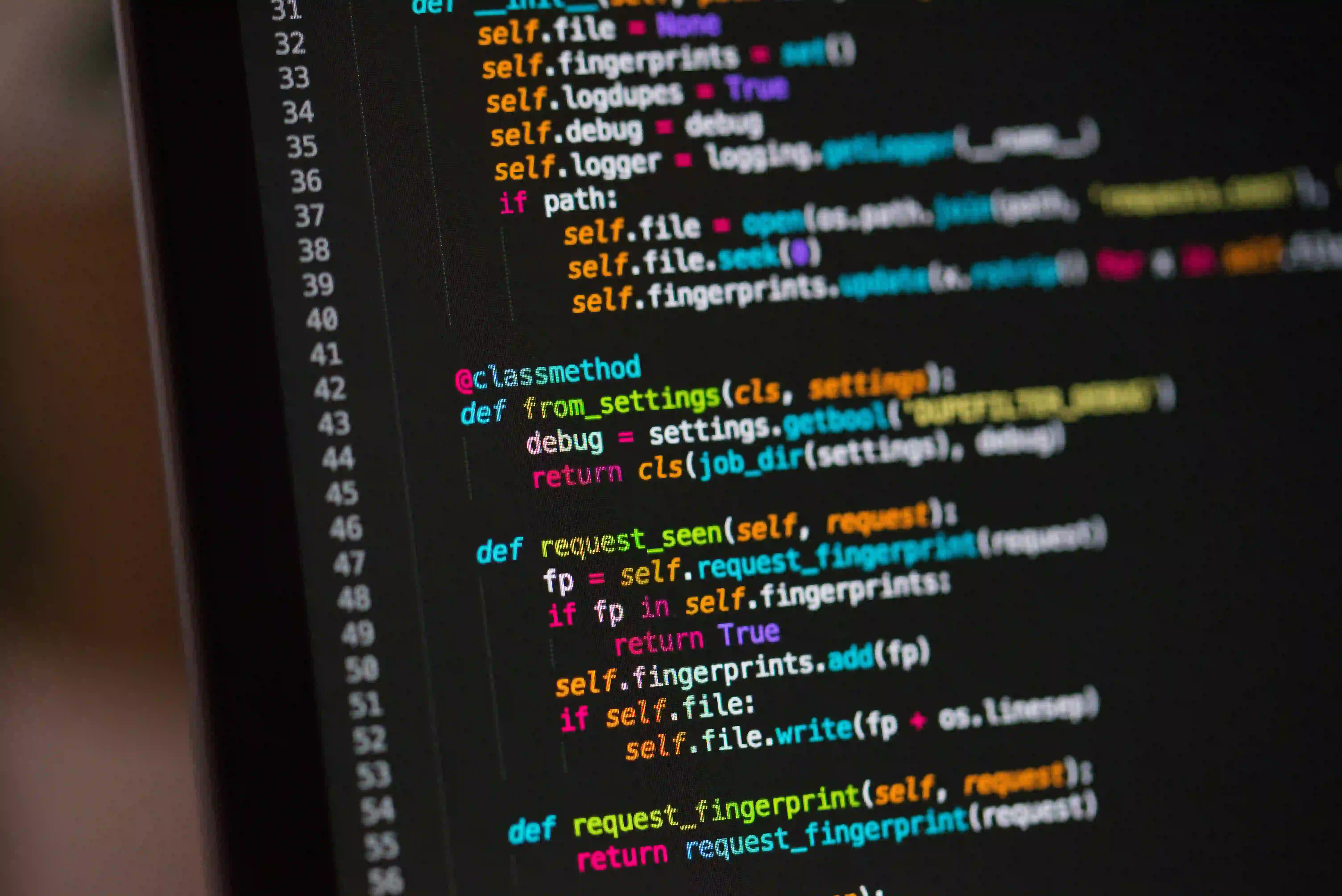
Managing Method Complexity in Java
In software development, maintaining clean, readable, and efficient code is crucial for the long-term success of a project. One important aspect of clean code is managing the complexity of methods. Methods with high complexity can be difficult to understand, debug, and maintain. In this article, we will discuss various techniques and best practices for managing method complexity in Java.
Understanding Method Complexity
Method complexity can be measured in various ways, but one common metric is the cyclomatic complexity. Cyclomatic complexity is a software metric used to measure the complexity of a program. In Java, cyclomatic complexity is calculated based on the number of linearly independent paths through a method's control flow.
