Top 5 Open Source Java Libraries for Efficient Development
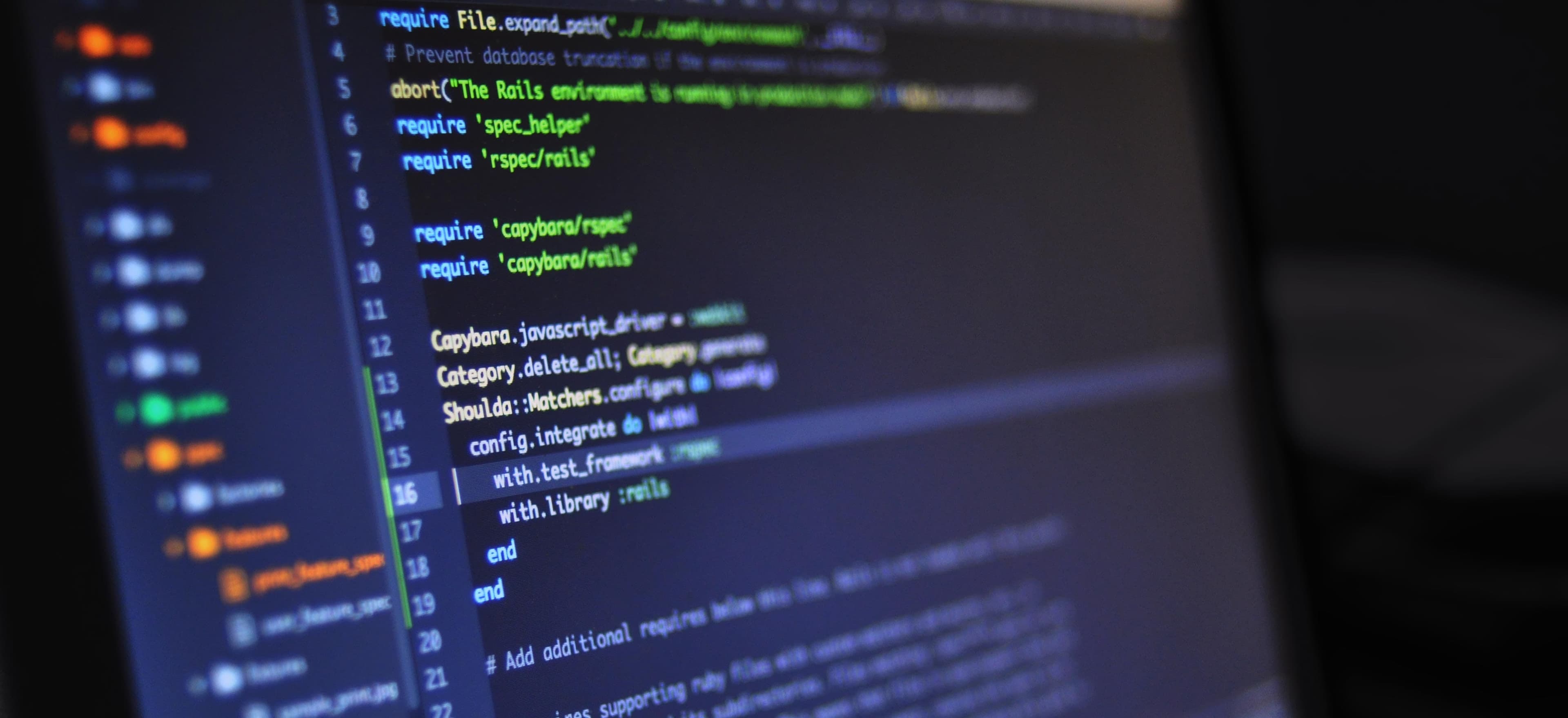
- Published on
Are you a Java developer looking to enhance your development process and deliver high-quality software more efficiently? Look no further! In this article, we'll explore five open-source Java libraries that are essential for any Java developer's toolkit. These libraries are designed to streamline development workflows, improve code quality, and boost productivity. Let's dive into the world of Java libraries and discover how they can revolutionize your development experience.
