Writing a blog article title: "Mastering Grails HQL Queries: executeQuery and executeUpdate"
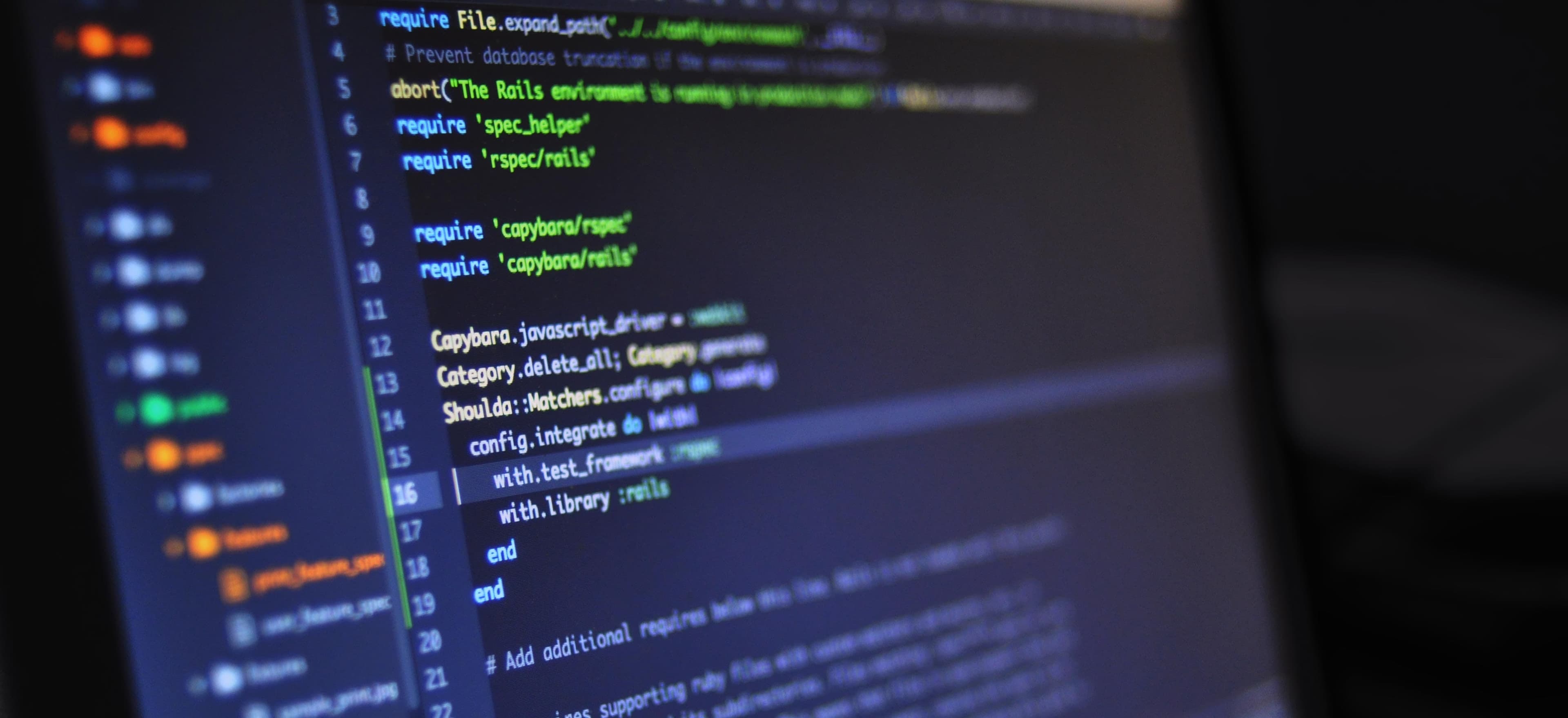
- Published on
Mastering Grails HQL Queries: executeQuery and executeUpdate
When working with Grails applications, the GORM (Grails Object Relational Mapping) provides a powerful and flexible way to interact with the database. HQL (Hibernate Query Language) is a key component in GORM that allows developers to write database queries in an object-oriented manner.