The Single Responsibility Principle
The Single Responsibility Principle (SRP) is a fundamental principle of software development that states that a class or method should have only one reason to change. When a method is responsible for multiple tasks, it tends to have higher complexity.
Let's consider an example:
public class PaymentProcessor {
public void processPayment(Order order) {
validateOrder(order);
calculatePaymentAmount(order);
applyDiscount(order);
sendPaymentConfirmation(order);
}
}
In the above example, the processPayment
method is responsible for multiple tasks such as validation, calculation, applying discount, and sending confirmation. This violates the SRP, leading to higher complexity. A better approach would be to have separate methods for each task, following the SRP.
Extracting Smaller Methods
One effective way to reduce method complexity is by extracting smaller methods from a large, complex method. This not only improves readability but also facilitates reusability and testability.
Consider the following example:
public class DataProcessor {
public void processData(List<Data> dataList) {
// Complex data processing logic
// ...
}
}
The processData
method contains complex logic for processing data. We can reduce the complexity by extracting smaller methods for specific tasks such as data validation, transformation, and aggregation.
public class DataProcessor {
public void processData(List<Data> dataList) {
validateData(dataList);
transformData(dataList);
aggregateData(dataList);
}
private void validateData(List<Data> dataList) {
// Data validation logic
// ...
}
private void transformData(List<Data> dataList) {
// Data transformation logic
// ...
}
private void aggregateData(List<Data> dataList) {
// Data aggregation logic
// ...
}
}
By extracting smaller methods, we have effectively reduced the complexity of the processData
method.
Utilizing Helper Methods and Classes
Another approach to manage method complexity is to utilize helper methods and classes. Helper methods encapsulate specific tasks and provide a modular way to break down complex logic.
For instance:
public class FileProcessor {
public void processFile(String filePath) {
// Complex file processing logic
// ...
}
}
We can reduce the complexity by utilizing helper methods for file validation, parsing, and handling.
public class FileProcessor {
public void processFile(String filePath) {
if (isValidFile(filePath)) {
String fileContent = parseFile(filePath);
handleFileContent(fileContent);
}
}
private boolean isValidFile(String filePath) {
// File validation logic
// ...
}
private String parseFile(String filePath) {
// File parsing logic
// ...
}
private void handleFileContent(String fileContent) {
// File content handling logic
// ...
}
}
Similarly, for more complex scenarios, it might be beneficial to create separate helper classes to encapsulate related functionality and reduce method complexity.
Utilizing Data Structures and Design Patterns
Applying appropriate data structures and design patterns can also help manage method complexity. For example, using data structures such as maps, sets, and lists can simplify complex data manipulation within a method. Additionally, design patterns such as the Strategy pattern, Factory pattern, and Decorator pattern can help in decoupling and simplifying complex logic.
Consider the following example using the Strategy pattern:
public class PaymentProcessor {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void processPayment(Order order) {
// Complex payment processing logic using the selected strategy
if (paymentStrategy != null) {
paymentStrategy.processPayment(order);
}
}
}
By using the Strategy pattern, the complexity of the payment processing logic is encapsulated within separate strategy implementations, making the processPayment
method more focused and less complex.
Writing Self-Documenting Code
Writing self-documenting code can greatly contribute to managing method complexity. By choosing descriptive and meaningful method and variable names, using consistent formatting, and providing clear comments when necessary, the code becomes easier to understand, reducing the cognitive load for developers.
For example:
public void calculateTotalPrice(Order order) {
double basePrice = calculateBasePrice(order);
double discount = calculateDiscount(order);
double tax = calculateTax(order);
double totalPrice = basePrice - discount + tax;
order.setTotalPrice(totalPrice);
}
In the above example, the method calculateTotalPrice
is self-documenting, making it clear that it calculates the total price for an order based on various components.
Bringing It All Together
Managing method complexity is essential for writing maintainable, scalable, and understandable code. By adhering to principles such as the Single Responsibility Principle, extracting smaller methods, utilizing helper methods and classes, leveraging appropriate data structures and design patterns, and writing self-documenting code, developers can effectively reduce method complexity in Java. These practices not only enhance readability and maintainability but also contribute to the overall quality of software systems.
In conclusion, method complexity management is not just a good practice—it's a necessity for building robust and sustainable software solutions in Java.
By implementing these techniques and best practices, developers can significantly improve the quality and maintainability of their Java codebase.
Remember, cleaner code leads to happier developers and better software!
Start managing method complexity today for a brighter and more maintainable codebase.
Happy coding!
Clean Code: A Handbook of Agile Software Craftsmanship Design Patterns: Elements of Reusable Object-Oriented Software