1. Lombok
Project Lombok is a phenomenal library that eliminates Java boilerplate code and empowers developers to write more concise and readable code. By using annotations such as @Getter
, @Setter
, @Builder
, and @Data
, Lombok automatically generates the corresponding methods at compile time, reducing the need for manual, repetitive coding. This results in cleaner, more maintainable code without sacrificing readability.
Here's an example of how Lombok can simplify your code:
// Without Lombok
public class User {
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
// With Lombok
import lombok.Data;
@Data
public class User {
private String firstName;
private String lastName;
}
By using Lombok, you eliminate boilerplate code, improve code readability, and reduce the likelihood of errors. Isn't that fantastic?
2. Guava
Guava, developed by Google, provides a wide range of utilities to simplify common programming tasks in Java. This powerful library offers functionalities for collections, caching, primitives support, concurrency, and more. One standout feature of Guava is its ImmutableCollections
, which provides immutable implementations of standard Java collection interfaces. Immutability ensures that the state of a collection cannot be altered after construction, preventing unexpected side effects and simplifying concurrent programming.
Let's take a look at an example of Guava's ImmutableCollections:
// Creating an immutable list using Guava
import com.google.common.collect.ImmutableList;
public class Main {
public static void main(String[] args) {
ImmutableList<String> immutableList = ImmutableList.of("apple", "banana", "cherry");
// immutableList.add("date"); // This will throw an UnsupportedOperationException
}
}
By utilizing Guava's ImmutableCollections, you can enhance the stability and predictability of your code, especially in multi-threaded environments.
3. Apache Commons Lang
Apache Commons Lang is a library that provides a host of helper utilities for the Java programming language. This library covers a wide range of topics, including String manipulation, exception handling, reflection, and more. One of the most widely used classes in Apache Commons Lang is StringUtils
, which offers powerful tools for working with strings.
Let's see how Apache Commons Lang can simplify string manipulation:
// Using Apache Commons Lang for string manipulation
import org.apache.commons.lang3.StringUtils;
public class Main {
public static void main(String[] args) {
String input = " Trim this sentence ";
String trimmed = StringUtils.trim(input);
System.out.println(trimmed); // Output: "Trim this sentence"
}
}
By leveraging Apache Commons Lang, you can enhance your string handling capabilities and reduce the amount of custom code you need to write.
4. JUnit
Unit testing is a crucial aspect of modern software development, and JUnit is the go-to framework for writing and running repeatable tests. With its annotation-driven approach, JUnit makes it easy to define and execute tests, assert expected outcomes, and handle test fixtures. Writing tests in JUnit not only ensures the reliability of your codebase but also promotes a test-driven development (TDD) approach, leading to higher code quality and maintainability.
Here's an example of a simple JUnit test:
// A simple JUnit test case
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 4);
assertEquals(7, result);
}
}
By incorporating JUnit into your development workflow, you can establish a robust testing infrastructure, catch bugs early, and instill confidence in your codebase.
5. Jackson
JSON (JavaScript Object Notation) has become the de-facto standard for data interchange in modern web applications. Jackson, a high-performance JSON processor, provides a seamless mechanism for parsing JSON data into Java objects and vice versa. With its annotation-based configuration and powerful data binding capabilities, Jackson simplifies the handling of JSON data, making it an indispensable library for working with web services and APIs.
Let's illustrate how Jackson facilitates JSON processing:
// Using Jackson for JSON processing
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) throws IOException {
ObjectMapper objectMapper = new ObjectMapper();
String jsonString = "{\"name\":\"John\",\"age\":30}";
Person person = objectMapper.readValue(jsonString, Person.class);
System.out.println(person.getName()); // Output: "John"
}
}
By leveraging Jackson, you can seamlessly integrate JSON data into your Java applications, paving the way for efficient communication with external systems.
Final Thoughts
In the world of Java development, having the right set of libraries at your disposal can make all the difference in streamlining your workflow, improving code quality, and boosting productivity. The open-source Java libraries mentioned in this article – Lombok, Guava, Apache Commons Lang, JUnit, and Jackson – offer a wealth of features and capabilities that cater to various aspects of modern software development. By incorporating these libraries into your projects, you can elevate your development experience and deliver exceptional software with confidence. Now, it's time to augment your Java arsenal with these incredible libraries and embark on a journey of efficient and robust development. Happy coding!
Checkout our other articles