In this article, we will dive deep into two essential methods for executing HQL queries in Grails: executeQuery
and executeUpdate
. Understanding these methods and how to utilize them effectively can greatly enhance your ability to work with databases in Grails.
Let's begin by exploring the executeQuery
method, which is primarily used for retrieving data from the database.
The executeQuery Method
The executeQuery
method allows you to execute arbitrary HQL or SQL against the database and retrieve the results as objects or collections of objects. This method is particularly useful when you need to perform complex queries that cannot be easily expressed using the methods provided by GORM.
Syntax
The syntax for using executeQuery
is as follows:
def results = YourDomainClass.executeQuery("from YourDomainClass where someProperty = :value", [value: propValue])
In this syntax:
YourDomainClass
refers to the domain class against which the query is being executed."from YourDomainClass where someProperty = :value"
represents the HQL query to be executed. Here,someProperty
is a placeholder for the actual property, and:value
is a named parameter that can be passed in as part of the query execution.[value: propValue]
is a map that provides values for the named parameters in the query.
Example
Suppose we have a Book
domain class, and we want to retrieve all books published after a certain year:
def year = 2010
def recentBooks = Book.executeQuery("from Book where publishYear > :year", [year: year])
In this example, recentBooks
will contain a collection of Book
objects that satisfy the query criteria.
Use Case: Custom Aggregations
One common use case for executeQuery
is when you need to perform custom aggregations or calculations that are not directly supported by GORM methods. For instance, if you need to calculate the average price of books in a certain category, you can use executeQuery
to achieve this.
The executeUpdate Method
While executeQuery
is intended for retrieving data, the executeUpdate
method is used for executing HQL or SQL statements that modify data in the database, such as INSERT, UPDATE, or DELETE operations.
Syntax
The syntax for using executeUpdate
is similar to executeQuery
:
def rowsAffected = YourDomainClass.executeUpdate("update YourDomainClass set someProperty = :newValue where anotherProperty = :value", [newValue: newValue, value: propValue])
In this syntax:
update YourDomainClass set someProperty = :newValue where anotherProperty = :value
represents the HQL update query to be executed.[newValue: newValue, value: propValue]
is a map containing values for named parameters in the query.
Example
Suppose we want to update the price of all books published by a specific author:
def author = "J.K. Rowling"
def newPrice = 15.99
def rowsAffected = Book.executeUpdate("update Book set price = :newPrice where author = :author", [newPrice: newPrice, author: author])
In this example, rowsAffected
will contain the number of book records that were updated.
Use Case: Batch Updates and Deletes
The executeUpdate
method is particularly useful when you need to perform batch updates or deletes based on certain criteria. Instead of retrieving individual records and updating them one by one, you can use executeUpdate
to perform the operation efficiently in a single database call.
Best Practices and Considerations
When using executeQuery
and executeUpdate
, it's important to keep the following best practices and considerations in mind:
Parameterized Queries
Always use parameterized queries to prevent SQL injection attacks and to ensure the security and integrity of your database operations.
Transaction Management
Both executeQuery
and executeUpdate
execute database operations outside of the standard GORM mechanisms. Therefore, you need to be mindful of transaction management, especially when performing updates or deletes that impact the database state.
Performance Implications
While executeQuery
and executeUpdate
provide flexibility, they can potentially have performance implications, especially when executing complex or frequent queries. Always benchmark and optimize your queries for better performance.
A Final Look
In this article, we have explored the executeQuery
and executeUpdate
methods in Grails HQL queries. These methods serve as powerful tools for executing custom and complex database operations, filling in the gaps left by the standard GORM methods.
By mastering these methods and understanding their best practices, you can unlock the full potential of HQL queries in Grails and elevate your database interaction to a new level of flexibility and efficiency.
Now that you have a grasp of these essential methods, it's time to incorporate them into your Grails projects and witness the impact they can have on your database operations.
For further reading on HQL and GORM, check out the Grails documentation and the Hibernate official site for a deeper understanding of the underlying concepts and practices.
Happy querying!